Question
Part I: Subtracting three integers. Write a program that subtracts three integers, i.e., calculating (x y - z) for three integers x, y, z that
Part I: Subtracting three integers.
Write a program that subtracts three integers, i.e., calculating (x y - z) for three integers x, y, z that user entered. Use the register EAX to store the final result. You can assume that x, y, and z will all be given as unsigned values such that x >= y + z (or that x y z >= 0). Your program shall have the following three features:
1. Display the welcome message;
2. Allowing the user to input the three integers from keyboard;
3. Display the result in decimal in the console window.
|
For example, the runtime screen shot can be as follows (the three numbers entered for x, y and z can be either on the same line or across three lines):
Welcome! This program subtracts three integer numbers.
Please input three integers x, y, and z:
40
20
5
The result of (x y z) is: 15
Press any key to continue
Part II: Making your program repetitive, i.e., allowing the user to calculate subtraction of a new group of three integers after having done with one group. Your program needs to clear the screen before starting with a new group.
Part III: Execute your program line by line and trace the change of register EAX. Include screenshots in your lab report to show the change of EAX. (You need to set a breakpoint at the beginning of your program and run your program with the option Start Debugging).
[Hints:
1. To display a string, you should first define the string in data segment. Then insert the following two instructions to display it (in you program, you should replace stringName with the actual name of your string):
mov edx, OFFSET stringName
call WriteString
2. The instruction call ReadInt reads a 32-bit signed integer from the keyboard and returns the value in EAX.
3. The instruction call WriteDec writes a 32-bit unsigned integer in EAX to the console window in decimal format.
4. You need to store the integer in memory every time you read an integer from the keyboard. Otherwise, it will be overwritten by the newly read integer.
The following two instructions might be needed for Part II.
5. The instruction call Clrscr clears the console window.
6. Instruction jmp allows you to jump to a place indicated by L1 below:
L1:
jmp L1
Please refer to Chapter 5 Section 5.4 for details on how to use these instructions. ]
Requirements:
Submit your source code (.asm file) which should run correctly. (You just need to submit one .asm file since part 3 includes part 2 and part 2 includes part 1). Necessary comments are needed in your code.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
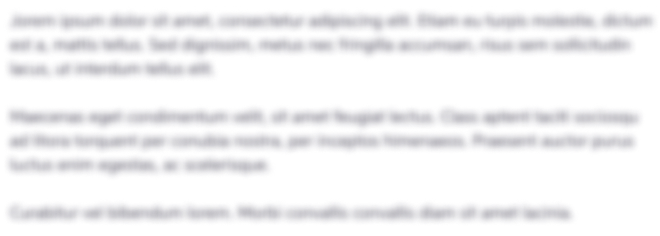
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started