Question
Pay stub application: Given the data name and hours worked, calculate gross pay, taxes, and net pay. Display a pay stub. For example, given: Sam_Jones,
Pay stub application:
Given the data name and hours worked, calculate gross pay, taxes, and net pay. Display a pay stub.
For example, given:
Sam_Jones, 45.5 (assume payrate is $7.25 because not given for this person)
Display:
***********************************************************
Sam_Jones Gross: $329.88
Taxes (25%): $82.47
Net: $247.41
***********************************************************
Next example, given:
Sally_Smith, 38.5, 10.50 (this time we have a third element for the pay rate)
Display:
***********************************************************
Sam_Jones Gross: $404.25
Taxes (25%): $101.06
Net: $303.19
***********************************************************
Write the following methods (note that getGross is overloaded overloading is required):
Return Type | Name | Parameters |
double | getGross | double workHours |
double | getGross | double workHours, double payRate |
double | getTaxes | double grossPay |
double | getNet | double grossPay, double taxes |
void | printPayStub | String name, double grossPay, double taxes, double netPay |
|
|
|
Pseudo code for your main method/loop
In your public static void main (String [] args) method:
declare variables for grossPay, taxes, netPay
declare variables for name, hoursWorked, payrate
declare a loop variable (e.g. boolean goAgain = true; you can name it what you want or use a sentinel)
while goAgain is true
get data for one employee from console or file (name, hours and payrate if applicable, if no payrate, enter -1 or 0, you decide how to handle no payrate)
call the appropriate getGross method assign the return value to your grossPay variable
declared above
(e.g. for Sam Jones it would be grossPay = getGross (45.5))
call the getTaxes method assign the return value to your taxes variable
call the getNetPay method assign the return value to your netPay variable
call the printPayStub method
ask user if they want to enter another persons data (update goAgain)
end while loop
TIP: Take this step by step. First, just read in the data with a loop.
Once that works, just do the grossPay, once that works, move on to taxes, etc.
Requirements
1. Write the five methods indicated above
2. Use a loop to control each employees entry
3. Complete the data entry and calculations (function calls) for gross, taxes, net
4. Call the printPayStub method your output should be formatted example:
Employee Name: Sam Jones
Gross Pay: $ xxxx.xx
Taxes Paid: $ xxxx.xx
Net Pay: $ xxxx.xx
Or something similar RIGHT ALIGN Your dollar amounts, see printf command.
6. Your methods should be written after your main method always put the main method first
7. Handle the following input:
Sam_Jones, 45.5
Sally_Smith , 38.5, 10.50
Tony_Casper, 38
Jane_Simmons, 50, 8.25
Clyde_Key, 35, 17.50
Next Project:
1. File I/0: create a text file to hold your data, read data from the file rather than the console, and write your stubs to a file
2. Handle overtime hours (pay the payrate *1.5 for the hours over 40)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
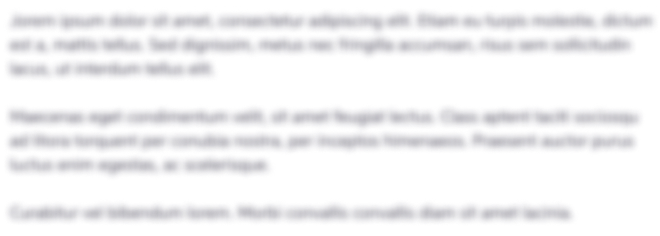
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started