Question
PLEASE HELP Can anyone help me with this c++ assignment? I have partial code given already below. I have to use inheritance by creating a
PLEASE HELP Can anyone help me with this c++ assignment? I have partial code given already below. I have to use inheritance by creating a class hierarchy for staff members. PLEASE HELP
I have to create the following files:
StaffMemberParser.h, StaffMember.h, HourlyEmployee.h, Volunteer.h, FullTimeEmployee.h
StaffMember class:
StaffMember is a class, which represents the basic attributes of any member in a company. It is used as the root of the company member hierarchy. It has the following attributes (should be protected):
firstName (String), lastName (String), memberId (String), pay (double)
All functions should be declared as public. The following constructor function should be provided to initialize the instance variables: StaffMember(string, string, string) The firstName, lastName, and memberId are initialized to the value of the first parameter, the second parameter, and the third parameter, respectively. The pay should be initialized to 0.0. The following accessor function should be provided for memberId : string getMemberId() The class StaffMember also has an abstract/pure virtual function (which should be implemented by its child classes, Volunteer, FullTimeEmployee, and HourlyEmployee classes) to compute the pay of the member: virtual void computePay() = 0; The following public function should be provided: virtual void printInfo() this function function prints a string of the following format: First name:\t\tBarack
Last name:\t\tObama
Member ID:\t\t000001
Pay:\t\t\t$30.00 You can make use of string manipulators fixed and setprecision in iomanip.h file
Volunteer Class: Volunteer is a subclass of StaffMember class. It represents a volunteer that works without any pay. There is no additional instance variable, but there are additional functions to the inherited ones. Its functions should be declared public. The following constructor function should be provided: Volunteer(string, string, string) This constructor calls the constructor of its parent. The following function should be implemented: virtual void computePay() It computes the pay for the volunteer. The pay is 0.0. Also, the following function should be implemented: virtual void printInfo() This function inherited from StaffMember class should be used to print a new string, and display a volunteer's information using the following format: Volunteer:
First name:\t\tBarack
Last name:\t\tObama
Member ID:\t\t000001
Pay:\t\t\t$0.00 This printInfo function should make use of the printInfo function of the parent class.
FullTimeEmployee class FullTimeEmployee is a subclass of StaffMember class. It represents a full time employee that can also receive bonus in addition to his/her salary. It has the following attribute in addition to the inherited ones: rate (double), bonus (double)
Its functions should be declared public. The following constructor function should be provided: FullTimeEmployee(string, string, string, double, double) The firstName, lastName, and memberId are initialized to the value of the first parameter, the second parameter, and the third parameter, respectively. The pay should be initialized to 0.0. Thus they are done by calling the constructor of the parent. The rate and bonus are initialized to the value of the forth and fifth parameters, respectively. The following function should be implemented: virtual void computePay() It computes the pay for the full time employee by adding rate and bonus. Also, the following function should be implemented: virtual void printInfo() This function inherited from StaffMember class should be used to print a new string, and display a full time employee's information using the following format: Full Time Employee:
First name:\t\tBarack
Last name:\t\tObama
Member ID:\t\t000001
Pay:\t\t\t$350.00
Rate:\t\t\t$300.00 Bonus:\t\t\t$50.00 This printInfo function should make use of the printInfo function of the parent class.
HourlyEmployee class HourlyEmployee is a subclass of StaffMember class. It represents an hourly paid employee in a company. It has the following additional attributes: rate (double), hoursWorked (int)
Its functions should be declared public. The following constructor function should be provided: HourlyEmployee(string, string, string, double, int) The firstName, lastName, and memberId are initialized to the value of the first parameter, the second parameter, and the third parameter, respectively. The pay should be initialized to 0.0. Thus they are done by calling the constructor of the parent. The rate and hoursWorked are initialized to the value of the forth and fifth parameters, respectively. The following function should be implemented: virtual void computePay() It computes the pay of the hourly employee. (computed by rate*hourWorked) Also, the following function should be implemented: virtual void printInfo() The printInfo() function inherited from the StaffMember class should be used to print a new string, and display an hourly employee's information using the following format: Hourly Employee:
First name:\t\tBarack Last name:\t\tObama
Member ID:\t\t000001
Pay:\t\t\t$150.00
Rate:\t\t\t$30.00
Hours:\t\t\t5
StaffMemberParser class The StaffMemberParser class is a utility class that will be used to create a pointer to a staff member object (one of a volunteer object, a full time employee object, and an hourly employee object) from a parsable string. The StaffMemberParser class object will not be instantiated. It must have the following public function: static StaffMember * parseStringToMember(string lineToParse) The parseStringToMember function's argument will be a string in the following format: For a volunteer, type/firstName/lastName/employeeId For a full time employee, type/firstName/lastName/emloyeeId/rate/bonus For an hourly employee, type/firstName/lastName/emloyeeId/rate/hoursWorked A real example of this string would be: Volunteer/Barack/Obama/000001 OR FullTimeEmployee/George/Bush/200005/400000/50000 OR HourlyEmployee/Bill/Clinton/000002/25.25/10 The StaffMemberParser will parse this string, pull out the information, allocates memory dynamically to a new object of one of Volunteer, FullTimeEmployee, or HourlyEmployee using their constructor with attributes of the object (depending on whether the first substring is Volunteer, FullTimeEmployee, or HourlyEmployee), and return it to the calling function. The type will always be present and always be one of Volunteer, FullTimeEmployee, and HourlyEmployee. You may add other functions to the Volunteer, FullTimeEmployee, and HourlyEmployee classes in order to make your life easier.
Main function description: The driver program will allow the user to interact with your other class modules. The purpose of this module is to handle all user input and screen output. The main function should start by displaying this updated menu in this exact format: Choice\t\tAction
------\t\t------
A\t\tAdd Member
C\t\tCompute Pay
D\t\tSearch for Member
L\t\tList Member
Q\t\tQuit
?\t\tDisplay Help Next, the following prompt should be displayed: What action would you like to perform? Read in the user input and execute the appropriate command. After the execution of each command, redisplay the prompt. Commands should be accepted in both lowercase and uppercase. Add Member Your program should display the following prompt: Please enter a member information to add: Read in the information and parse it using the staff member parser. Then add the new object of one of volunteer, full time employee, or hourly employee (created by staff member parser) to the member list. Compute Pay Your program should compute pay for all members created so far by calling computePay() function. After computing pay, display the following: pay computed Search for Member Your program should display the following prompt: Please enter a memberID to search: Read in the string and look up the member list, if there exists a member object with the same member ID, then display the following: member found Otherwise, display this: member not found List Members List the members in the member list. Make use of printInfo function defined in child classes of the StaffMember class.
If there is no member in the member list, then display following: no member Quit Your program should stop executing. Display Help Your program should redisplay the "choice action" menu. Invalid Command If an invalid command is entered, display the following line: Unknown action
Here is the given code, it is missing a little bit of code but it says what code is needed:
#include
#include
#include
#include
#include "StaffMember.h"
#include "StaffMemberParser.h" using namespace std; void printMenu(); int main()
{
char input1;
string inputInfo;
bool operation;
vector
{
cout << "What action would you like to perform?" << endl;
cin >> input1; switch (input1)
{
case 'A': //Add Member
cout << "Please enter a member information to add: ";
cin >> inputInfo;
/***********************************************************************************
ADD your code here to create a pointer to an object of one of child classes of StaffMember class and add it to the memberList ***********************************************************************************/
break;
case 'C': //Compute Pay
/*********************************************************************************** ***
ADD your code here to compute the pay for all members the memberList.
***********************************************************************************/
cout << "pay computed ";
break;
case 'D': //Search for Member
cout << "Please enter a memberID to search: ";
cin >> inputInfo;
operation = false;
/*********************************************************************************** ***
ADD your code here to search a given memberID. If found, set "found" true,
*** and set "found" false otherwise.
***********************************************************************************/
if (operation == true)
cout << "member found ";
else
cout << "member not found ";
break;
case 'L': //List Members
/*********************************************************************************** ***
ADD your code here to print out all member objects. If there is no member,
*** print "no member "
***********************************************************************************/
break;
case 'Q': //Quit
for (int j=0; j < memberList.size(); j++)
{
delete memberList.at(j);
}
break;
case '?': //Display Menu
printMenu();
break;
default:
cout << "Unknown action ";
break;
} } while (input1 != 'Q' && input1 != 'q'); // stop the loop when Q is read } /** The method printMenu displays the menu to a user **/
void printMenu()
{
cout << "Choice\t\tAction ";
cout << "------\t\t------ ";
cout << "A\t\tAdd Member ";
cout << "C\t\tCompute Pay ";
cout << "D\t\tSearch for Member ";
cout << "L\t\tList Members ";
cout << "Q\t\tQuit ";
cout << "?\t\tDisplay Help ";
}
I have an example output if needed
Step by Step Solution
There are 3 Steps involved in it
Step: 1
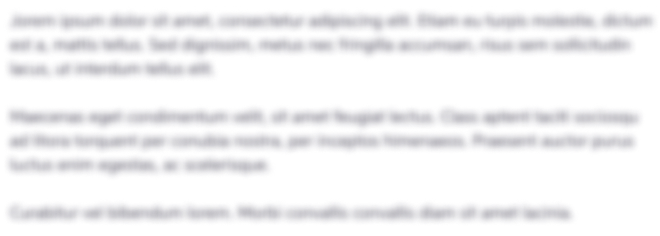
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started