Question
Periodic table of elements Objectives Learn how to read from and write to files Practice using data structures Practice top-down program design Practice re-using code
Periodic table of elements
Objectives
Learn how to read from and write to files
Practice using data structures
Practice top-down program design
Practice re-using code
Overview
For this lab, you will implement a program that can output information about any element in the periodic table of elements.
Requirements:
Read in a comma-separated values text file that contains the information about the elements in the periodic table.
Save those elements in a data structure for further processing.
Accept command-line parameters:
Program arguments shall be the chemical symbols for wish the user wants more information
Optionally, the user may provide as the first program argument, the name of a file to write the information out to.
Print out, or write to a file, information about the elements requested.
Data structure for elements
You will need to declare a data structure to hold the information about the chemical elements. Your structure will have the following components:
Atomic Number (integer)
Chemical Symbol (string)
Name (string)
Atomic Weight (floating point number)
State at room temp (string)
Bonding type (string)
Year discovered (string)
Incomplete Sample Code
You can download an incomplete sample code, though you don't have to completely follow it: incomplete sample code.
Read in file
First, download the element database file by clicking here: element_db.csv.
Save this file in the same directory as the source code for your lab.
The first thing your program should do is read in the element_db.csv file, line by line. Every line of the file represents one element in the periodic table of elements.
Open the .csv file for reading a text file, and read in every line of the file using the function fgets.
The file in csv format, which means the data fields are separated by commas. Use the function strtok to extract the data field by field.
You can use the function atoi to convert a string to an integer.
The function atof can be used to convert a string to a floating point number.
Save all of the elements scanned in into an array for further processing.
Remember to close the file once you have read in the contents.
Process program arguments
Your program needs to accept one or more command line program arguments. If the program is called with no arguments, it should print this error message and then exit:
ERROR: Please provide at least one program argument.
Each of the arguments provided to the program should be the chemical symbol of a chemical to look up information about (with one exception, mentioned below).
The program should check the first argument provided to see if it contains a . character. If it does, that argument may be assumed to be a filename, and the program should write the output to that file. You may want to use the function strchr to search for the . character.
For every chemical symbol argument, the program should look up the relevant information about that element.
You can do this by looping through the array of elements, using strcmp to check if the chemical symbol matches the program argument.
When you find a matching element, either print the information to the screen or write it out to the output file, depending if that first argument was a filename or not.
Note that you should be able to use the same function to accomplish this regardless of the target output stream, by using fprintf with the output file pointer or the built-in file pointer stdout.
If no element was found for the input symbol, print a message like this:
WARNING: No such element: [symbol]
(replace [symbol] with the name of the symbol that could not be found).
Print out the element in a similar format to the one used for Lab 9:
--------------- | 3 6.9410 | Li Lithium | solid | metallic | Found: 1817 ---------------
Print the atomic number and atomic weight on the top line, separated by a '\t' character.
Print the chemical symbol and name on the next line, separated by a '\t' character.
Print the physical state on the next line
Print the bonding type on the line after that
Print the date discovered on the last line, after the word "Found: ".
Print the top and bottom with the dash - character, and side of the box using the pipe | character.
Remember to close the output file once you have finished processing all of the command-line arguments.
Example Execution
No program arguments
[esus] $ ./lab10 ERROR: Please provide at least one program argument.
Output to screen: Iron, Silver, Carbon, Potassium
[esus] $ ./lab10 Fe C Ag K --------------- | 26 55.8450 | Fe Iron | solid | metallic | Found: Ancient --------------- --------------- | 6 12.0107 | C Carbon | solid | covalent network | Found: Ancient --------------- --------------- | 47 107.8682 | Ag Silver | solid | metallic | Found: Ancient --------------- --------------- | 19 39.0983 | K Potassium | solid | metallic | Found: 1807 ---------------
Output to screen: Mercury, Phosphorus, Flourine
[esus] $ ./lab10 Hg P F --------------- | 80 200.5900 | Hg Mercury | liquid | metallic | Found: Ancient --------------- --------------- | 15 30.9738 | P Phosphorus | solid | covalent network | Found: 1669 --------------- --------------- | 9 18.9984 | F Fluorine | gas | atomic | Found: 1670 ---------------
Output to file: Lithium, Aluminum, Iodine saved to file output.txt (note: no output is printed to screen).
[esus] $ ./lab10 output.txt Li Al I [esus] $
Content of output.txt:
[esus] $ cat output.txt --------------- | 3 6.9410 | Li Lithium | solid | metallic | Found: 1817 --------------- --------------- | 13 26.9815 | Al Aluminum | solid | metallic | Found: Ancient --------------- --------------- | 53 126.9045 | I Iodine | solid | covalent network | Found: 1811 ---------------
Program arguments have invalid symbol name:
[esus] $ ./lab10 S Hh H --------------- | 16 32.0650 | S Sulfur | solid | covalent network | Found: Ancient --------------- WARNING: No such element: Hh --------------- | 1 1.0079 | H Hydrogen | gas | diatomic | Found: 1766 ---------------
Compile & Test
Compile your program using this gcc command. c99 is a shortcut for running gcc -std=c99, which uses the C99 standard instead of the default C89 standard.
$ c99 -Wall lab10.c -o program10
NOTE: Make sure you output what is expected, nothing more and nothing less.
******incomplete sample code*****
#include#include #include #define ELEMENT_FILENAME "element_db.csv" typedef struct { int atomic_number; char symbol[4]; char name[25]; float atomic_weight; char state[25]; char bonding_type[25]; char discovered[25]; } element_t; element_t * find_element( element_t * list, char * symbol ); int main( int argc, char * argv[] ) { if ( argc < 2 ) { printf(" ERROR: Please provide at least one program argument. "); return 0; } element_t * elements = (element_t *)calloc( 118 , sizeof( element_t ) ); FILE * element_file = fopen( ELEMENT_FILENAME, "r" ); if ( element_file != NULL ) { char line[100]; while( fgets(line, 100, element_file ) != NULL ) { // remove newline character from line char * nl = strchr( line, ' ' ); if ( nl ) { *nl = '\0'; } //printf("line = %s ", line ); element_t e; char * str = strtok( line, "," ); int col = 0; while ( str != NULL ) { switch( col ) { case 0: e.atomic_number = atoi( str ); break; case 1: strcpy( e.symbol, str ); break; } str = strtok( NULL, "," ); col++; } elements[ e.atomic_number - 1 ] = e; //printf(" %s ", elements[ e.atomic_number - 1].symbol ); } fclose( element_file ); } // process program arguments. FILE * output_f = NULL; int i; for( i = 1; i < argc; i++ ) { if ( i == 1 ) // check for filename { char * dot = strchr( argv[i], '.' ); if ( dot ) { // this is a filename output_f = fopen( argv[i], "w" ); continue; } } // Look up this element element_t * ele = find_element( elements, argv[i] ); if ( ele ) { printf("%d %s ", ele->atomic_number, ele->symbol); } } return 0; } element_t * find_element( element_t * list, char * symbol ) { int i; for( i = 0; i < 118; i++ ) { if ( strcmp( list[i].symbol, symbol ) == 0 ) { element_t * result = &list[i]; return result; } } return NULL; }
*******element_db.csv*******
1 | H | Hydrogen | 1.00794 | gas | diatomic | 1766 |
2 | He | Helium | 4.002602 | gas | atomic | 1868 |
3 | Li | Lithium | 6.941 | solid | metallic | 1817 |
4 | Be | Beryllium | 9.012182 | solid | metallic | 1798 |
5 | B | Boron | 10.811 | solid | covalent network | 1807 |
6 | C | Carbon | 12.0107 | solid | covalent network | Ancient |
7 | N | Nitrogen | 14.0067 | gas | diatomic | 1772 |
8 | O | Oxygen | 15.9994 | gas | diatomic | 1774 |
9 | F | Fluorine | 18.9984032 | gas | atomic | 1670 |
10 | Ne | Neon | 20.1797 | gas | atomic | 1898 |
11 | Na | Sodium | 22.98976928 | solid | metallic | 1807 |
12 | Mg | Magnesium | 24.305 | solid | metallic | 1808 |
13 | Al | Aluminum | 26.9815386 | solid | metallic | Ancient |
14 | Si | Silicon | 28.0855 | solid | metallic | 1854 |
15 | P | Phosphorus | 30.973762 | solid | covalent network | 1669 |
16 | S | Sulfur | 32.065 | solid | covalent network | Ancient |
17 | Cl | Chlorine | 35.453 | gas | covalent network | 1774 |
18 | Ar | Argon | 39.948 | gas | atomic | 1894 |
19 | K | Potassium | 39.0983 | solid | metallic | 1807 |
20 | Ca | Calcium | 40.078 | solid | metallic | Ancient |
21 | Sc | Scandium | 44.955912 | solid | metallic | 1876 |
22 | Ti | Titanium | 47.867 | solid | metallic | 1791 |
23 | V | Vanadium | 50.9415 | solid | metallic | 1803 |
24 | Cr | Chromium | 51.9961 | solid | metallic | Ancient |
25 | Mn | Manganese | 54.938045 | solid | metallic | 1774 |
26 | Fe | Iron | 55.845 | solid | metallic | Ancient |
27 | Co | Cobalt | 58.933195 | solid | metallic | Ancient |
28 | Ni | Nickel | 58.6934 | solid | metallic | 1751 |
29 | Cu | Copper | 63.546 | solid | metallic | Ancient |
30 | Zn | Zinc | 65.38 | solid | metallic | 1746 |
31 | Ga | Gallium | 69.723 | solid | metallic | 1875 |
32 | Ge | Germanium | 72.64 | solid | metallic | 1886 |
33 | As | Arsenic | 74.9216 | solid | metallic | Ancient |
34 | Se | Selenium | 78.96 | solid | metallic | 1817 |
35 | Br | Bromine | 79.904 | liquid | covalent network | 1826 |
36 | Kr | Krypton | 83.798 | gas | atomic | 1898 |
37 | Rb | Rubidium | 85.4678 | solid | metallic | 1861 |
38 | Sr | Strontium | 87.62 | solid | metallic | 1790 |
39 | Y | Yttrium | 88.90585 | solid | metallic | 1794 |
40 | Zr | Zirconium | 91.224 | solid | metallic | 1789 |
41 | Nb | Niobium | 92.90638 | solid | metallic | 1801 |
42 | Mo | Molybdenum | 95.96 | solid | metallic | 1778 |
43 | Tc | Technetium | 98 | solid | metallic | 1937 |
44 | Ru | Ruthenium | 101.07 | solid | metallic | 1827 |
45 | Rh | Rhodium | 102.9055 | solid | metallic | 1803 |
46 | Pd | Palladium | 106.42 | solid | metallic | 1803 |
47 | Ag | Silver | 107.8682 | solid | metallic | Ancient |
48 | Cd | Cadmium | 112.411 | solid | metallic | 1817 |
49 | In | Indium | 114.818 | solid | metallic | 1863 |
50 | Sn | Tin | 118.71 | solid | metallic | Ancient |
51 | Sb | Antimony | 121.76 | solid | metallic | Ancient |
52 | Te | Tellurium | 127.6 | solid | metallic | 1782 |
53 | I | Iodine | 126.90447 | solid | covalent network | 1811 |
54 | Xe | Xenon | 131.293 | gas | atomic | 1898 |
55 | Cs | Cesium | 132.9054519 | solid | metallic | 1860 |
56 | Ba | Barium | 137.327 | solid | metallic | 1808 |
57 | La | Lanthanum | 138.90547 | solid | metallic | 1839 |
58 | Ce | Cerium | 140.116 | solid | metallic | 1803 |
59 | Pr | Praseodymium | 140.90765 | solid | metallic | 1885 |
60 | Nd | Neodymium | 144.242 | solid | metallic | 1885 |
61 | Pm | Promethium | 145 | solid | metallic | 1947 |
62 | Sm | Samarium | 150.36 | solid | metallic | 1853 |
63 | Eu | Europium | 151.964 | solid | metallic | 1901 |
64 | Gd | Gadolinium | 157.25 | solid | metallic | 1880 |
65 | Tb | Terbium | 158.92535 | solid | metallic | 1843 |
66 | Dy | Dysprosium | 162.5 | solid | metallic | 1886 |
67 | Ho | Holmium | 164.93032 | solid | metallic | 1878 |
68 | Er | Erbium | 167.259 | solid | metallic | 1842 |
69 | Tm | Thulium | 168.93421 | solid | metallic | 1879 |
70 | Yb | Ytterbium | 173.054 | solid | metallic | 1878 |
71 | Lu | Lutetium | 174.9668 | solid | metallic | 1907 |
72 | Hf | Hafnium | 178.49 | solid | metallic | 1923 |
73 | Ta | Tantalum | 180.94788 | solid | metallic | 1802 |
74 | W | Tungsten | 183.84 | solid | metallic | 1783 |
75 | Re | Rhenium | 186.207 | solid | metallic | 1925 |
76 | Os | Osmium | 190.23 | solid | metallic | 1803 |
77 | Ir | Iridium | 192.217 | solid | metallic | 1803 |
78 | Pt | Platinum | 195.084 | solid | metallic | Ancient |
79 | Au | Gold | 196.966569 | solid | metallic | Ancient |
80 | Hg | Mercury | 200.59 | liquid | metallic | Ancient |
81 | Tl | Thallium | 204.3833 | solid | metallic | 1861 |
82 | Pb | Lead | 207.2 | solid | metallic | Ancient |
83 | Bi | Bismuth | 208.9804 | solid | metallic | Ancient |
84 | Po | Polonium | 209 | solid | metallic | 1898 |
85 | At | Astatine | 210 | solid | covalent network | 1940 |
86 | Rn | Radon | 222 | gas | atomic | 1900 |
87 | Fr | Francium | 223 | solid | metallic | 1939 |
88 | Ra | Radium | 226 | solid | metallic | 1898 |
89 | Ac | Actinium | 227 | solid | metallic | 1899 |
90 | Th | Thorium | 232.03806 | solid | metallic | 1828 |
91 | Pa | Protactinium | 231.03588 | solid | metallic | 1913 |
92 | U | Uranium | 238.02891 | solid | metallic | 1789 |
93 | Np | Neptunium | 237 | solid | metallic | 1940 |
94 | Pu | Plutonium | 244 | solid | metallic | 1940 |
95 | Am | Americium | 243 | solid | metallic | 1944 |
96 | Cm | Curium | 247 | solid | metallic | 1944 |
97 | Bk | Berkelium | 247 | solid | metallic | 1949 |
98 | Cf | Californium | 251 | solid | metallic | 1950 |
99 | Es | Einsteinium | 252 | solid | 1952 | |
100 | Fm | Fermium | 257 | 1952 | ||
101 | Md | Mendelevium | 258 | 1955 | ||
102 | No | Nobelium | 259 | 1957 | ||
103 | Lr | Lawrencium | 262 | 1961 | ||
104 | Rf | Rutherfordium | 267 | 1969 | ||
105 | Db | Dubnium | 268 | 1967 | ||
106 | Sg | Seaborgium | 271 | 1974 | ||
107 | Bh | Bohrium | 272 | 1976 | ||
108 | Hs | Hassium | 270 | 1984 | ||
109 | Mt | Meitnerium | 276 | 1982 | ||
110 | Ds | Darmstadtium | 281 | 1994 | ||
111 | Rg | Roentgenium | 280 | 1994 | ||
112 | Cn | Copernicium | 285 | 1996 | ||
113 | Uut | Ununtrium | 284 | 2003 | ||
114 | Uuq | Ununquadium | 289 | 1998 | ||
115 | Uup | Ununpentium | 288 | 2003 | ||
116 | Uuh | Ununhexium | 293 | 2000 | ||
117 | Uus | Ununseptium | 294 | 2010 | ||
118 | Uuo | Ununoctium | 294 | 2002 |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
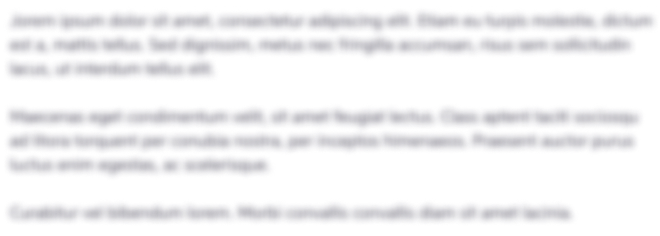
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started