Question
Please help with Java code. Here is a helpful piece of code to get a better understanding of getting the files from a .txt file
Please help with Java code.
Here is a helpful piece of code to get a better understanding of getting the files from a .txt file for input into the code. Copy and paste this into your Java software and compile/run the program. Separately, create a plain text file called 'test.txt' and input these numbers:
5
51
2112
42
12
-123
Once that is complete, run this code below and input 'test.txt' when prompted for input filename.
import java.util.Scanner; import java.io.File; import java.io.FileNotFoundException; public class TestingFileInput { public static void main (String args[]) throws FileNotFoundException { // Create a regular Scanner for keyboard input Scanner keyboard = new Scanner(System.in); // Now create a String variable and read the filename System.out.println("Enter input filename: "); String inFile; // Notice the use of the System.in Scanner for this input inFile = keyboard.next(); // Need to create a File variable based on the inFile file name File file = new File(inFile); // Now create a Scanner attached to the file instead of System.in // This Scanner will be used for file input!!! Scanner fileInput = new Scanner(file); // Read something out of the file, which is an integer int v1 = fileInput.nextInt(); System.out.println("First int of the file is : " + v1); // Set up a loop that reads the rest of the int data from the file System.out.println ( "Now reading the rest of the data!" ); for (int which = 0; which
Here is the rest of the program I need help with. Thanks in advance!
Here is the starter code to work with:
import java.util.Scanner; import java.io.File; import java.io.FileNotFoundException;
public class FileOfDates { /** * It's the main method! * @param args Arguments to the main method * @throws Exception is a file-related exception we're not processing */
public static void main(String args[]) throws FileNotFoundException { // The Scanner named get is for keyboard input Scanner get = new Scanner(System.in); System.out.print("Enter filename to process: "); String fn = get.next();
// The File thing attempts to connect to a file named in variable fn File thing = new File(fn); // The Scanner named fileInput is for reading data from the user Scanner fileInput = new Scanner(thing); // The following statement changes default behavior of this file // Scanner; see assignment for more information fileInput.useDelimiter("[/\\s]+");
}
}
This is the Date.class file that you can use for Date class. You don't need to rewrite the methods here as they are pasted below for you to work with.
Here is the Date.class code:
public class Date {
// Declare your fields here
/** * The default constructor builds a date of 1/1/1753 and should utilize * the appropriate setter methods to change the date. It should also * set the date to be "valid". */
public Date() {
}
/** * The specific constructor sets the date to whatever the parameters are. * It should assume the date is valid, and then if it is found to be * invalid, it should set the date to 1/1/1753. The method should * use the appropriate setter methods. * * @param m The month to attempt to set * @param d The day to attempt to set * @param y The year to attempt to set */
/** * setDate() attempts to set the date to whatever the parameters are. * It should assume the date is valid, and then if it is found to be * invalid, it should set the date to 1/1/1753. The method should * use the appropriate setter methods. * * @param m The month to attempt to set * @param d The day to attempt to set * @param y The year to attempt to set * @return A boolean true or false if the date is valid */
public boolean setDate( int m, int d, int y ) {
}
/** * setMonth() attempts to set the month to a valid value. * If the parameter is a valid month, the field is set to that value. * If the parameter is not valid, the field should be set to 1 and * the date should be set to be invalid. * * @param m The month to attempt to set */
private void setMonth( int mm ) {
}
/** * setDay() attempts to set the day of the month to a valid value. * If the day is valid, based on month and year, then the field is * set to that value. * If the day is not valid, the field should be set to 1 and * the date should be set to be invalid. * * @param m The month * @param d The day to attempt to set * @param y The year */
/** * setYear() attempts to set the year to a valid value. * If the parameter is a valid year (1753-3000 inclusive), the field is * set to that value. * If the parameter is not valid, the field should be set to 1 and * the date should be set to be invalid. * * @param y The year to attempt to set */
/** * getMonth() simply returns the value of the month field. */ public int getMonth() {
}
/** * getDay() simply returns the value of the day field. */
/** * getYear() simply returns the value of the year field. */
/** * setValid() will set the valid field to the parameter sent to it. * * @param v a boolean value to set */
/** * isValid() simply returns the value of the valid field. */
/** * dayOfYear() will return the day of the year of the date stored in the * object. The method should take into account leap years. * * @return A value between 1 and 366 */ public int dayOfYear() {
}
/** * daysSince1753() will return the number of days that have elapsed * since 1/1/1753. * * @return A value between 1 and 455821 */
/** * isLeapYear() will return if the year stored in the date is a leap * year or not. * * @return true if the year is a leap year, false otherwise */
/** * equals() will return if the current object's date is equal to the * date of another Date object. * * @param Date Another Date object to cmopare against * @return true if the two dates are equal, false otherwise */ public boolean equals( Date o ) { return ( this.getMonth() == o.getMonth() && this.getDay() == o.getDay() && this.getYear() == o.getYear() ); }
/** * toString() will return a String that is the date stored in the object * formatted as MM/DD/YYYY. * * @return A formatted String in MM/DD/YYYY format */
public String toString() { return String.format("%02d/%02d/%02d", getMonth(), getDay(), getYear() ); } }
Here is one of the .txt files for testing the program.
d6.txt
6 2/23/2096 5/29/2100 12/12/2831 11/8/2833 7/7/2972 5/29/2100
Determining Closest and Furthest Dates Closest Dates The closest dates will be determined by comparing each date to all of the dates in the file except for itself. The result may be 0 , but only if there are duplicate dates in the file. Furthest Dates The furthest dates will also be determined by comparing each date to all of the dates in the file except itself. Given the storage range of dates possible in a Date object, the maximum number of days between dates could be 455821 Examples See the sample runs at the end of the file for examples of different results. Considerations for Entering and Validating Dates Entering Dates For previous assignments when entering dates, the month, day, and year needed to be entered separately. For this assignment, you'll change a property of the Scanner attached to the file that you create so that you can read dates in MM/DD/YYYY format. By default, a Scanner uses whitespace like spaces and newlines (enter/return) to identify different pieces of input, like this: Java Scanner get new Scanner System. in) System.out.print("Enter your date: ") int m get.nextInt int d get.nextInt) int y get.nextInt)Step by Step Solution
There are 3 Steps involved in it
Step: 1
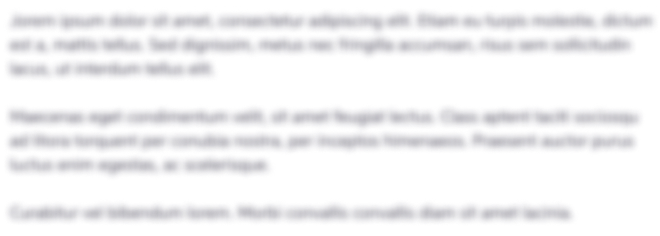
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started