Question
PLEASE help me with this assignment. For this assignment, you will continue to improve your MyVector class. To do so, templatize your MyVector class so
PLEASE help me with this assignment.
For this assignment, you will continue to improve your MyVector class.
To do so, templatize your MyVector class so that it will work with any data type, not just integers.
Then, have the overloaded array operator ( [] ) and the at method throw an exception, the string literal "OUT OF BOUNDS", if an invalid index is used.
Place all of the code for your class in a single file named MyVector.h and submit only this file (class templates don't like being split up across files).
Hints:
The generic data type has to be different for operator<< than it does for the MyVector class. If you use the same one, you'll get an error message about shadowed generic types.
THIS IS THE LASS ASSIGNMENT WITH ITS CLASS. MyVector.h
#ifndef MYVECTOR_H #define MYVECTOR_H #includeusing std::ostream; class MyVector { private: int* list, list_size, max_size; public: MyVector(); ~MyVector(); void push_back(int); void pop_back(); int& at(int); void clear(); int size() const; MyVector(const MyVector&); bool operator<(const MyVector&) const; bool operator==(const MyVector&) const; MyVector operator+(const MyVector&) const; int& operator[](int); MyVector operator++(int); MyVector& operator=(const MyVector&); friend ostream& operator<<(ostream&, MyVector&); }; #endif
THIS IS MyVector.cpp
#include "MyVector.h" MyVector::MyVector() { list_size = 0; max_size = 10; list = new int[max_size]; } MyVector::~MyVector() { delete [] list; } void MyVector::push_back(int i) { if(list_size < max_size) { list[list_size] = i; list_size++; } } void MyVector::pop_back() { if(list_size > 0) list_size--; } int& MyVector::at(int i) { return list[i]; } void MyVector::clear() { list_size = 0; } int MyVector::size() const { return list_size; } bool MyVector::operator<(const MyVector& r) const { return list_size < r.list_size; } bool MyVector::operator==(const MyVector& r) const { bool same = true; if(list_size != r.list_size) same = false; else { for(int i = 0; i < list_size; i++) if(list[i] != r.list[i]) { same = false; break; } } return same; } MyVector MyVector::operator+(const MyVector& r) const { MyVector temp; int j = list_size > r.list_size ? list_size : r.list_size; for(int i = 0; i < j; i++) temp.push_back(list[i] + r.list[i]); return temp; } int& MyVector::operator[](int i) { return list[i]; } MyVector MyVector::operator++(int) { MyVector temp = *this; for(int i = 0; i < temp.size(); i++) list[i]++; return temp; } MyVector& MyVector::operator=(const MyVector& r) { if(this != &r) { if(list) delete [] list; list_size = r.list_size; max_size = r.max_size; list = new int[max_size]; for(int i = 0; i < list_size; i++) list[i] = r.list[i]; } return *this; } MyVector::MyVector(const MyVector& r) { list = nullptr; *this = r; } ostream& operator<<(ostream& o, MyVector& r) { o << "["; if( r.size() > 0 ) { int i = 0; for(i; i < r.size() - 1; i++) o << r[i] << ", "; o << r[i]; } o << "]"; return o; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
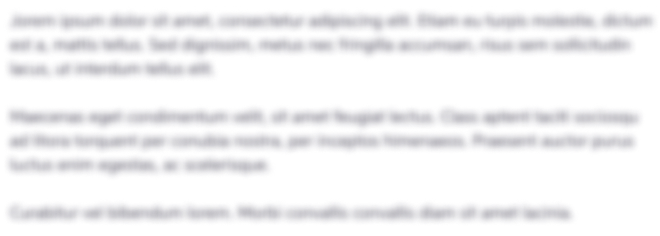
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started