Question
Please find all the even numbers from [1, 100] and put them in the stack then display the stack. After that pop the numbers in
Please find all the even numbers from [1, 100] and put them in the stack then display the stack. After that pop the numbers in the stack until all the numbers in the stack are under 25. Below you will find the contents of Stack.h and Stack.cpp
/*--Stack.h--*/
#include
#ifndef STACK
#define STACK
const int STACK_CAPACITY = 128;
typedef int StackElement;
class Stack
{
public:
/***** Function Members *****/
/***** Constructor *****/
Stack();
/*----------------------------------------------------------------------
--
Construct a Stack object.
Precondition: None.
Postcondition: An empty Stack object has been constructed (myTop is
initialized to -1 and myArray is an array with STACK_CAPACITY
elements of type StackElement).
-----------------------------------------------------------------------
*/
bool empty() const;
/*----------------------------------------------------------------------
--
Check if stack is empty.
Precondition: None
Postcondition: Returns true if stack is empty and false otherwise.
-----------------------------------------------------------------------
*/
void push(const StackElement & value);
/*----------------------------------------------------------------------
--
Add a value to a stack.
Precondition: value is to be added to this stack
Postcondition: value is added at top of stack provided there is space;
otherwise, a stack-full message is displayed and execution is
terminated.
-----------------------------------------------------------------------
*/
void display(ostream & out) const;
/*----------------------------------------------------------------------
--
Display values stored in the stack.
Precondition: ostream out is open.
Postcondition: Stack's contents, from top down, have been output to
out.
-----------------------------------------------------------------------
*/
StackElement top() const;
/*----------------------------------------------------------------------
--
Retrieve value at top of stack (if any).
Precondition: Stack is nonempty
Postcondition: Value at top of stack is returned, unless the stack is
empty; in that case, an error message is displayed and a "garbage
value" is returned.
-----------------------------------------------------------------------
*/
void pop();
/*----------------------------------------------------------------------
--
Remove value at top of stack (if any).
Precondition: Stack is nonempty.
Postcondition: Value at top of stack has been removed, unless the
stack
is empty; in that case, an error message is displayed and
execution allowed to proceed.
-----------------------------------------------------------------------
*/
private:
/***** Data Members *****/
StackElement myArray[STACK_CAPACITY];
int myTop;
}; // end of class declaration
#endif
/*--Stack.cpp--*/
#include
using namespace std;
#include "Stack.h"
//--- Definition of Stack constructor
Stack::Stack()
: myTop(-1)
{}
//--- Definition of empty()
bool Stack::empty() const
{
return (myTop == -1);
}
//--- Definition of push()
void Stack::push(const StackElement & value)
{
if (myTop < STACK_CAPACITY - 1) //Preserve stack invariant
{
++myTop;
myArray[myTop] = value;
}
else
{
cerr << "*** Stack full -- can't add new value *** "
"Must increase value of STACK_CAPACITY in Stack.h ";
exit(1);
}
}
//--- Definition of display()
void Stack::display(ostream & out) const
{
for (int i = myTop; i >= 0; i--)
out << myArray[i] << endl;
}
//--- Definition of top()
StackElement Stack::top() const
{
if ( !empty() )
return (myArray[myTop]);
else
{
cerr << "*** Stack is empty -- returning garbage value *** ";
return (myArray[STACK_CAPACITY - 1]);
}
}
//--- Definition of pop()
void Stack::pop()
{
if ( !empty() )
myTop--;
else
cerr << "*** Stack is empty -- can't remove a value *** ";
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
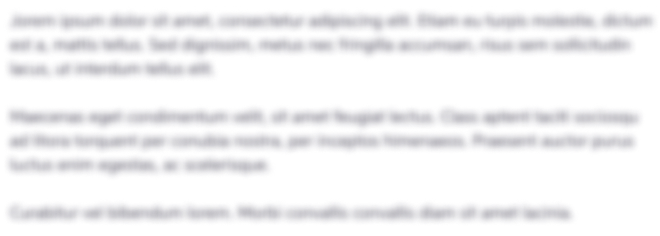
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started