Question
PLEASE HELP ME FIND A BETTER SOLUTION FOR HASHING ON JAVA WITHOUT ANY COLLISIONS (Perfect Hashing given set of strings) You are going to be
PLEASE HELP ME FIND A BETTER SOLUTION FOR HASHING ON JAVA WITHOUT ANY COLLISIONS (Perfect Hashing given set of strings)
You are going to be creating a hash function for a String. Your hash function should produce a number between 0 and 35 (inclusive), and there are more points for creating a function whose maximum is lower. Although you may discuss the assignment with others, points are also awarded for the uniqueness of your algorithm.
Your output should list each hash value followed by the corresponding String.
Compute the hash values of the following Strings:
DOMINICAN, STARS, BASEBALL, BASKETBALL, BOWLING, CROSS COUNTRY, GOLF, SOCCER, SOFTBALL, TENNIS, VOLLEYBALL, MEN, WOMEN, IGINLI, CHAMPIONSHIP
TOURNAMENT, ARENA, FIELD, COURT, ALLEY, NET, SCORE, HOME, AWAY
Requirements:
- You can use methods for a String, like getting a char (which, remember, can be cast to an int, since its just a number), or getting the length. You may not use the Strings hashCode method.
- You may use any combination of the hash methods described in class (modular hashing, folding, arithmetic operations (+, *, etc.) and bitwise operations (&, |, ^, <<, >>), but you may not use other methods (exponential functions, etc).
- Your hash method should not use if statements (I dont want you to, for example, write an if to say NET should return 0, SCORE should return 1, etc).
- The hash value of a particular word must be the same every time the program is run, even if the words are rearranged.
Your hash function should produce integers between 0 and 35.
2 points are subtracted for every collision (every String after the first that has the same hash value)
----------------------------------------------------------------------------------------------------------------------------------
Here is my code:
import java.io.File; import java.util.Scanner;
public class hashsample { public static void main(String[] args) { int count = 0; String input; String[] words = new String[24]; try { Scanner fileIn = new Scanner(new File("words.txt")); while (fileIn.hasNext()) { input = fileIn.nextLine(); words[count] = input; count++; } fileIn.close(); } catch (Exception ex) { System.out.println("Error: " + ex.getMessage()); ex.printStackTrace(); } for (String word : words) { int hashValue = calculateHash(word); System.out.printf("%-15s %3d%n", word, hashValue); } } public static int calculateHash(String str) { int sum = 0; for (int i = 0; i < str.length(); i++) { sum = sum + (str.charAt(i) - 'A') * (str.charAt(i) - 'A') * (i + 1); /* explanation: str.charAt(i) returns value of a char present at index i in the string. example: ASCII value of B is 66. substracting 'B' - 'A' yields to 1. which is the corresponding position of B in the letters 'A' based on ASCII value (definite values - Example: A will always be 65) */ } return (sum * 41323) % 25 ^ 7; }
}
--------------------------------------------------
Output from the code above:
DOMINICAN 23 STARS 1 BASEBALL 12 BASKETBALL 7 BOWLING 19 CROSS COUNTRY 14 GOLF 21 SOCCER 14 SOFTBALL 8 TENNIS 19 VOLLEYBALL 1 MEN 14 WOMEN 23 IGINLI 9 CHAMPIONSHIP 15 TOURNAMENT 15 ARENA 18 FIELD 8 COURT 12 ALLEY 5 NET 0 SCORE 10 HOME 6 AWAY 1
Step by Step Solution
There are 3 Steps involved in it
Step: 1
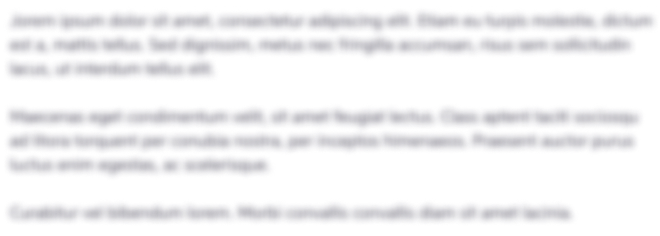
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started