Question
Please complete the following below in c++ coding: #include #include #include #include using namespace std; // Declaration of struct for Statistics. Do not modify. struct
Please complete the following below in c++ coding:
#include #include #include #include using namespace std;
// Declaration of struct for Statistics. Do not modify.
struct Statistics { int wordCount; int longestWord; int shortestWord; int numChars; };
// Declarations of functions in GivenFunctions.cpp. Do not modify.
void toCString (string s, char c[]); void displayText (string message, string title); void displayText2 (char s[], char title[]); bool isLetter (char ch); void toUpperCase(char s[]); void toLowerCase(char s[]); int getCharacters(char data[], char filename[]); int getWord (char data[], int start, char word[]); char byteToChar (int bits[]); void charToByte (int bits[], char ch);
// Declarations of functions for Assignment #2. Do not modify.
void encrypt (char document[], char filename[]); void decrypt (char document[], char filename[]); int readWordsDictionary(string dictionary[]); int checkSpelling (string dictionary[], int numWords, char document[], int start, char errorWord[]); int checkSpellingAll (string dictionary[], int numWords, char document[], string errorWords[]); int searchWord (char document[], int start, char keyWord[]); Statistics getStatistics (char document[]);
// This function accepts two C-string parameters, document and filename. // It opens the file specified by filename. It then encrypts each character // in the document C-string using the encryption algorithm and stores it // in the file.
void encrypt (char document[], char filename[]) {
displayText ("Encrypting ...", "Encrypt"); }
// This function accepts two C-strings as parameters, document and filename. // It opens the file specified by filename. It reads each character from the // file and stores it in the document C-string. The characters in the // document C-string are then decrypted using an algorithm that reverses the // encryption algorithm. void decrypt (char document[], char filename[]) { displayText ("Decrypting ...", "Decrypt"); }
// This function accepts the dictionary (array of string) as its only // parameter. It opens the dictionary file and reads all the words and // stores them in the dictionary array. It returns the amount of words // stored in the dictionary array. All the words in the dictionary are // in lowercase.
int readWordsDictionary(string dictionary[]) {
displayText ("Reading words from dictionary.txt ...", "Dictionary"); return 0; }
// This function accepts the dictionary as a parameter as well as a C-string containing // the characters of the document. It checks the spelling of all the words that come after // location start in the document C-string. If an incorrectly spelt word is encountered, // it is copied to the C-string errorWord and the function terminates by returning the // starting location of the word that has been spelt incorrectly. If there are no spelling // errors, the function must return -1.
int checkSpelling (string dictionary[], int numWords, char document[], int start, char errorWord[]) { displayText ("Searching for spelling errors ...", "Check Spelling");
return -1; }
// This function is similar to the checkSpelling function. It searches the entire document // C-string for incorrectly spelt words. Whenever an incorrectly spelt word is encountered, // it is inserted in the array of errorWords which was passed as a parameter. The function // returns the amount of words that were incorrectly spelt in the document.
int checkSpellingAll (string dictionary[], int numWords, char document[], string errorWords[]) { displayText ("Searching for spelling errors ...", "Check Spelling");
return 0; }
// This function searches the document C-string to find the // keyWord C-string, starting from location start. If the keyWord // substring is found in the document C-string, it returns the // starting location where it was found. Otherwise, it returns -1.
int searchWord (char document[], int start, char keyWord[]) {
displayText ("Searching for word ...", "Search"); return -1; }
// This function accepts the document C-string as a parameter, analyses it, and stores // the results of the analysis in a Statistics struct. This struct is then returned to // the caller.
Statistics getStatistics (char document[]) {
Statistics result;
displayText ("Generating statistics ...", "Statistics"); result.wordCount = 0; result.longestWord = 0; result.shortestWord = 0; result.numChars = 0; return result; }
Sample data of Dictionary.txt:
a a-horizon a-ok aardvark aardwolf ab aba abaca abacist aback abactinal abacus abaddon abaft abalienate abalienation abalone abampere abandon abandoned abandonment abarticulation abase
Given functions:
#include
void toCString (string s, char c[]); void displayText (string message, string title); void displayText2 (char s[], char title[]); bool isLetter (char ch); void toUpperCase(char s[]); void toLowerCase(char s[]); int getCharacters(char data[], char filename[]); int getWord (char data[], int start, char word[]); char byteToChar (int bits[]); void charToByte (int bits[], char ch);
// This function converts a variable of type string to a C-string.
void toCString (string s, char c[]) {
int length = s.length(); for (int i=0; i // This function displays the message passed as a parameter on a // pop-up window. The message is of type string. void displayText (string m, string t) { char message[100]; char title[100]; toCString(m, message); toCString(m, title); MessageBox(NULL, message, title, MB_ICONEXCLAMATION | MB_OK); } // This function displays the message passed as a parameter on a // pop-up window. The message is a C-string. void displayText2 (char message[], char title[]) { MessageBox(NULL, message, title, MB_ICONEXCLAMATION | MB_OK); } // This function returns true if the character passed as a // parameter is a letter, or false, otherwise. bool isLetter (char ch) { if ((ch >= 'A' && ch = 'a' && ch // This function accepts a C-string as a parameter and converts // all the lowercase letters (if any) to uppercase letters. void toUpperCase(char s[]) { int i; i = 0; while (s[i] != '\0') { if (s[i] >= 'a' && s[i] // This function accepts a C-string as a parameter and converts // all the uppercase letters (if any) to lowercase letters. void toLowerCase(char s[]) { int i; i = 0; while (s[i] != '\0') { if (s[i] >= 'A' && s[i] // This function accepts two C-strings as parameters, data and // filename. It opens the file with the given name and reads all // the characters in the file and stores them in the data C-string. int getCharacters(char data[], char filename[]) { ifstream encryptedFile; char ch; int i; encryptedFile.open(filename); if (!encryptedFile.is_open()) { string message = "File could not be opened for reading."; string title = "File Error"; displayText(message, title); return -1; } i = 0; encryptedFile >> noskipws; encryptedFile >> ch; while (!encryptedFile.eof()) { data[i] = ch; i = i + 1; encryptedFile >> ch; } data[i] = '\0'; encryptedFile.close(); return i; } // This function accepts two C-strings, data and word, as // parameters. It also accepts an integer, start, as a // parameter. From location start, it finds the first word // in data and stores it in word. It returns the location of // the character that comes after the word in the data C-string. // If it does not find a word, it returns 0. int getWord (char data[], int start, char word[]) { int i, j, size; size = strlen(data); i = start; while (i if (i == size) return 0; j = 0; while (isLetter(data[i])) { word[j]= data[i]; i = i + 1; j = j + 1; } word[j]= '\0'; return i; } // This function accepts an integer array of eight 1's and 0's // containing the byte representation of an ASCII character // and returns the actual ASCII character. char byteToChar (int bits[]) { int i, sum, power2; sum = 0; power2 = 1; for (i=0; i // This function accepts an ASCII character as a parameter // and finds the 8-bit (byte) representation of the character. // The byte representation is stored in the bits array. void charToByte (int bits[], char ch) { int charValue, i, remainder; charValue = ch; for (i=0; i Description This assignment requires you to write various functions for a simple Windows-based text editor. The user interface of the editor is shown below: *My Secret Editor Search: From Beginning Dear life, when I said: "Can my day get any worse?' It was rhetorical, not a challenge. I want to be like a caterpillar. Eat a lot. Sleep for a while. Wake up beautiful. # you're searching for that one person that will change your lite, take a look in the mirror f you think you are too small to be effective. you have never been in the dark with a mosquito." - Betty Reese "The difference betweeen genius and stupidity is: genius has its limits." Albert Einstein My therapist told me the way to achieve true inner peace is to finish what I start. So far I've finished two bags of M&Ms and a chocolate cake. I feel better already."-Dave Barry From Cursor Statistics File Operations: Check Spelling Open File -> From Beginning Save File As-> From Cursor Clear Editor All Words The functions you are required to write for this assignment are called when the buttons are clicked on the user interface. They are described under the following sections, File Operations, Check Spelling, Search, and Statistics. File Operations You must write two functions with the following headings: void encrypt (char document[], char filename[]) void decrypt (char document[], char filename[]) The user creates a document by typing characters in the editor. When the user is ready to save the document to a file, the file name must be entered in the field to the right of the Save File As button. The Save File As button is then clicked. The encrypt function is called by the user interface. When called, the characters in the document are passed as a C-string parameter, document, to the encrypt function. The name of the file is also passed as a C-string parameter. The encrypt function opens the file. Each character in the document C-string is then encrypted and stored in the file. The following encryption algorithm should be used to encrypt each character before it is stored in the file: (2) (3) Let length = the amount of characters in the document C-string. Let shift = length % 4+1 For each character in the document (3.1) add shift to that character. (3.2) interchange bit 3 with bit 4 in the character. (3.3) Store the character in the file. When the user wants to open a file, the file name must be entered in the field to the right of the Open File button. The Open File button is then clicked. The decrypt function is called by the user interface. When called, a C-string document, is passed as a parameter to the decrypt function. A C- string containing the name of the file is also passed as a parameter. The decrypt function opens the file. Each character from the file is then read, decrypted, and inserted in the document C-string which was passed as a parameter. The decryption algorithm should reverse the steps of the encryption algorithm When the user clicks on the Clear Editor button, the contents of the editor and file name/s are erased. You do not have to write code for this behaviour. Check Spelling You must write three functions with the following headings: int readWordsDictionary(string dictionary[]); int checkSpelling (string dictionary[], int numWords, char document[], int start, char errorword[]); int checkSpellingAll (string dictionary[], int numWords, char document[], string errorWords[]); The first function reads all the words from the dictionary file, dictionary.txt, and stores them in dictionary, an array of string passed as a parameter. It returns the amount of words in the dictionary, All the words in the dictionary are in lowercase. The function is called as soon as the program starts. The checkSpelling function accepts the dictionary as a parameter as well as a C-string containing the characters of the document. It checks the spelling of all the words that come after location start in the document C-string. If an incorrectly spelt word is encountered, it is copied to the C-string error Word and the function terminates by returning the starting location of the word that has been spelt incorrectly. If there are no spelling errors, the function must return-1. The checkSpelling All function is similar to the check Spelling function. However, it searches the entire document C-string for incorrectly spelt words. Whenever an incorrectly spelt word is encountered, it is inserted in the array of errorWords which was passed as a parameter. The function returns the amount of words that were incorrectly spelt in the document. It should be noted that the user may type uppercase and lowercase letters in the editor. So, "The and "the" are correctly spelt even though the dictionary only contains the word "the". Also, the get Word and getWords functions discussed in the lectures are good starting points for writing the checkSpelling and check Spelling All functions. Search The search feature allows the user to search for a particular word in the document. You must write a function with the following heading to perform the search: int searchword (char document[], int start, char keyword(1) The function must search the document C-string to find the keyword C-string, starting from location start. If the keyword substring is found in the document C-string, you must return the starting location where it was found. Otherwise, return-1. Statistics A Statistics struct is used to store statistics about the document in the edito". It is declared as follows: struct Statistics ( int wordCount; // amount of words in the document int longestWord; // length of the longest word in the document int shortestword; // length of the shortest word in the document int numChars; // total number of characters in the document You must write the following function to generate the statistics and store it in a variable of type Statistics: Statistics getStatistics (char document[]); The function accepts the document C-string, analyses it, and stores the resut in a Statistics struct which is returned to the caller. What You are given You are given the following files: Assignment2.dev // a special file called a project "ile GivenFunctions.cpp // contains useful functions from the lectures Graphics.cpp // contains functions which display the user interface Assignment2.cpp // write the functions for Assignment 2 in this file dictionary.txt // dictionary with 69,903 English words You must open the project file, Assignment2.dev, not the individual.cpp files. When the project file is opened, the three files.cpp files are shown in Dev-C++: Assignment2.cpp, GivenFunctions.cpp, and Graphics.cpp. You can view the code in GivenFunctions.cop and Graphics.cpp. However, you should not modify the code in both files. You must write the functions required for this assignment in Assignment2.cpp. Since the functions are being called from the user interface code in Graphics.cpp, if any of the function headings in Assignment2.cpp are modified, the program will not compile. The file Given Functions.cpp contains several functions that were discussed during the lectures (e.g. isletter, to UpperCase, byte ToChar, etc.). You are free to call these functions when writing your own. 3 Project Classes Debug - MySecretEditor Assignment2.cpp Graphics.cpp Project Classes Debug *' Statistics : struct checkSpelling (string checkSpelling All (str decrypt(char docume encrypt(char docume getStatistics (char do readWords Dictionar searchWord (char do Description This assignment requires you to write various functions for a simple Windows-based text editor. The user interface of the editor is shown below: *My Secret Editor Search: From Beginning Dear life, when I said: "Can my day get any worse?' It was rhetorical, not a challenge. I want to be like a caterpillar. Eat a lot. Sleep for a while. Wake up beautiful. # you're searching for that one person that will change your lite, take a look in the mirror f you think you are too small to be effective. you have never been in the dark with a mosquito." - Betty Reese "The difference betweeen genius and stupidity is: genius has its limits." Albert Einstein My therapist told me the way to achieve true inner peace is to finish what I start. So far I've finished two bags of M&Ms and a chocolate cake. I feel better already."-Dave Barry From Cursor Statistics File Operations: Check Spelling Open File -> From Beginning Save File As-> From Cursor Clear Editor All Words The functions you are required to write for this assignment are called when the buttons are clicked on the user interface. They are described under the following sections, File Operations, Check Spelling, Search, and Statistics. File Operations You must write two functions with the following headings: void encrypt (char document[], char filename[]) void decrypt (char document[], char filename[]) The user creates a document by typing characters in the editor. When the user is ready to save the document to a file, the file name must be entered in the field to the right of the Save File As button. The Save File As button is then clicked. The encrypt function is called by the user interface. When called, the characters in the document are passed as a C-string parameter, document, to the encrypt function. The name of the file is also passed as a C-string parameter. The encrypt function opens the file. Each character in the document C-string is then encrypted and stored in the file. The following encryption algorithm should be used to encrypt each character before it is stored in the file: (2) (3) Let length = the amount of characters in the document C-string. Let shift = length % 4+1 For each character in the document (3.1) add shift to that character. (3.2) interchange bit 3 with bit 4 in the character. (3.3) Store the character in the file. When the user wants to open a file, the file name must be entered in the field to the right of the Open File button. The Open File button is then clicked. The decrypt function is called by the user interface. When called, a C-string document, is passed as a parameter to the decrypt function. A C- string containing the name of the file is also passed as a parameter. The decrypt function opens the file. Each character from the file is then read, decrypted, and inserted in the document C-string which was passed as a parameter. The decryption algorithm should reverse the steps of the encryption algorithm When the user clicks on the Clear Editor button, the contents of the editor and file name/s are erased. You do not have to write code for this behaviour. Check Spelling You must write three functions with the following headings: int readWordsDictionary(string dictionary[]); int checkSpelling (string dictionary[], int numWords, char document[], int start, char errorword[]); int checkSpellingAll (string dictionary[], int numWords, char document[], string errorWords[]); The first function reads all the words from the dictionary file, dictionary.txt, and stores them in dictionary, an array of string passed as a parameter. It returns the amount of words in the dictionary, All the words in the dictionary are in lowercase. The function is called as soon as the program starts. The checkSpelling function accepts the dictionary as a parameter as well as a C-string containing the characters of the document. It checks the spelling of all the words that come after location start in the document C-string. If an incorrectly spelt word is encountered, it is copied to the C-string error Word and the function terminates by returning the starting location of the word that has been spelt incorrectly. If there are no spelling errors, the function must return-1. The checkSpelling All function is similar to the check Spelling function. However, it searches the entire document C-string for incorrectly spelt words. Whenever an incorrectly spelt word is encountered, it is inserted in the array of errorWords which was passed as a parameter. The function returns the amount of words that were incorrectly spelt in the document. It should be noted that the user may type uppercase and lowercase letters in the editor. So, "The and "the" are correctly spelt even though the dictionary only contains the word "the". Also, the get Word and getWords functions discussed in the lectures are good starting points for writing the checkSpelling and check Spelling All functions. Search The search feature allows the user to search for a particular word in the document. You must write a function with the following heading to perform the search: int searchword (char document[], int start, char keyword(1) The function must search the document C-string to find the keyword C-string, starting from location start. If the keyword substring is found in the document C-string, you must return the starting location where it was found. Otherwise, return-1. Statistics A Statistics struct is used to store statistics about the document in the edito". It is declared as follows: struct Statistics ( int wordCount; // amount of words in the document int longestWord; // length of the longest word in the document int shortestword; // length of the shortest word in the document int numChars; // total number of characters in the document You must write the following function to generate the statistics and store it in a variable of type Statistics: Statistics getStatistics (char document[]); The function accepts the document C-string, analyses it, and stores the resut in a Statistics struct which is returned to the caller. What You are given You are given the following files: Assignment2.dev // a special file called a project "ile GivenFunctions.cpp // contains useful functions from the lectures Graphics.cpp // contains functions which display the user interface Assignment2.cpp // write the functions for Assignment 2 in this file dictionary.txt // dictionary with 69,903 English words You must open the project file, Assignment2.dev, not the individual.cpp files. When the project file is opened, the three files.cpp files are shown in Dev-C++: Assignment2.cpp, GivenFunctions.cpp, and Graphics.cpp. You can view the code in GivenFunctions.cop and Graphics.cpp. However, you should not modify the code in both files. You must write the functions required for this assignment in Assignment2.cpp. Since the functions are being called from the user interface code in Graphics.cpp, if any of the function headings in Assignment2.cpp are modified, the program will not compile. The file Given Functions.cpp contains several functions that were discussed during the lectures (e.g. isletter, to UpperCase, byte ToChar, etc.). You are free to call these functions when writing your own. 3 Project Classes Debug - MySecretEditor Assignment2.cpp Graphics.cpp Project Classes Debug *' Statistics : struct checkSpelling (string checkSpelling All (str decrypt(char docume encrypt(char docume getStatistics (char do readWords Dictionar searchWord (char do
Step by Step Solution
There are 3 Steps involved in it
Step: 1
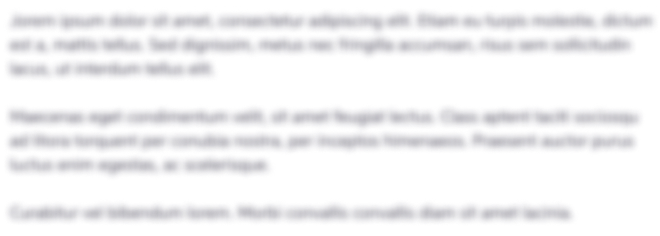
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started