Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Person.java /** Represents a person and their list of friends */ public class Person { private String firstName; private String friendList; private int friendCount; /**
Person.java /** Represents a person and their list of friends */ public class Person { private String firstName; private String friendList; private int friendCount; /** Creates a person with a given name and no friends in their list. * @param name the first name of the person of interest */ public Person(String name) { //-----------Start below here. To do: approximate lines of code = 3 // initialize instance variable firstName to name, initialize friendList to "" and friendCount to 0 //-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions. } /** Retrieves the names of all of a person's friends. * @return a string containing all of the names of a person's friends */ public String getFriendNames() { return friendList; } /** Adds a person to the list of friends of this person. * @param p the person whose name is to be added to the friend list */ public void befriend(Person p) { //-----------Start below here. To do: approximate lines of code = 2 // add (concatenate) the first name of Person p to the String friendList followed by a space " " // increment friendCount //-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions. } /** Removes a person from the list of friends of this person. * @param p the person whose name is to be removed from the friend list */ public void unfriend(Person p) { //-----------Start below here. To do: approximate lines of code = 2 // removes the first name of Person p from the friendList string. Decrement friendCount // Hint: use the replace method of class String. Don't forget to remove the space following the first name (see befriend above) // e.g. if first name is "xxx" then remove "xxx " //-----------------End here. Please do not remove this comment. Reminder: no changes outside the todo regions. } /** Returns the number of friends a person currently has. * @return a count of the number of friends the person has */ public int getFriendCount() { return friendCount; } }
PersonTester.java public class PersonTester { public static void main(String[] args) { Person individual = new Person("Frodo"); individual.befriend(new Person("Samwise")); individual.befriend(new Person("Aragorn")); individual.befriend(new Person("Boromir")); System.out.println("Friend List: " + individual.getFriendNames()); System.out.println("Expected: Samwise Aragorn Boromir "); System.out.println("Friend Count: " + individual.getFriendCount()); System.out.println("Expected: 3"); individual.unfriend(new Person("Boromir")); System.out.println("Friend List: " + individual.getFriendNames()); System.out.println("Expected: Samwise Aragorn "); System.out.println("Friend Count: " + individual.getFriendCount()); System.out.println("Expected: 2"); individual.befriend(new Person("Gandalf")); System.out.println("Friend List: " + individual.getFriendNames()); System.out.println("Expected: Samwise Aragorn Gandalf "); System.out.println("Friend Count: " + individual.getFriendCount()); System.out.println("Expected: 3"); individual.unfriend(new Person("Samwise")); System.out.println("Friend List: " + individual.getFriendNames()); System.out.println("Expected: Aragorn Gandalf "); System.out.println("Friend Count: " + individual.getFriendCount()); System.out.println("Expected: 2"); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
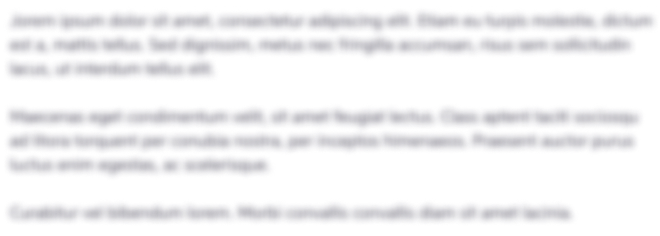
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started