Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Please help with Java (Milestone 2 only) Java code: import java.util.*; class Product { String name; int id; float price; Product(Strting name, int id, float
Please help with Java (Milestone 2 only)
Java code:
import java.util.*; class Product { String name; int id; float price; Product(Strting name, int id, float price) { // constructor this.name = name; this.id = id; this.price = price; } // gettedrs int getId(){ return this.id; } String getName(){ return this.name; } float getPrice(){ return this.price; } } class Inventory { // for each product we will use a parallel array to store the quantity ArrayListquantity = new ArrayList (); ArrayList products = new ArrayList (); Scanner sc = new Scanner(System.in); Inventory() { // initialize the inventory } void addProduct(Product obj) { // method to add a new product to the inventory products.add(obj); quantity.add(0); } int getStocks(int id) { // given a product id, return the stock of that product int index; for(Product i : products) { if(i.getId() == id) { // then find the index of that id index = products.indexOf(i); return(quantity.get(index));// return the quantity of that product } } return 0; // invalid stock } void addStock(int id, int addedStockValue) { // add the specified amount of stock to given id. if id is not found create a new id add the stock to it int index; for(Product i : products) { if(i.getId() == id) { // then find the index of that id index = products.indexOf(i); int value = quantity.get(index); //get the current value of the stock // add the new stock to that product quantity.set(index, value+addedStockValue); return; } } // if the loop ends , then the product is not there, so create anew System.out.print(" Enter the name of the product : "); String name = sc.next(); System.out.print(" Enter the price of the product : "); String price = sc.nextFloat(); // create the new product products.add(new Product(name, id, price)); // add the stock to the arraylist quantity.add(addedStockValue); } void removeStock(int id, int stockToRemove) { // find the exact product int index; for(Product i : products) { if(i.getId() == id) { // then find the index of that id index = products.indexOf(i); int value = quantity.get(index); //get the current value of the stock // update the new stock to that product value = value - stockToRemove; if(value MILESTONE 2 Overview In this milestone, you will be defining and coding the beginnings of the StoreView and Shopping Cart classes. You will likely have to refactor some of your code from Milestone 1 and make additions to the Store Manager class. Keep in mind that most of the tasks you will be required to do are relatively open-ended. You must justify any decisions you made that were not obvious in your Change Log. Refer to the examples in Milestone 1. The Shopping Cart Class The Shopping Cart class is very similar to the Inventory class in purpose. A Shopping Cart will keep track of the state of the user's shopping cart. It should maintain the contents that the user adds to it. Remember, the user can also remove items. Shopping Carts are maintained for every user by the Store Manager class as previously mentioned. The StoreView Class The Store View class will manage the GUI for your system. For this milestone, it will be textually displayed in the console. Note that the StoreView class must contain the main() method (i.e. the entry point of the program). Each instance of the StoreView class contains a Store Manager and a unique cartiD used to identify the user of the system. In our case, each Store View instance will have a unique cartID. You can choose how the StoreView class will obtain the Store Manager and cartID (you can pass them via a constructor if you want). However, the cartID should be generated by the Store Manager class. An example of how the StoreView textual User Interface (UI) can look is shown in the figure below. CHOOSE YOUR STOREVIEW >>> O CART >>> 0 Enter a command... browse I- -THE COURSE STORE- --BROWSE- Type 'help' for a list of commands. Stock Product Name Unit Price 76 | SYSC2004 $100.0 0 | SYSC4906 $55.0 32 | SYSC2006 $45.0 3 | MUSI1001 $35.0 12 CRCJ1000 $0.01 132 | ELEC4705 $25.0 322 | SYSC4907 $145.0 GO TO ANOTHER STOREVIEW? (y) >>> n CART >>> 0 Enter a command... addtocart -THE COURSE STORE --ADD- Stock Product Name Unit Price Option 76 | SYSC2004 | $100.0 () 0 | SYSC 4906 $55.0 (1) 32 | SYSC2006 | $45.0 (2) 3 | MUSI1001 $35.0 (3) 12 CRCJ1000 $0.01 (4) 132 | ELEC4705 $25.0 (5) 322 | SYSC4907 $145.0 (6) Option: In the example, the user types a command to enter a certain subroutine. Selections can then be made by using the number displayed adjacent the option on the page. This is just an idea for how you can implement your Ul. In the end, you will be creating a GUI anyway. Remember to document how a user should navigate your system. This should be a combination of a good explanation in your Change Log, and helpful command line prompts. You should think of each instance of the Store View class as a separate user browsing the store. Like multiple users on the internet browsing an online store. However, your store is obviously much simpler. Some code is supplied in the Appendix of this document that might be helpful for simulating multiple users connected to the store. Updates to the Store Manager Class The Store Manager needs some new functionality. Now, it will not only be managing the Inventory, but it will also be managing user Shopping Carts. Each user that connects to the store (new StoreView instance) should have their own unique Shopping Cart. If a user adds something to their cart, the Product's stock in the store Inventory should be decreased accordingly. A user can also remove items from their cart. Note that if the user removes a product from the shopping cart, the inventory must also be updated accordingly. Upon request, the Store Manager should return a new, unique cartID. This means Store Manager should be keeping track of cartIDs in some way. It could be as simple as having a counter that increments every time a new Shopping Cart is made. This implementation is ultimately up to you. Just be sure to document what you do! A user needs to be able to checkout once they are ready (your method for processing a transaction from Milestone 1 will likely need to be changed, or completely removed...). This method should return the total and summary of the items in the cart (print it for the user to see). You can choose to disconnect the user at this point or reset the ort- up to you! If the user quits before checking out, any items in the cart should be returned to the Inventory stock. Note: quitting means the user entered 'quit', not your program suddenly closes; you do not need to worry about that. Now that you will be implementing the UI for the store, you need some way to get the information needed to drive this Ul. Store Manager should have some methods that return needed information about Shopping Carts, or available Products. The Store View class will be using this information to populate the UI for the user. Remember, all communication by the Store View class must be done with the Store Manager only! Questions 1. What kind of relationship is the StoreView and Store Manager relationship? Explain. 2. Due to their behavioral similarity, would make sense to have Shopping Cart extend Inventory, or the other way around? Why or why not? 3. What are some reasons you can think of for why composition might be preferable over inheritance? These do not have to be Java-specific. 4. What are some reasons you can think of for why inheritance might be preferable over composition? These do not have to be Java-specific. Milestone 2 Deliverables 1. The following classes completed according to Milestone 2 specifications: StoreView.java, Shopping Cart.java, Inventory.java, Store Manager.java, and. Do not jump ahead! APPENDIX public static void main(String[] args) { StoreManager sm = new StoreManager(); StoreView svl = new StoreView(sm, sm.assignNewCartID()); StoreView sv2 = new Storeview (sm, sm.assignNewCartID()); StoreView sy3 = new StoreView(sm, sm.assignNewCartID()); StoreView [] users = {svl, sv2, sv3); int activeSV = users.length; Scanner sc = new Scanner (System.in); while (activeSV > 0) { System.out.print("CHOOSE YOUR STOREVIEW >>> "); int choice = sc.nextInt(); if (choice = 0) { if (users (choice] != null) { String chooseAnother = ""; while (!chooseAnother.equals("/") && !chooseAnother.equals("Y")) { // this implementation of displayGUI waits for input and displays the page // corresponding to the user's input, it does this once, and then returns I true if the user entered 'checkout' or 'quit'. if (users (choice].displayGUI()) { users [choice] = null; activeSV--; break; } System.out.print ("GO TO ANOTHER STOREVIEW? (y) >>> "); chooseAnother = sc.next(); } } else { System.out.println("MAIN > ERROR > BAD CHOICE THAT STOREVIEW WAS DEACTIVATED"); } } else { System.out.println( String.format("MAIN > ERROR > BAD CHOICE PLEASE CHOOSE IN RANGE ($d, $d]", 0, users.length - 1) } System.out.println("ALL STOREVIEWS DEACTIVATED"); } MILESTONE 1 Overview In this milestone, you will develop the UML Class diagram of your software using the information provided in this overview. You will also be coding the beginnings of the Store Manager, Inventory and Product classes. You can find the specific requirements for each class below. Keep in mind that most of the tasks you will be required to do are relatively open-ended. You must justify in your Change Log any decisions you made that were not obvious. For example, you should give a brief reason why you used an Array instead of an ArrayList for a class field. However, you should not explain why you gave studentName a type of String. Another example: you do not need to explain why you made a class method public, or a class field private, because doing this is usually normal. But, if for some reason, you made a class attribute protected, you need to explain why. The Product Class The Product class will store information about items being sold by the store. A Product object must only have a (1) name, (2) id, and (3) price. It does not have any other fields. The information in these fields should be retrievable but cannot be changed once the Product object is created. The Inventory Class The Inventory class will track the state of the inventory of your system. It should keep track of the type and quantity of each Product, as well as provide methods to access and modify this information. The following functionalities should be available in any given Inventory object: Get the amount of stock for a given Product ID (Note: it is possible the Product does not exist in the Inventory!). Add a specified amount of stock for a given Product to the inventory (Note: new Products can be added!) Remove a specified amount of stock for a given Product ID from the inventory (Note: you cannot have negative stock, and you cannot delete Products from the Inventory; if a Product's stock reaches 0, leave it.). Get information on a Product given a Product ID. Initialize the Inventory; set the contents of the Inventory to some default values upon object creation. The Store Manager Class Store Manager is the "brain" of the system. It contains all the functionality for managing the Inventory, Shopping Carts, and providing information to the StoreView class. Store Manager manages a single Inventory and it will have a variety of methods - two for now- to interact with this Inventory object. A Store Manager object should: Create a new Inventory object upon object creation (i.e., when a Store Manager object is created). Have functionality to check how much stock of a given Product is in the Inventory. Have functionality to process a transaction given an Array of Product information. For example, imagine that the content of a user's shopping cart is as follows: "[[productID1, quantity), [productiD2, quantity], [product/D3, quantity]]. Given each productID, your method should: Check that the desired quantity exists in the Inventory and return the total for all of the Products. If there is insufficient quantity of any of the products, your method should return some indication of this failure. It could be, for example -1. Remember to subtract the quantities from the Inventory stock if the transaction is successful. O Project Description This course project will allow you to get practice developing a complete Java application. You will work to develop a prototype for a virtual store interface in Java only - it will not be web-based. In the store, you will be able to sell any product of your choice, but it must be specialized. That means, you need to choose a product and focus on selling varieties of that product, for example, varieties of cars, computers, plants, or any other product you would like. The application will provide an interface for a user to shop for products from you store, which, as we already mention is specialized in something you selected. The user should be able to: (1) browse the products, (2) add products to a virtual cart, and eventually, (3) checkout. At first, the interaction will be textual, i.e., though the console. But later, a GUI will be added to complete the experience. The system will be implemented in Java and will have the following classes: (1) Store Manager, (2) StoreView, (3) Inventory, (4) Shopping Cart, (5) Product, and (6) several other classes of your choosing (these will be the classes modelling the products you sell). A brief overview of these classes is presented below. More detailed requirements will be outlined in the relevant milestones. Store Manager: This class will be the controller of the system. Store Manager will (1) manage an Inventory, (2) maintain Shopping Carts for users and (3) respond to queries/prompts/inputs received from the user via the Store View class. StoreView: This class will be the starting point of your application. Ideally, Store Manager and Store View would be able to exist independently. However, communication in this project will be done entirely between objects (no web, sockets, multi-threading, etc.). Therefore, the StoreView will be responsible for initializing the entire system. StoreView will allow the user to interact with the store via an interface. This interface will be textual at first (through the console), and later refactored as a GUI. Inventory: This will be the class responsible for keeping track and maintaining information relating to the products available in the store. The Inventory will be managed by the Store Manager. Shopping Cart: A Shopping Cart will be maintained for any user using the store interface (likely just one; but that could be subject to change!). A user will be able to view the contents of their cart and modify them appropriately. Interactions between the user and their cart will be moderated via the Store Manager. That means, the Store View class cannot actually see any Shopping Cart. The Store Manager will create and manage Shopping Cart objects for users as requested by the StoreView class. MILESTONE 2 Overview In this milestone, you will be defining and coding the beginnings of the StoreView and Shopping Cart classes. You will likely have to refactor some of your code from Milestone 1 and make additions to the Store Manager class. Keep in mind that most of the tasks you will be required to do are relatively open-ended. You must justify any decisions you made that were not obvious in your Change Log. Refer to the examples in Milestone 1. The Shopping Cart Class The Shopping Cart class is very similar to the Inventory class in purpose. A Shopping Cart will keep track of the state of the user's shopping cart. It should maintain the contents that the user adds to it. Remember, the user can also remove items. Shopping Carts are maintained for every user by the Store Manager class as previously mentioned. The StoreView Class The Store View class will manage the GUI for your system. For this milestone, it will be textually displayed in the console. Note that the StoreView class must contain the main() method (i.e. the entry point of the program). Each instance of the StoreView class contains a Store Manager and a unique cartiD used to identify the user of the system. In our case, each Store View instance will have a unique cartID. You can choose how the StoreView class will obtain the Store Manager and cartID (you can pass them via a constructor if you want). However, the cartID should be generated by the Store Manager class. An example of how the StoreView textual User Interface (UI) can look is shown in the figure below. CHOOSE YOUR STOREVIEW >>> O CART >>> 0 Enter a command... browse I- -THE COURSE STORE- --BROWSE- Type 'help' for a list of commands. Stock Product Name Unit Price 76 | SYSC2004 $100.0 0 | SYSC4906 $55.0 32 | SYSC2006 $45.0 3 | MUSI1001 $35.0 12 CRCJ1000 $0.01 132 | ELEC4705 $25.0 322 | SYSC4907 $145.0 GO TO ANOTHER STOREVIEW? (y) >>> n CART >>> 0 Enter a command... addtocart -THE COURSE STORE --ADD- Stock Product Name Unit Price Option 76 | SYSC2004 | $100.0 () 0 | SYSC 4906 $55.0 (1) 32 | SYSC2006 | $45.0 (2) 3 | MUSI1001 $35.0 (3) 12 CRCJ1000 $0.01 (4) 132 | ELEC4705 $25.0 (5) 322 | SYSC4907 $145.0 (6) Option: In the example, the user types a command to enter a certain subroutine. Selections can then be made by using the number displayed adjacent the option on the page. This is just an idea for how you can implement your Ul. In the end, you will be creating a GUI anyway. Remember to document how a user should navigate your system. This should be a combination of a good explanation in your Change Log, and helpful command line prompts. You should think of each instance of the Store View class as a separate user browsing the store. Like multiple users on the internet browsing an online store. However, your store is obviously much simpler. Some code is supplied in the Appendix of this document that might be helpful for simulating multiple users connected to the store. Updates to the Store Manager Class The Store Manager needs some new functionality. Now, it will not only be managing the Inventory, but it will also be managing user Shopping Carts. Each user that connects to the store (new StoreView instance) should have their own unique Shopping Cart. If a user adds something to their cart, the Product's stock in the store Inventory should be decreased accordingly. A user can also remove items from their cart. Note that if the user removes a product from the shopping cart, the inventory must also be updated accordingly. Upon request, the Store Manager should return a new, unique cartID. This means Store Manager should be keeping track of cartIDs in some way. It could be as simple as having a counter that increments every time a new Shopping Cart is made. This implementation is ultimately up to you. Just be sure to document what you do! A user needs to be able to checkout once they are ready (your method for processing a transaction from Milestone 1 will likely need to be changed, or completely removed...). This method should return the total and summary of the items in the cart (print it for the user to see). You can choose to disconnect the user at this point or reset the ort- up to you! If the user quits before checking out, any items in the cart should be returned to the Inventory stock. Note: quitting means the user entered 'quit', not your program suddenly closes; you do not need to worry about that. Now that you will be implementing the UI for the store, you need some way to get the information needed to drive this Ul. Store Manager should have some methods that return needed information about Shopping Carts, or available Products. The Store View class will be using this information to populate the UI for the user. Remember, all communication by the Store View class must be done with the Store Manager only! Questions 1. What kind of relationship is the StoreView and Store Manager relationship? Explain. 2. Due to their behavioral similarity, would make sense to have Shopping Cart extend Inventory, or the other way around? Why or why not? 3. What are some reasons you can think of for why composition might be preferable over inheritance? These do not have to be Java-specific. 4. What are some reasons you can think of for why inheritance might be preferable over composition? These do not have to be Java-specific. Milestone 2 Deliverables 1. The following classes completed according to Milestone 2 specifications: StoreView.java, Shopping Cart.java, Inventory.java, Store Manager.java, and. Do not jump ahead! APPENDIX public static void main(String[] args) { StoreManager sm = new StoreManager(); StoreView svl = new StoreView(sm, sm.assignNewCartID()); StoreView sv2 = new Storeview (sm, sm.assignNewCartID()); StoreView sy3 = new StoreView(sm, sm.assignNewCartID()); StoreView [] users = {svl, sv2, sv3); int activeSV = users.length; Scanner sc = new Scanner (System.in); while (activeSV > 0) { System.out.print("CHOOSE YOUR STOREVIEW >>> "); int choice = sc.nextInt(); if (choice = 0) { if (users (choice] != null) { String chooseAnother = ""; while (!chooseAnother.equals("/") && !chooseAnother.equals("Y")) { // this implementation of displayGUI waits for input and displays the page // corresponding to the user's input, it does this once, and then returns I true if the user entered 'checkout' or 'quit'. if (users (choice].displayGUI()) { users [choice] = null; activeSV--; break; } System.out.print ("GO TO ANOTHER STOREVIEW? (y) >>> "); chooseAnother = sc.next(); } } else { System.out.println("MAIN > ERROR > BAD CHOICE THAT STOREVIEW WAS DEACTIVATED"); } } else { System.out.println( String.format("MAIN > ERROR > BAD CHOICE PLEASE CHOOSE IN RANGE ($d, $d]", 0, users.length - 1) } System.out.println("ALL STOREVIEWS DEACTIVATED"); } MILESTONE 1 Overview In this milestone, you will develop the UML Class diagram of your software using the information provided in this overview. You will also be coding the beginnings of the Store Manager, Inventory and Product classes. You can find the specific requirements for each class below. Keep in mind that most of the tasks you will be required to do are relatively open-ended. You must justify in your Change Log any decisions you made that were not obvious. For example, you should give a brief reason why you used an Array instead of an ArrayList for a class field. However, you should not explain why you gave studentName a type of String. Another example: you do not need to explain why you made a class method public, or a class field private, because doing this is usually normal. But, if for some reason, you made a class attribute protected, you need to explain why. The Product Class The Product class will store information about items being sold by the store. A Product object must only have a (1) name, (2) id, and (3) price. It does not have any other fields. The information in these fields should be retrievable but cannot be changed once the Product object is created. The Inventory Class The Inventory class will track the state of the inventory of your system. It should keep track of the type and quantity of each Product, as well as provide methods to access and modify this information. The following functionalities should be available in any given Inventory object: Get the amount of stock for a given Product ID (Note: it is possible the Product does not exist in the Inventory!). Add a specified amount of stock for a given Product to the inventory (Note: new Products can be added!) Remove a specified amount of stock for a given Product ID from the inventory (Note: you cannot have negative stock, and you cannot delete Products from the Inventory; if a Product's stock reaches 0, leave it.). Get information on a Product given a Product ID. Initialize the Inventory; set the contents of the Inventory to some default values upon object creation. The Store Manager Class Store Manager is the "brain" of the system. It contains all the functionality for managing the Inventory, Shopping Carts, and providing information to the StoreView class. Store Manager manages a single Inventory and it will have a variety of methods - two for now- to interact with this Inventory object. A Store Manager object should: Create a new Inventory object upon object creation (i.e., when a Store Manager object is created). Have functionality to check how much stock of a given Product is in the Inventory. Have functionality to process a transaction given an Array of Product information. For example, imagine that the content of a user's shopping cart is as follows: "[[productID1, quantity), [productiD2, quantity], [product/D3, quantity]]. Given each productID, your method should: Check that the desired quantity exists in the Inventory and return the total for all of the Products. If there is insufficient quantity of any of the products, your method should return some indication of this failure. It could be, for example -1. Remember to subtract the quantities from the Inventory stock if the transaction is successful. O Project Description This course project will allow you to get practice developing a complete Java application. You will work to develop a prototype for a virtual store interface in Java only - it will not be web-based. In the store, you will be able to sell any product of your choice, but it must be specialized. That means, you need to choose a product and focus on selling varieties of that product, for example, varieties of cars, computers, plants, or any other product you would like. The application will provide an interface for a user to shop for products from you store, which, as we already mention is specialized in something you selected. The user should be able to: (1) browse the products, (2) add products to a virtual cart, and eventually, (3) checkout. At first, the interaction will be textual, i.e., though the console. But later, a GUI will be added to complete the experience. The system will be implemented in Java and will have the following classes: (1) Store Manager, (2) StoreView, (3) Inventory, (4) Shopping Cart, (5) Product, and (6) several other classes of your choosing (these will be the classes modelling the products you sell). A brief overview of these classes is presented below. More detailed requirements will be outlined in the relevant milestones. Store Manager: This class will be the controller of the system. Store Manager will (1) manage an Inventory, (2) maintain Shopping Carts for users and (3) respond to queries/prompts/inputs received from the user via the Store View class. StoreView: This class will be the starting point of your application. Ideally, Store Manager and Store View would be able to exist independently. However, communication in this project will be done entirely between objects (no web, sockets, multi-threading, etc.). Therefore, the StoreView will be responsible for initializing the entire system. StoreView will allow the user to interact with the store via an interface. This interface will be textual at first (through the console), and later refactored as a GUI. Inventory: This will be the class responsible for keeping track and maintaining information relating to the products available in the store. The Inventory will be managed by the Store Manager. Shopping Cart: A Shopping Cart will be maintained for any user using the store interface (likely just one; but that could be subject to change!). A user will be able to view the contents of their cart and modify them appropriately. Interactions between the user and their cart will be moderated via the Store Manager. That means, the Store View class cannot actually see any Shopping Cart. The Store Manager will create and manage Shopping Cart objects for users as requested by the StoreView class
Step by Step Solution
There are 3 Steps involved in it
Step: 1
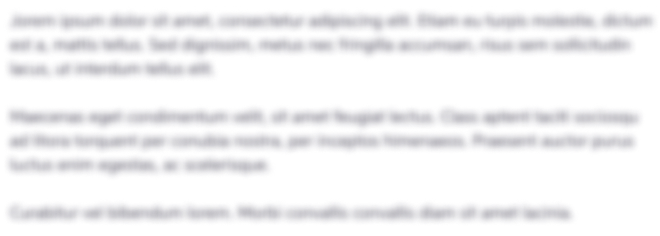
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started