Question
Please Help writing the following Nim game in C language. Part A) For this first part of the lab write a program to play the
Please Help writing the following Nim game in C language.
Part A)
For this first part of the lab write a program to play the "nim" game.
As a reminder, the rules are these:
Start with three heaps of stones containing 5, 4, and 3 stones.
Players alternate turns.
On their turn a player may take as many stones as they like from any one bowl, but must take
at least one stone.
The player to take the last stone(s) from the last non-empty heap wins.
Here is a the nim program with some added comments:
#include
#include
int system(const char *string);
int main(void)
{
int heapCount1, heapCount2, heapCount3;
int heap, player, take,i;
int *heapCount_p;
/* initialize heap counts */
heapCount1 = 3;
heapCount2 = 4;
heapCount3 = 5;
player = 2; // first player will be player 1 (see below)
/* while any stones remain, */
while (heapCount1 + heapCount2 + heapCount3 > 0)
{
/* select the next player */
player = 3 - player; // player 1 -> 2 and 2 -> 1
printf("player %d's turn ", player);
/* print out the counts */
printf("(1) ");for (i=0;i printf(" "); printf("(2) ");for (i=0;i printf(" "); printf("(3) ");for (i=0;i printf(" "); /* let the player select a heap that contains at least one stone */ for (;;) { /* let the player select a heap */ for (;;) { printf("heap to take stones from (1 - 3): "); scanf("%d", &heap); if (1 <= heap && heap <= 3) { break; } printf("illegal heap value -- try again "); 3/29/2018 Lab 9 - Pointers } /* * At this point, we know that `heap` is valid, so we * check to make sure it's not empty. */ /* make `heapCount_p` point at the relevant heap */ switch (heap) { case 1: heapCount_p = &heapCount1; break; case 2: heapCount_p = &heapCount2; break; case 3: heapCount_p = &heapCount3; break; } /* * If there are stones in it, the move is legal, so break * out of the loop. */ if ((*heapCount_p) > 0) { break; } printf("heap %d contains no stones -- try again ", heap); } /* let the player select a "take" -- a count of stones to remove */ for (;;) { printf("count of stones to take from heap %d (1 - %d): ", heap, (*heapCount_p)); scanf("%d", &take); /* * If the take is in the proper range, the move is legal, * so break out of the loop. */ if (1 <= take && take <= (*heapCount_p)) { break; } printf("illegal heap count -- try again "); } /* deduct those stones */ (*heapCount_p) -= take; printf(" "); // blank line for cosmetic purposes system("cls"); } /* declare the last player the winner */ printf("player %d wins ", player); return 0; } Part B) The objective of this part is for you to rewrite (or "refactor") the code we developed in class to improve its readability by decomposing it into five separate, well-defined functions: printStatus() prints out the player (number) whose turn it is and the current contents of all heaps. setHeapCountPointer() returns a pointer to the appropriate heap count, given the heap number. getHeap() prompts the user for a (legal) heap number. getTake() prompts the user for a (legal) number of stones to take from a given heap. playNim() plays the game and returns the winner. (We have already refactored this in the template below.) The first four functions are independent of each other, but playNim() will call the others. Do not implement the same code in more than one place. (i.e. "DRY -- Don't Repeat Yourself") The listing marks off the sections of code that belong to each function. Replace each section with a call to that function. When you turn that code into a function and design its prototype, look at the block of code and answer these questions: What are the inputs? What are the outputs? (And some outputs may also be inputs.) What kind of function is this? (Remember the table from class.) Constraints: Don't pass any arguments to a function that aren't inputs or outputs. If the function only has a single output (only) value, use a return. Otherwise, use pointer arguments. Don't use a pointer argument if a pointer argument isn't required. Here is a template to put all of these functions in. As a demonstration, we have already done this for playNim(). You are not permitted to modify main(): #include /* * ASSIGNMENT * * Cut, paste, and (slightly) modify `printStatus()` here. */ 3/29/2018 Lab 9 - Pointers /* * ASSIGNMENT * * Cut, paste, and (slightly) modify `getHeap()` here. */ /* * ASSIGNMENT * * Cut, paste, and (slightly) modify `getHeapCountPointer()` here. */ /* * ASSIGNMENT * * Cut, paste, and (slightly) modify `getTake()` here. */ /* * Here is the (already-refactored) `playNim()` function as an * example. */ int playNim(void) { int heapCount1, heapCount2, heapCount3; int heap, player, take; int *heapCount_p; /* initialize heap counts */ heapCount1 = 3; heapCount2 = 4; heapCount3 = 5; player = 2; // first player will be player 1 (see below) /* while any stones remain, */ while (heapCount1 + heapCount2 + heapCount3 > 0) { /* select the next player */ player = 3 - player; // player 1 -> 2 and 2 -> 1 // ---- beginning of `printStatus()` code // (replace with `printStatus()` call) printf("player %d's turn ", player); /* print out the counts */ printf("heap 1: %d ", heapCount1); printf("heap 2: %d ", heapCount2); printf("heap 3: %d ", heapCount3); // ---- end of `printStatus()` code /* let the player select a heap that contains at least one stone */ for (;;) 3/29/2018 Lab 9 - Pointers { /* let the player select a heap */ // ---- beginning of `getHeap()` code // (replace with `getHeap()` call) for (;;) { printf(" heap to take stones from (1 - 3): "); scanf("%d", &heap); if (1 <= heap && heap <= 3) { break; } printf("no such heap -- try again "); } // ---- end of `getHeap()` code /* * At this point, we know that `heap` is valid, so we * check to make sure it's not empty. */ // ---- beginning of `getHeapCountPointer()` code // (replace with `getHeapCountPointer()` call) /* make heapCount_p point at the relevant heap */ switch (heap) { case 1: heapCount_p = &heapCount1; break; case 2: heapCount_p = &heapCount2; break; case 3: heapCount_p = &heapCount3; break; } // ---- end of `getHeapCountPointer()` code if ((*heapCount_p) > 0) { break; } printf("heap %d contains no stones -- try again ", heap); } /* let the player select a "take" -- a count of stones to remove */ // ---- beginning of `getTake()` code // (replace with `getTake()` call) for (;;) { printf("count of stones to take from heap %d (1 - %d): ", heap, (*heapCount_p)); scanf("%d", &take); /* * If the take is in the proper range, the move is legal, * so break out of the loop. */ 3/29/2018 Lab 9 - Pointers if (1 <= take && take <= (*heapCount_p)) { break; } printf("illegal stone count -- try again "); } // ---- end of `getTake()` code /* deduct those stones */ (*heapCount_p) -= take; printf(" "); // blank line for cosmetic purposes } return player; } int main(void) { int winner; winner = playNim(); printf("player %d wins ", winner); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
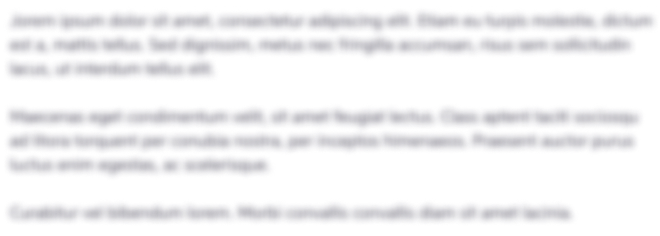
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started