Question
person_1.php sets up the data to be edited. However, the button labelled Update does not perform any function. Develop the functionality to update Person record
person_1.php sets up the data to be edited. However, the button labelled Update does not perform any function. Develop the functionality to update Person record to the updated values provided by the user. To clarify: when the user presses the Update button, the new values provided by the user are saved for that Person record. Do not allow the ID to be edited, and include a login authentication mechanism for accessing your page.
The newly updated program should also have functionality to edit in a file person_2.php, which performs the Update operation.
person_1.php:
// PHP script showing how to connect to MySQL. // Record for a person can be recorded, added, and edited $servername = dalec('IP'); $username = dalec('C9_USER'); $password = "d1f1"; $database = "c9"; $dbport = 3306;
// Create connection $db = new mysqli($servername, $username, $password, $database, $dbport);
// Check connection if ($db->connect_error) { die("Connection failed: " . $db->connect_error); } echo "Connected successfully (".$db->host_info.")"; $thisPHP = $_SERVER['PHP_SELF']; if (!isset($_POST['btnEdit'])) { echo <<
ID: Name: Phone: Email:
EOT; }
// Start executing the script $id = $_POST["id"]; $name = $_POST["name"]; $phone = $_POST["phone"]; $email = $_POST["emil"]; // At least name must be specified if (!empty($name)){ // Form sql string $sql = "insert into Person (ID, Name, Phone, Email) values ('$id', '$name', '$phone', '$email')"; if ($db->query ($sql) == TRUE) { echo "Record added "; } } // Check if delete is selected if (isset($_POST['btnDelete'])) { $id = $_POST['id']; $sql = "delete from Person where id='$id'"; if ($db->query ($sql) == TRUE) { echo "Record deleted "; } } else if (isset($_POST['btnEdit'])) { $sql = "select * from Person where id='$id'"; if (($result = $db->query ($sql)) == TRUE) { while($row = $result->fetch_assoc()) { $id = $row["ID"]; $name = $row["Name"]; $email = $row["Email"]; $phone = $row["Phone"]; } } echo <<
ID: Name: Phone: Email:
EOE; } // Show rows $sql = "SELECT * FROM Person"; $result = $db->query($sql);
if ($result->num_rows > 0) { // Output data of each row while($row = $result->fetch_assoc()) { $id = $row["ID"]; echo "id: " . $id . " - Name: " . $row["Name"] . " - Email: " . $row["Email"] . " - Phone: " . $row["Phone"] ; echo "
"; echo ""; echo " "; echo "
" . " "; } } else { echo "0 results"; } $db->close(); ?>
person_2.php:
// PHP script showing how to connect to MySQL. // Record for a person can be recorded, added, and edited $servername = dalec('IP'); $username = dalec('C9_USER'); $password = "d1f1"; $database = "c9"; $dbport = 3306;
// Create connection $db = new mysqli($servername, $username, $password, $database, $dbport);
// Check connection if ($db->connect_error) { die("Connection failed: " . $db->connect_error); } echo "Connected successfully (".$db->host_info.")"; $thisPHP = $_SERVER['PHP_SELF']; // Always show this, unless we are coming in with the Edit button pressed if (!isset($_POST['btnEdit'])) { echo <<
ID: Name: Phone: Email:
EOT; }
// Extract POST variables. Only set when new record is added $id = $_POST["id"]; $name = $_POST["name"]; $phone = $_POST["phone"]; $email = $_POST["email"]; // Check if the btnAdd is pressed if (isset($_POST['btnAdd'])){ // At least name must be specified if (!empty($name)){ // Form sql string $sql = "insert into Person (ID, Name, Phone, Email) values ('$id', '$name', '$phone', '$email')"; if ($db->query ($sql) == TRUE) { echo "Record added "; } } } // Check if delete is selected if (isset($_POST['btnDelete'])) { $sql = "delete from Person where id='$id'"; if ($db->query ($sql) == TRUE) { echo "Record deleted "; } } // Check if edit button is pressed if (isset($_POST['btnEdit'])) { $sql = "select * from Person where id='$id'"; if (($result = $db->query ($sql)) == TRUE) { while($row = $result->fetch_assoc()) { $id = $row["ID"]; $name = $row["Name"]; $email = $row["Email"]; $phone = $row["Phone"]; } } echo <<
ID: Name: Phone: Email:
EOE; } // Check if update button is pressed if (isset($_POST['btnUpdate'])) { $sql = "update Person SET Name='$name', Email='$email', Phone='$phone' where id='$id'"; if (($result = $db->query ($sql)) == TRUE) { echo "Record Updated "; } } // Show rows $sql = "SELECT * FROM Person"; $result = $db->query($sql);
if ($result->num_rows > 0) { // Output data of each row while($row = $result->fetch_assoc()) { $id = $row["ID"]; echo "id: " . $id . " - Name: " . $row["Name"] . " - Email: " . $row["Email"] . " - Phone: " . $row["Phone"] ; echo "
"; echo ""; echo " "; echo "
" . " "; } } else { echo "0 results"; } $db->close(); ?>
Step by Step Solution
There are 3 Steps involved in it
Step: 1
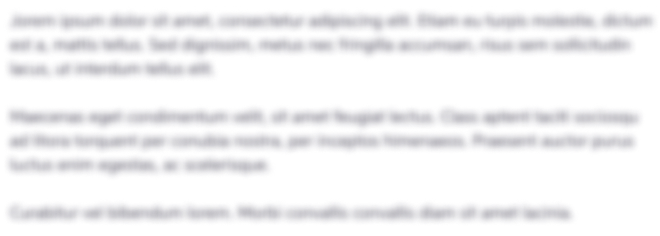
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started