Question
Please answer in c# with the code available to copy :) Program 3: Design (pseudocode) and implement (source code) a class (name it BankAccount) that
Please answer in c# with the code available to copy :)
Program 3: Design (pseudocode) and implement (source code) a class (name it BankAccount) that defines the following data fields and methods:
Private int data field named id to store the account ID (default value is 0).
-
Private double data field named balance to store the account balance (default value is 0.0).
-
Private double data field named annualInterestRate to store the interest rate (default value is 0.0%). Assume all accounts have same interest rate. Annual interest rate is percentage such as 3.2%, thus you need to divide by 100 to get double value 0.032).
-
Private Date data field named dateCreated to store the date when the account was created.
-
Non-argument constructor method that creates a default account (with default values).
-
Constructor method that creates an account with specified ID and initial balance.
-
Get and Set methods for variables id, balance, and annualInterestRate.
-
Get method for variable dateCreated.
-
Method named getMonthlyInterestRate() that returns the monthly interest rate (i.e., annualInterestRate / 12 , formatted as percentage (%)).
-
Method named getMonthlyInterest() that returns the earned monthly interest amount (i.e., balance * monthlyInterestRate, formatted as currency ($)).
-
Method named withdraw() that withdraws a specific amount from the account.
-
Method named deposit() that deposits a specific amount to the account.
-
Method toString()to printout a meaningful description of an account object using all of its instance variables in the following format:
Account ID: 123456
Account Balance: $7,000.00
Interest Rate: 2.50%
Date Opened: Sun Nov 2 14:18:16 EDT 2017
Now design (pseudocode) and implement (source code) a test program (name it TestBankAccount)to create an account object named myObject as follows:
- Account ID is 123456;
- The Initial balance is $10,000
- The annual interest rate is 2.5%.
- Withdraw $3,500
- Deposit $500
- Print out the account balance
- Print out the earned monthly interest
- Print out the date the account was created
please do in C#
Step by Step Solution
There are 3 Steps involved in it
Step: 1
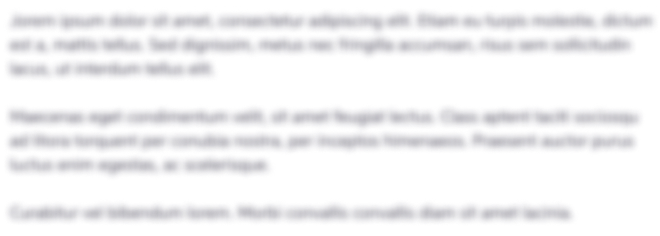
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started