Question
partial solution works fine too if it hard to solve the whole thing. File: cursor_based_list.py Description: Cursor-based list utilizing a header node and a trailer
partial solution works fine too if it hard to solve the whole thing.
File: cursor_based_list.py Description: Cursor-based list utilizing a header node and a trailer node. """
from node2way import Node2Way
class CursorBasedList(object): """ Linked implementation of a positional list.""" def __init__(self): """ Creates an empty cursor-based list.""" self._header = Node2Way(None) self._trailer = Node2Way(None) self._trailer.setPrevious(self._header) self._header.setNext(self._trailer) self._current = None self._size = 0
def hasNext(self): """ Returns True if the current item has a next item. Precondition: the list is not empty.""" if self.isEmpty(): raise AttributeError("Empty list has no next item") return self._current.getNext() != self._trailer
def hasPrevious(self): """ Returns True if the current item has a previous item. Precondition: the list is not empty.""" pass def first(self): """Moves the cursor to the first item if there is one. Precondition: the list is not empty.""" pass def last(self): """Moves the cursor to the last item if there is one. Precondition: the list is not empty.""" pass
def next(self): """Precondition: hasNext returns True. Postcondition: The current item is has moved to the right one item""" pass
def previous(self): """Precondition: hasPrevious returns True. Postcondition: The current item is has moved to the left one iten""" pass
def insertAfter(self, item): """Inserts item after the current item, or as the only item if the list is empty. The new item is the current item.""" pass
def insertBefore(self, item): """Inserts item before the current item, or as the only item if the list is empty. The new item is the current item.""" pass
def getCurrent(self): """ Returns the current item without removing it or changing the current position. Precondition: the list is not empty""" pass
def remove(self): """Removes and returns the current item. Making the next item the current item if one exists; otherwise the tail item in the list is the current item. Precondition: the list is not empty.""" pass
def replace(self, newItemValue): """Replaces the current item by the newItemValue. Precondition: the list is not empty.""" pass
def isEmpty(self): pass
def __len__(self): """ Returns the number of items in the list.""" # replace below return 0
def __str__(self): """Includes items from first through last.""" # replace below return ""
""" File: cursorBasedListTester.py
Menu-driven tester for Cursor-Based List implementation """
from cursor_based_list import CursorBasedList
def testList(): myList = CursorBasedList() while True: print(" ===============================================================") print("Current List:",myList) if myList.isEmpty(): print("Empty list") else: print("length:",len(myList), " Current item:", myList.getCurrent()) print(" Test Positional List Menu:") print("A - insertAfter") print("B - insertBefore") print("C - getCurrent") print("E - isEmpty") print("F - First") print("L - Last") print("N - Next") print("P - Previous") print("R - remove") print("U - replace") print("X - eXit testing") response = input("Menu Choice? ").upper() if response == 'A': item = input("Enter the new item to insertAfter: ") myList.insertAfter(item) elif response == 'B': item = input("Enter the new item to insertBefore: ") myList.insertBefore(item) elif response == 'C': item = myList.getCurrent() print("The current item in the list is:",item) elif response == 'E': print("isEmpty returned:", myList.isEmpty()) elif response == 'F': myList.first() elif response == 'L': myList.last() elif response == 'N': myList.next() elif response == 'P': myList.previous() elif response == 'R': item = myList.remove() print("item removed:",item) elif response == 'U': item = input("Enter replacement item: ") myList.replace(item) elif response == 'X': break else: print("Invalid Menu Choice!")
testList()
I will post the codes to work with at the end of the questions. Use PYTHON programing Part A: Recall that in a cursor-base list a cursor (indicating the current item can be moved around the list with the cursor being used to identify the region of the list to be manipulated. We will insert and removing items relative to the current item. A current item must always be defined as long as the list is not em Cursor-based operations .getCurrent ( ) L.hasNext () .next ( ) L.hasPrevious () L.previous Description of operation Precondition: the list is not empty. Returns the current item without removing it or changing the current position. Precondition: the list is not empty. Returns True if the current item has a next item; otherwise return False Precondition: hasNext returns True. Postcondition: The current item has moved right one item Precondition: the list is not empty. Returns True if the current item has a vious item; otherwise return False Precondition: hasPrevious returns True. Postcondition: The current item has moved left one item Precondition: the list is not empty. Makes the first item the current item Precondition: the list is not empty. Makes the last item the current item L.first .last ( ) L.insertAfter (item) Inserts item after the current item, or as the only item if the list is empty. The new item is the current item. L.insertBefore (item) Inserts item before the current item, or as the only item if the list is empty. The new item is the current item. L.replace (newValue L.remove ( Precondition: the list is not empty. Replaces the current item by the newValue Precondition: the list is not empty. Removes and returns the current item. Making the next item the current item if one exists; otherwise the tail item in the list is the current item unless the list in now emm The cur sor_based list.py file contains a skeleton CursorBasedList class. You MUST uses a doubly-linked list implementation with a header node and a trailer node. All "real" list items will be inserted between the header and trailer nodes to reduce the number of "special cases" (e.g., inserting first item in an empty list, deleting the last item from the list, etc.). An empty list looks like header current trailer vious data next previous data next size0 Use the provided cur sorBasedListTester.py program to test your list. PART B: Once you have your Cur sorBasedList class finished, you are to write a simple text-editor program that utilizes your CursorBasedList class. I will post the codes to work with at the end of the questions. Use PYTHON programing Part A: Recall that in a cursor-base list a cursor (indicating the current item can be moved around the list with the cursor being used to identify the region of the list to be manipulated. We will insert and removing items relative to the current item. A current item must always be defined as long as the list is not em Cursor-based operations .getCurrent ( ) L.hasNext () .next ( ) L.hasPrevious () L.previous Description of operation Precondition: the list is not empty. Returns the current item without removing it or changing the current position. Precondition: the list is not empty. Returns True if the current item has a next item; otherwise return False Precondition: hasNext returns True. Postcondition: The current item has moved right one item Precondition: the list is not empty. Returns True if the current item has a vious item; otherwise return False Precondition: hasPrevious returns True. Postcondition: The current item has moved left one item Precondition: the list is not empty. Makes the first item the current item Precondition: the list is not empty. Makes the last item the current item L.first .last ( ) L.insertAfter (item) Inserts item after the current item, or as the only item if the list is empty. The new item is the current item. L.insertBefore (item) Inserts item before the current item, or as the only item if the list is empty. The new item is the current item. L.replace (newValue L.remove ( Precondition: the list is not empty. Replaces the current item by the newValue Precondition: the list is not empty. Removes and returns the current item. Making the next item the current item if one exists; otherwise the tail item in the list is the current item unless the list in now emm The cur sor_based list.py file contains a skeleton CursorBasedList class. You MUST uses a doubly-linked list implementation with a header node and a trailer node. All "real" list items will be inserted between the header and trailer nodes to reduce the number of "special cases" (e.g., inserting first item in an empty list, deleting the last item from the list, etc.). An empty list looks like header current trailer vious data next previous data next size0 Use the provided cur sorBasedListTester.py program to test your list. PART B: Once you have your Cur sorBasedList class finished, you are to write a simple text-editor program that utilizes your CursorBasedList classStep by Step Solution
There are 3 Steps involved in it
Step: 1
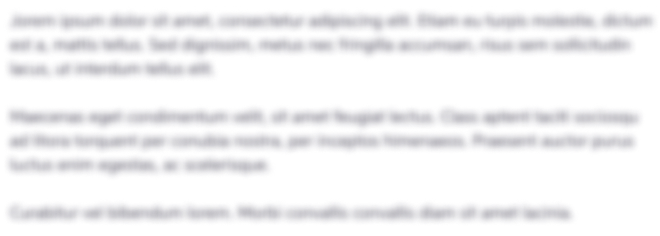
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started