Question
Please convert these from Java to Python. Thank you for your help! ------------------------------------------------------------------------------------------------------- //AccountTester.java /** This program tests the BankAccount class and its subclasses. */
Please convert these from Java to Python. Thank you for your help!
-------------------------------------------------------------------------------------------------------
//AccountTester.java
/**
This program tests the BankAccount class and
its subclasses.
*/
public class AccountTester
{
public static void main(String[] args)
{
SavingsAccount momsSavings
= new SavingsAccount(0.5);
CheckingAccount harrysChecking
= new CheckingAccount(100);
momsSavings.deposit(10000);
// Transfer $2000
momsSavings.withdraw(2000);
harrysChecking.deposit(2000);
harrysChecking.withdraw(1500);
harrysChecking.withdraw(80);
// Transfer $1000
momsSavings.withdraw(1000);
harrysChecking.deposit(1000);
harrysChecking.withdraw(400);
// Simulate end of month
momsSavings.addInterest();
harrysChecking.deductFees();
System.out.println("Mom's savings balance = $"
+ momsSavings.getBalance());
System.out.println("Harry's checking balance = $"
+ harrysChecking.getBalance());
// Inheritance TimeDepositAccount
System.out.println("Test with Penalty");
TimeDepositAccount tda1 = new TimeDepositAccount ( 5, 3 );
tda1.deposit(1000);
tda1.addInterest(); //monthly: month 1
tda1.addInterest(); // month 2
System.out.println("Before withdrawal ($500): " + tda1.getBalance());
tda1.withdraw(500);
System.out.println("After withdrawal ($500): " +tda1.getBalance());
System.out.println("Test without Penalty");
TimeDepositAccount tda2 = new TimeDepositAccount ( 5, 3 );
tda2.deposit(1000);
tda2.addInterest(); //monthly: month 1
tda2.addInterest(); // month 2
tda2.addInterest(); // month 3
System.out.println("Before withdrawal ($500): " + tda2.getBalance());
tda2.withdraw(500);
System.out.println("After withdrawal ($500): " +tda2.getBalance());
// Polymorphism, abstract, toString
// BankAccount not = new BankAccount(); // NOTE: cannot instantiate a BankAccount; must be a subclass
BankAccount[] accounts = {momsSavings, harrysChecking, tda1, tda2};
for (int i=0; i
{
accounts[i].deposit(25);
System.out.println("Account: " + accounts[i]);
}
}
}
-------------------------------------------------------------------------------------------------------
//BankAccount.java
/**
A bank account has a balance that can be changed by
deposits and withdrawals.
*/
public abstract class BankAccount
{
private double balance;
/**
Constructs a bank account with a zero balance.
*/
public BankAccount()
{
balance = 0;
}
/**
Constructs a bank account with a given balance.
@param initialBalance the initial balance
*/
public BankAccount(double initialBalance)
{
balance = initialBalance;
}
/**
Deposits money into the bank account.
@param amount the amount to deposit
*/
public void deposit(double amount)
{
balance = balance + amount;
}
/**
Withdraws money from the bank account.
@param amount the amount to withdraw
*/
public void withdraw(double amount)
{
balance = balance - amount;
}
/**
Gets the current balance of the bank account.
@return the current balance
*/
public double getBalance()
{
return balance;
}
/**
Overrides the default toString method of Object
@return the class of the object and its instance fields
*/
public String toString()
{
return getClass().getName() + "[" + "balance=" + balance + "]";
}
}
-------------------------------------------------------------------------------------------------------
//CheckingAccount.java
/** A checking account that charges transaction fees. */ public class CheckingAccount extends BankAccount {
private int transactionCount;
private static final int FREE_TRANSACTIONS = 3; private static final double TRANSACTION_FEE = 2.0;
/** Constructs a checking account with a given balance. @param initialBalance the initial balance */ public CheckingAccount(double initialBalance) { // Construct superclass super(initialBalance); // Initialize transaction count transactionCount = 0; }
public void deposit(double amount) { transactionCount++; // Now add amount to balance super.deposit(amount); } public void withdraw(double amount) { transactionCount++; // Now subtract amount from balance super.withdraw(amount); }
/** Deducts the accumulated fees and resets the transaction count. */ public void deductFees() { if (transactionCount > FREE_TRANSACTIONS) { double fees = TRANSACTION_FEE * (transactionCount - FREE_TRANSACTIONS); super.withdraw(fees); } transactionCount = 0; }
/** * Returns a string representation of a CheckingAccount * @return string representing a CheckingAccount with inherited fields */
public String toString() { return super.toString() + "[transactionCount=" + transactionCount + "]"; } }
-------------------------------------------------------------------------------------------------------
//SavingsAccount.java
/** An account that earns interest at a fixed rate. */ public class SavingsAccount extends BankAccount {
private double interestRate;
/** Constructs a bank account with a given interest rate. @param rate the interest rate */ public SavingsAccount(double rate) { interestRate = rate; }
/** Adds the earned interest to the account balance. */ public void addInterest() { double interest = getBalance() * interestRate / 100; deposit(interest); } /** * Returns a string representation of a SavingsAccount * @return string representing a SavingsAccount including inherited instance fields */
public String toString() { return super.toString() + "[interestRate= " + interestRate + "]"; } }
-------------------------------------------------------------------------------------------------------
//TimeDepositAccount.java
/*
* To change this template, choose Tools | Templates
* and open the template in the editor.
*/
/**
* TimeDepositAccount represents a CD.
*/
public class TimeDepositAccount extends SavingsAccount {
private int monthsToMaturity;
private static double EARLY_WITHDRAWAL_PENALTY= 20;
/**
* Constructs a new TimeDepositAccount
* @param initRate initial interest rate
* @param months months to maturity
*/
TimeDepositAccount(double initRate, int months){
super(initRate);
monthsToMaturity = months;
}
/**
* Adds interest to the time deposit account,
* reducing the number of months to maturity.
*/
public void addInterest()
{
monthsToMaturity--;
super.addInterest();
}
/**
* Withdraws amount from time deposit account,
* charging an early withdrawal penalty if not mature
* @param amount amount to withdraw
*/
public void withdraw(double amount)
{
if (monthsToMaturity > 0)
super.withdraw(EARLY_WITHDRAWAL_PENALTY);
super.withdraw(amount);
}
/**
* Returns a string representation of a TimeDepositAccount
* @return string representing TimeDepositAccount with inherited instance fields
*/
public String toString()
{
return super.toString() + "[monthsToMaturity= " + monthsToMaturity + "]";
}
}
BankAccount double balance BankAccount +BankAccount (double initialBal) +void deposit(double amount) tvoid withdraw (double amount) +double getBalance) 0 SavingsAccount CheckingAccount SavingsAccount -double interestRate -int transactionCount interestRate 0.05 +CheckingAccount (double initialBal) tvoid deposit (double amount) tvoid withdraw (double amount) +void deductFees () +SavingsAccount (double rate) tvoid addInterest () ul Time ositAccount TimeDepositAccount -int monthsToMaturity -static double EARLY WITHDRAWAL PENALTY monthsToMaturity +TimeDepositAccount (double initRate, int months) tvoid addInterest () tvoid withdraw (double amount) TimeDepositAccount tda = new TimeDepositAccount ( 0.05, 3 ) BankAccount double balance BankAccount +BankAccount (double initialBal) +void deposit(double amount) tvoid withdraw (double amount) +double getBalance) 0 SavingsAccount CheckingAccount SavingsAccount -double interestRate -int transactionCount interestRate 0.05 +CheckingAccount (double initialBal) tvoid deposit (double amount) tvoid withdraw (double amount) +void deductFees () +SavingsAccount (double rate) tvoid addInterest () ul Time ositAccount TimeDepositAccount -int monthsToMaturity -static double EARLY WITHDRAWAL PENALTY monthsToMaturity +TimeDepositAccount (double initRate, int months) tvoid addInterest () tvoid withdraw (double amount) TimeDepositAccount tda = new TimeDepositAccount ( 0.05, 3 )Step by Step Solution
There are 3 Steps involved in it
Step: 1
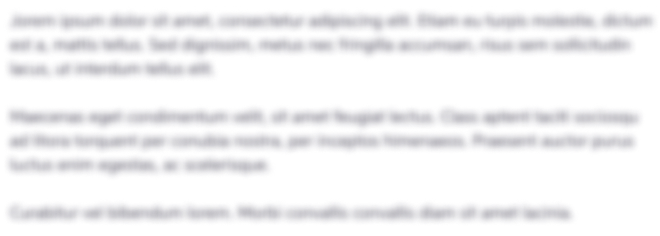
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started