Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Please follow the steps to write a small project in kotlin This is the code that was done before: Step 1 : Create Player Interface
Please follow the steps to write a small project in kotlin
This is the code that was done before:
Step : Create Player Interface and Concrete Player Classes
Player.kt
interface Player
val currentHP: Int
val identity: String
class Lordoverride val currentHP: Int : Player
override val identity: String "Lord"
class Loyalistoverride val currentHP: Int : Player
override val identity: String "Loyalist"
class Spyoverride val currentHP: Int : Player
override val identity: String Spy
class Rebeloverride val currentHP: Int : Player
override val identity: String "Rebel"
Step : Modify General Class to Implement Decorator Pattern
General.kt
class Generalval player: Player : Player by player
Removed currentHP property
Updated to delegate properties to the underlying player object
init
printlnGeneral $playeridentity created."
println$playeridentity $playeridentity a $playeridentity has $playercurrentHP health point."
Step : Extend GeneralFactory for Creating Different Players
Factory.kt
object GeneralFactory : GeneralFactory
override fun createPlayern: Int: Player
return when n
Lord
Loyalist
Rebel
Spy
else throw IllegalArgumentExceptionInvalid player number: $n
object LordFactory : GeneralFactory
override fun createPlayern: Int: Player
return when n
Lord
else throw IllegalArgumentExceptionInvalid player number for Lord: $n
Other factories LoyalistFactory RebelFactory, SpyFactory can be similarly implemented
Step : Modify GeneralManager to Handle Single Lord
GeneralManager.kt
class GeneralManager
fun createGeneralsnumPlayers: Int
printlnCreating $numPlayers players:"
for i in numPlayers
val player GeneralFactory.createPlayeri
You can modify the output according to your needs
printlnTotal number of players: $numPlayers"
Inside main function
fun main
val manager GeneralManager
manager.createGenerals
Step : Implement Adapter for GuanYu Class
Existing class
class GuanYu
val maximumHP
Adapter class
class GuanYuAdapterprivate val guanYu: GuanYu : Player
override var currentHP: Int guanYu.maximumHP
override val identity: String "Guan Yu
Optionally, you can implement methods specific to GuanYu if needed
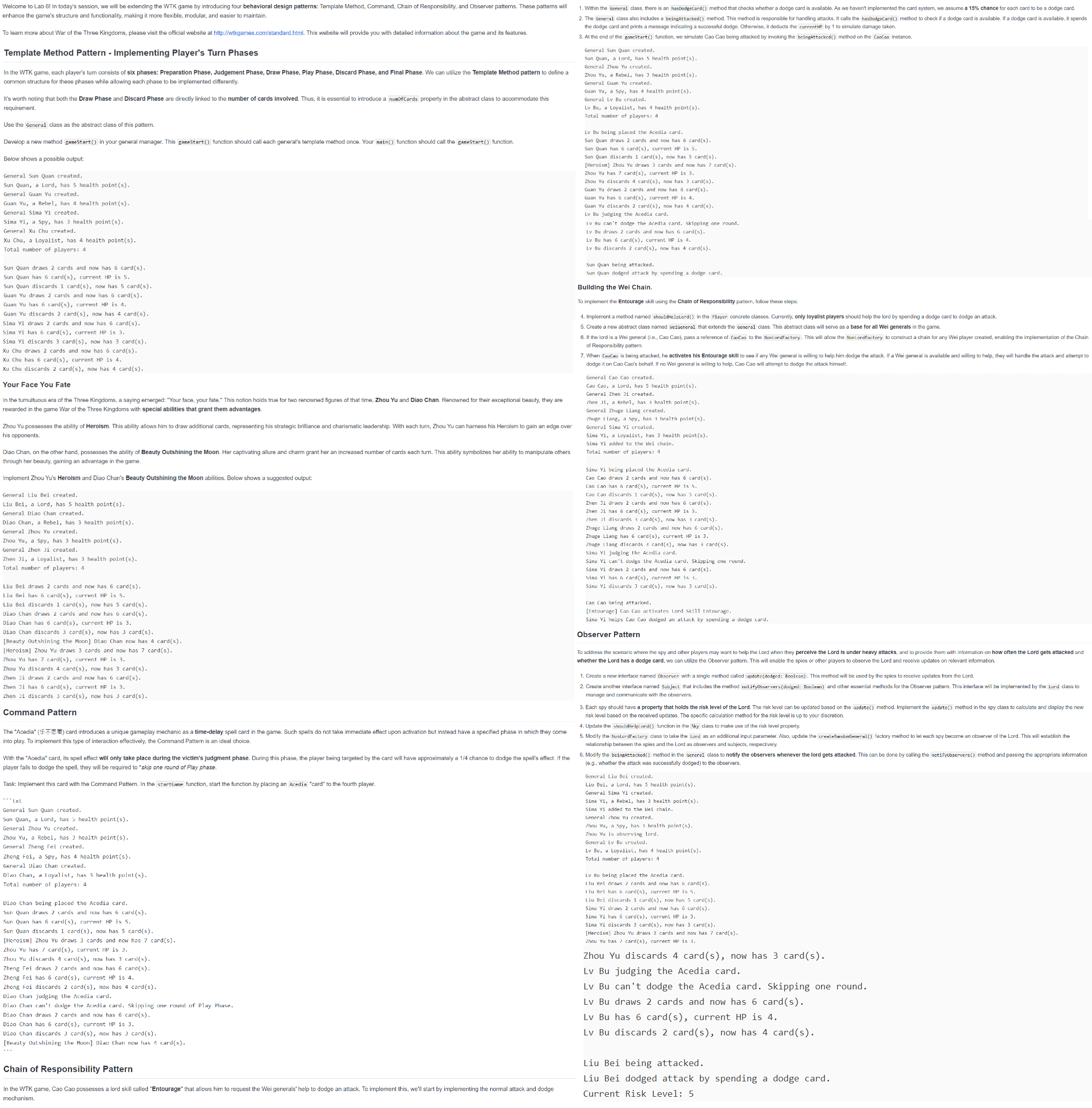
Step by Step Solution
There are 3 Steps involved in it
Step: 1
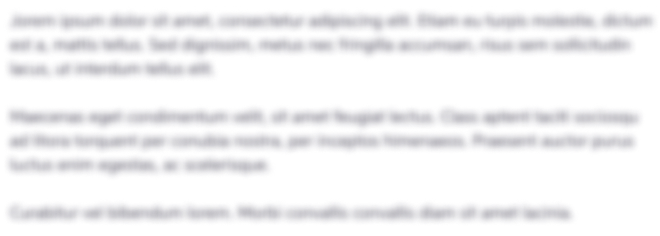
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started