Question
Please help me with programming question: I have to complete 4 methods in the TreeTraversal.java file based on the two given jave file: (BinarySearchTree?.java and
Please help me with programming question: I have to complete 4 methods in the TreeTraversal.java file based on the two given jave file: (BinarySearchTree?.java and INode?.java). For further infor., look up the link: https://www.eecs.yorku.ca/course_archive/2017-18/W/2030/labs/lab8/lab8.html
TreeTraversal.java file:
import java.util.ArrayList; import java.util.LinkedList; import java.util.List; import java.util.Queue;
/** * A utility class that provides methods for traversing a binary * search tree. * */ public class TreeTraversal { private TreeTraversal() { // empty by design }
/** * Returns the list of strings formed by traversing the specified tree using * an inorder traversal. * * @param tree * a binary search tree * @return the list of strings formed by traversing the specified tree using * an inorder traversal */ public static List
/** * Returns the list of strings formed by traversing the specified tree using * a preorder traversal. * * @param tree * a binary search tree * @return the list of strings formed by traversing the specified tree using * a preorder traversal */ public static List
/** * Returns the list of strings formed by traversing the specified tree using * a postorder traversal. * * @param tree * a binary search tree * @return the list of strings formed by traversing the specified tree using * a postorder traversal */ public static List
/** * Returns the list of strings formed by traversing the specified tree using * a breadth first traversal. The traversal visits nodes of the tree * starting at the root moving left to right for each level of the tree. * * @param tree * a binary search tree * @return the list of strings formed by traversing the specified tree using * a breadth first traversal */ public static List
// in Java, a LinkedList is a Queue // to enqueue a node, use the Queue method add // to dequeue a node, use the Queue method remove Queue
return result; }
/** * Returns the list of strings formed by traversing a tree having the * specified root using an inorder traversal. * * @param root * the root of the tree * @return the list of strings formed by traversing a tree having the * specified root using an inorder traversal */ private static List
/** * Returns the list of strings formed by traversing a tree having the * specified root using a preorder traversal. * * @param root * the root of the tree * @return the list of strings formed by traversing a tree having the * specified root using a preorder traversal */ private static List
return result; }
/** * Returns the list of strings formed by traversing a tree having the * specified root using a postorder traversal. * * @param root * the root of the tree * @return the list of strings formed by traversing a tree having the * specified root using a postorder traversal */ private static List
return result; }
}
BinarySearchTree.java file:
public class BinarySearchTree
/** * A class that represents nodes in the binary search tree. Each node is an * aggregation of a data element, and has a left and right child node. This * class is a top-level public class for testing purposes only; normally, Node * would be a private inner class. * * @param
/** * Create a node with the given data element. The left and right child nodes * are set to null. * * @param data * the element to store */ public Node(E data) { this.data = data; this.left = null; this.right = null; }
/** * Get the left child node. * * @return the left child node */ public Node
/** * Get the right child node. * * @return the right child node */ public Node
/** * Get the data stored in the node. * * @return the data stored in the node */ public E data() { return this.data; }
/** * Set the left child of this node to the specified node. * * @param left * the left child of this node */ public void setLeft(Node
/** * Set the right child of this node to the specified node. * * @param right * the right child of this node */ public void setRight(Node
/** * Set the data of this node to the specified element. * * @param element * the data for this node */ public void setData(E element) { this.data = element; } } private Node
/** * Create an empty binary search tree. */ public BinarySearchTree() { this.root = null; }
/** * Add an element to the tree. The element is inserted into the tree in a * position that preserves the definition of a binary search tree. * * @param element * the element to add to the tree */ public void add(E element) { if (this.root == null) { this.root = new Node
/** * Add an element to the subtree rooted at root
. The * element is inserted into the tree in a position that preserves the * definition of a binary search tree. * * @param element * the element to add to the subtree * @param root * the root of the subtree */ private static
/** * Returns true
if the tree contains the given element, * false
otherwise. * * @param element * the element to search for * @return true
if the tree contains the given element, * false
otherwise */ public boolean contains(E element) { return contains(element, this.root); }
/** * Returns true
if the subtree rooted at * root
contains the given element, false
* otherwise. * * @param element * the element to search for * @param root * the root of the subtree * @return true
if the subtree rooted at * root
contains the given element, * false
otherwise */ private static
/** * Return a string representation of the tree. * *
* The string is made up of the elements stored in the tree separated by * commas; the entire list of elements is enclosed in braces. The elements * are in ascending sorted order. * * @see java.lang.Object#toString() * * @return a string representation of the tree */ @Override public String toString() { return "{" + toString(this.root) + "}"; }
/** * Return a string representation of the subtree rooted at * root
. * *
* The string is made up of the elements stored in the tree separated by * commas where the elements are in ascending sorted order. * *
* The string is generated using inorder processing of the subtree: * *
- *
- the string corresponding to
root.left()
is computed * - the string corresponding to
root.data()
is computed * - the string corresponding to
root.right()
is * computed *
* The returned string is the concatenation of the three strings computed by * the inorder processing of the subtree. * * @param root * the root of the subtree * @return the string representation of the subtree */ private static
/** * Get the root node of the tree. For testing purposes only. * * @return the root node of the tree. */ public INode
}
INode?.java file:
public class INode
private BinarySearchTree.Node
/** * Get the right child node. * * @return the right child node */ public INode
/** * Get the data stored in the node. * * @return the data stored in the node */ public E data() { return this.node.data(); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
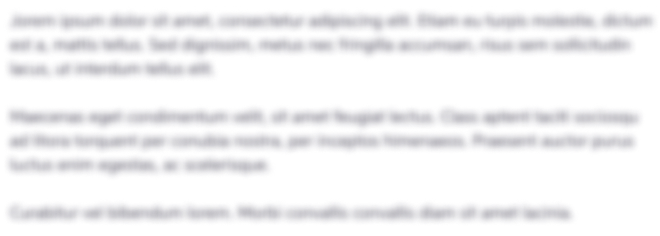
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started