Question
Please implement queue.h and queue.cpp with throw/catch/try exceptions and test with queuetest.cpp Queue.h using namespace std; #include //----- Globally setting up the aliases ---------------- typedef
Please implement queue.h and queue.cpp with throw/catch/try exceptions and test with queuetest.cpp
Queue.h
using namespace std; #include
//----- Globally setting up the aliases ----------------
typedef string el_t; // el_t is an alias for the data type // el_t is unknown to the client
const int MAX_SIZE = **; // this is the max number of elements // MAX is unknown to the client //-------------------------------------------------------
class queue { private: // Data members are: el_t el[MAX_SIZE]; // an array called el. // Elements will be found between front and rear but // front may be behind rear since the queue wraps around int count; // how many elements do we have right now? int front; // where the front element of the queue is. int rear; // where the rear element of the queue is.
public: // prototypes to be used by the client // Note that no parameter variables are given
//add exception handling classes here with comments ** **
queue(); // constructor to create an object ~queue(); //destructor to destroy an object
// PURPOSE: returns true if queue is empty, otherwise false bool isEmpty();
// PURPOSE: returns true if queue is full, otherwise false bool isFull();
// PURPOSE: if full, throws an exception Overflow // if not full, enters an element at the rear // PRAMETER: provide the element to be added void add(el_t);
// PURPOSE: if empty, throws an exception Underflow // if not empty, removes the front element to give it back // PRAMETER: provide a holder for the element removed (pass by ref) void remove(el_t&);
// PURPOSE: if empty, throws an exception Underflow // if not empty, give back the front element (but does not remove it) // PARAMETER: provide a holder for the element (pass by ref) void frontElem(el_t&);
// PURPOSE: returns the current size to make it // accessible to the client caller int getSize();
// PURPOSE: display everything in the queue from front to rear // enclosed in [] // if empty, displays [ empty ] void displayAll();
// PURPOSE: if empty, throws an exception Underflow //if queue has just 1 element, does nothing //if queue has more than 1 element, moves the front one to the rear void goToBack();
};
Queue.cpp
using namespace std; #include
// constructor queue::queue() { }
//destructor queue::~queue() { // nothing }
// PURPOSE: returns true if queue is empty, otherwise false bool queue::isEmpty() { }
// PURPOSE: returns true if queue is full, otherwise false bool isFull() {}
// PURPOSE: if full, throws an exception Overflow // if not full, enters an element at the rear // PAREMETER: provide the element (newElem) to be added void add(el_t newElem) {}
// PURPOSE: if empty, throw Underflow // if not empty, removes the front element to give it back // PARAMETER: provide a holder (removedElem) for the element removed (pass by ref) void remove(el_t& removedElem) {}
// PURPOSE: if empty, throws an exception Underflow // if not empty, give back the front element (but does not remove it) //PARAMETER: provide a holder (theElem) for the element (pass by ref) void frontElem(el_t& theElem) {}
// PURPOSE: returns the current size to make it // accessible to the client caller int getSize() {}
// PURPOSE: display everything in the queue horizontally // from front to rear enclosed in [ ]
// if empty, displays [ empty ] void displayAll() { if (isEmpty()) { cout << "[ empty ]" << endl;} else { int j = front; cout << "["; for (int i = 1; i <= count; i++) { ** } cout << "]" << endl; } }
// PURPOSE: if empty, throws an exception Underflow //if queue has just 1 element, does nothing //if queue has more than 1 element, moves the front one to the rear by //calling remove followed by add. void goToBack() { // ** comment a local variable }
Main.cpp
using namespace std;
#include
// Purpose of the Program: // This test program tests a string queue class you have developed. // It tests each method using a menu. // It displays the queue whenever it is modified. // How to use: ./a.out after compiling all .cpp files. // Overall Algorithm: switch inside a do-while loop int main () {
cout << "************** Queue Test Program *************" << endl; cout << "This program tests the queue ADT implemented as" << endl; cout << "an array. " << endl; cout << "It is a menu based program allowing you to test each method. " << endl; cout << "It displays the entire queue whenever it is modified." << endl; cout << "***********************************************" << endl;
queue Q; // this is the queue object Q
int selection; // this will indicate what the user wants to do
do { try { cout << endl << "MENU: These are your options: " << endl << endl; cout << " (1) Add an element " << endl; cout << " (2) Display the entire queue " << endl; cout << " (3) Remove an element" << endl; cout << " (4) Display the front element" << endl; cout << " (5) Check to see if is it empty" << endl; cout << " (6) Check to see if is it full" << endl; cout << " (7) Go to Back" << endl; cout << " (8) Get size" << endl; cout << " Enter ( 0 ) to quit " << endl; cout << "===>";
cin >> selection;
string toadd; //this is what the user wants to add to the queue string erased; // this is what is removed from the queue string looked; // this is the element at the front
switch (selection) {
case 1: // the Add choice
cout << "Enter a string to be added : "; cin >> toadd; Q.add(toadd); cout << "The updated contents of the queue are: " << endl; Q.displayAll(); break;
case 2: // the Display choice cout << "The current contents are: " << endl; Q.displayAll(); break;
case 3: // The Pop choice Q.remove(erased); cout << erased << " has been removed." << endl; cout << "The updated contents of the queue are: "; Q.displayAll(); break;
case 4: // the top element case Q.frontElem(looked); cout << looked << " is at the front." << endl; break;
case 5: // the check-empty case if (Q.isEmpty()) cout << "Queue is empty right now." << endl; else cout << "Queue has some elements." << endl; break;
case 6: // the check-full case if (Q.isFull()) cout << "Queue is full right now." << endl; else cout << "Queue has more room." << endl; break;
case 7: // go to back case Q.goToBack(); Q.displayAll(); break;
case 8: // check the size case cout << Q.getSize() << " elements are in Queue." << endl; break;
} // end of switch statement
}// end of try - Now catch exceptions
catch(queue::Overflow) {cerr << "Error: You have caused the queue to overflow." << endl;} catch(queue::Underflow) {cerr << "Error: You have caused the queue to underflow." << endl;}
} while(selection!=0);
cout << "Bye bye - ending the queue test program" << endl;
}// end of main
Step by Step Solution
There are 3 Steps involved in it
Step: 1
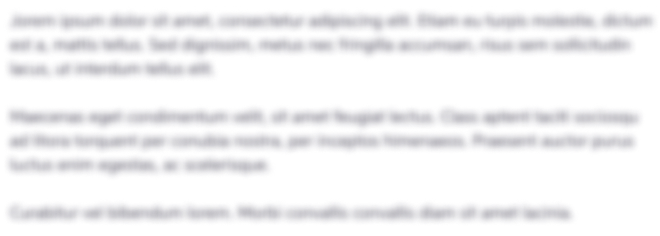
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started