Question
Please help me with the driver program and the display method. Should replicate the output. Assignment: Write an implementation of the LinkedOrderedList class. LinkedListDriver package
Please help me with the driver program and the display method. Should replicate the output.
Assignment: Write an implementation of the LinkedOrderedList class.
LinkedListDriver
package jsjf;
import java.util.Scanner;
public class LinkedListDriver {public static void main(String[] args) {Scanner input=new Scanner(System.in); LinkedList
System.out.print("Enter your choice: "); menu=Integer.parseInt(input.next()); switch(menu) { case 1: break; case 2: break; case 3: break; case 4:
break;
} } while(menu!=5); //iterates until 5 (Exit) is entered input.close(); } }
LinearNode.java
package jsjf;
public class LinearNode
public LinearNode() {next = null; element = null; }
public LinearNode(E elem) {next = null; element = elem; }
public LinearNode
public void setNext(LinearNode
public E getElement() {return element; }
public void setElement(E elem) {element = elem; } }
LinkedList.java
package jsjf;
import jsjf.exceptions.*; import java.util.*;
public abstract class LinkedList
public LinkedList() { count = 0; head = tail = null; modCount = 0; }
public T removeFirst() throws EmptyCollectionException { if (isEmpty()) { System.out.println("The collection is empty!"); } else { LinearNode
public T removeLast() throws EmptyCollectionException { if (isEmpty()) { System.out.println("The collection is empty!"); } else { LinearNode
while (current.getNext() != null) { previous = current; current = current.getNext(); } LinearNode
public T remove(T targetElement) throws EmptyCollectionException, ElementNotFoundException { if (isEmpty()) throw new EmptyCollectionException("LinkedList");
boolean found = false; LinearNode
while (current != null && !found) if (targetElement.equals(current.getElement())) found = true; else { previous = current; current = current.getNext(); }
if (!found) throw new ElementNotFoundException("LinkedList");
if (size() == 1) // only one element in the list head = tail = null; else if (current.equals(head)) // target is at the head head = current.getNext(); else if (current.equals(tail)) // target is at the tail { tail = previous; tail.setNext(null); } else // target is in the middle previous.setNext(current.getNext());
count--; modCount++;
return current.getElement(); }
public T first() throws EmptyCollectionException { if (isEmpty()) { System.out.println("The collection is empty!"); } else { return head.getElement(); } return null; }
public T last() throws EmptyCollectionException { if (isEmpty()) { System.out.println("The collection is empty!"); } else { return tail.getElement(); } return null; }
public boolean contains(T targetElement) throws EmptyCollectionException { if (isEmpty()) { System.out.println("The collection is empty!"); } else { boolean found = false; LinearNode
while (current != null && !found) if (targetElement.equals(current.getElement())) found = true; else current = current.getNext(); return found; } return false;
}
public boolean isEmpty() { return (count == 0); }
public int size() { return count; }
public String toString() { LinearNode
while (current != null) { result = result + current.getElement() + " "; current = current.getNext(); } return result; }
public Iterator
private class LinkedListIterator implements Iterator
public LinkedListIterator() { current = head; iteratorModCount = modCount; }
public boolean hasNext() throws ConcurrentModificationException { if (iteratorModCount != modCount) throw new ConcurrentModificationException();
return (current != null); }
public T next() throws ConcurrentModificationException { if (!hasNext()) throw new NoSuchElementException();
T result = current.getElement(); current = current.getNext(); return result; }
public void remove() throws UnsupportedOperationException { throw new UnsupportedOperationException(); } }
}
LinkedOrderedList.java
package jsjf;
import jsjf.exceptions.*;
public class LinkedOrderedList
public void add(T element) { if (!(element instanceof Comparable)) { throw new NonComparableElementException("LinkedOrderedList"); } else { Comparable
LinearNode
ListADT.java
package jsjf;
import java.util.Iterator;
public interface ListADT
public T removeLast();
public T remove(T element);
public T first();
public T last();
public boolean contains(T target);
public boolean isEmpty();
public int size();
public Iterator
public String toString(); }
OrderedListADT.java
package jsjf;
public interface OrderedListADT
ElementNotFoundException.java
package jsjf.exceptions;
public class ElementNotFoundException extends RuntimeException {public ElementNotFoundException (String collection) {super ("The target element is not in this " + collection); } }
EmptyCollectionException.java
package jsjf.exceptions;
public class EmptyCollectionException extends RuntimeException {public EmptyCollectionException(String collection) {super("The " + collection + " is empty."); } }
NonComparableElementException.java
package jsjf.exceptions;
public class NonComparableElementException extends RuntimeException {public NonComparableElementException (String collection) {super ("The " + collection + " requires Comparable elements."); } }
Sample Output
Step by Step Solution
There are 3 Steps involved in it
Step: 1
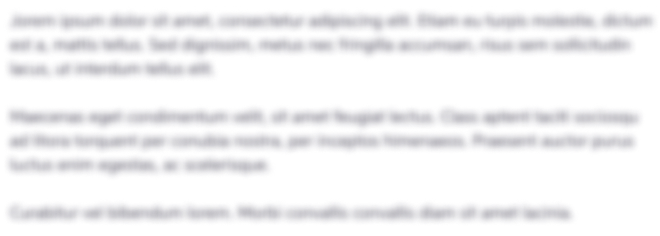
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started