Question
PLease only use , , and Problem 2 (40 points): Word Search We will implement a word search game for this problem. The file DataFile.txt
PLease only use
We will implement a word search game for this problem. The file DataFile.txt contains a word
search puzzle that is size 201 x 100 (201 lines and each line has 100 characters) all characters are
uppercase.
This puzzle is made simple in that all words are hidden horizontally, and in forward order (i.e. no
words are written backwards, nor vertically nor diagonally).
Although the words are all hidden in forward order, they may occur multiple times on any given
line (see sample code execution)
Since there are no lowercase characters in the puzzle, you should not worry about converting
lowercase to uppercase or vice versa.
A sample portion of the puzzle is
(BLANK)
which is difficult enough to search as it is.
The words that are hidden in the puzzle are: PROGRAMMING, FPRINTF, FSCANF, DOUBLE,
MAIN, DOWHILE, FGETS, INT, IF, WHILE, ENGINEERING, RETURN, CHAR, FCLOSE,
ELECTRICAL, ECE, VOID, FLOAT, FOR
Write a C program that will search the text file for the word entered by a user. Your program should
a) read from the text file one line at a time (Hint: use fgets)
b) print to the screen
b1) the word that is currently being searched.
b2) number of times the word was found on the line
b3) the line number and the line where the word was found.
b4) how many times in total the word was found in the puzzle or the word is not found
c) be interactive such that it asks a user whether he/she wants to search the puzzle with a new word.
See sample code execution.
d) use the following given user-defined function:
int search_current_line(char Line_str[], char word[])
//This function searches a test string (Line_str) for a word
//and returns number of times the word is found on Line_str
A command that you might find useful is:
Assuming that fp is declared as FILE *fp;
you can rewind the file pointer back to the beginning of the file using
rewind(fp);
3
A function that you might find useful is:
void my_strcpy(char In[], char Out[], int L){
int i;
for(i = 0; i
Out[i] = In[i];
Out[L] = '\0';
return;
}
Sample Code Executions (Red entered by a user)
Enter a word you want to search for: MAIN
Found MAIN 3 times on line 82
The line is:
MAINBZFHNJLYOXGNGSSGNVEXJITUTSMAINZHJRJMCZKBUITWQKFZVDBPDITNNKOGQZHDTC
LTMAINEYUWPYKJCQSHKGYSNAZJFYJ
Found MAIN 1 times on line 94
The line is:
ZFDVDNEEPTFWGIAZYKZHPKZWZMSXDMAINLYNZEZFQOQJWASFVUQAKQQMSMULJJHXKZLG
VWXOEELYDSOSCHKONUACTCXAPTDMNKZ
The string: "MAIN" was found a total of 4 times in the puzzle.
Do you want to search another word (q to quit)? y
Enter a word you want to search for: TEST
The string: "TEST" was NOT found in the puzzle.
Do you want to search another word (q to quit)? y
Enter a word you want to search for: PROGRAMMING
Found PROGRAMMING 1 times on line 43
The line is:
ABOZMAKPCFBGZZXPROGRAMMINGUOURMWESVJJGDHRTNRAPOODNDSZTBZZSAVRQPWWA
MRRUBUXPGPJSWIUVHLSHTOULWEVTVZHHB
This function copies one string to another. However, it can
copy a portion of a string. For example, if you were
working with a findstring of length 7, and a string named
str that contains
012345 Here is an example string
and you want to compare only the 5th through the 11th
elements to the findstring, then you would call
my_strcpy(&str[4], outstr, 7);
Then outstr contains
45 Here
and is of length 7. Now outstr is ready to be compared to
the find string using strcmp which will compare2 strings
of the same length.
4
Found PROGRAMMING 2 times on line 54
The line is:
DPUIHKVUOVKWNORNQPROGRAMMINGKODKWFFPREYOUCNXXOPDNNUPLFQDQXMCRVRNW
PIYGQJYRJLECDDVAKOPKMMTPROGRAMMING
The string: "PROGRAMMING" was found a total of 3 times in the puzzle.
Do you want to search another word (q to quit)? y
Enter a word you want to search for: IF
Found IF 1 times on line 3
The line is:
ZYJCTQVIHSZICSMMODWDNXIVKLKGPJOVGKHJUPZEDAIQXJQVYBQCXRBHIFIXCHDLPCCRAG
NESNRYYACYNPIIBMRNWSWAIZIPLUF
Found IF 1 times on line 11
The line is:
OVKWNCVGIIIXGMTGUIUOLIWNZNTGBQSVNEHSLJERSHVNZXYAAXECOEPOZDVBZPCFJMBLZK
FBLLKHDIFBTDUACNPQRPKCNDYDLSY
Found IF 1 times on line 17
The line is:
GLTSKOQUYNEXKSSCJRPGDSXCEGYYEUIFXQCLYAOKJONYUGBHQISHPNBABBMHZOWPDXZLB
YXFLYJERRZRDCESTNGTAMTVFSRNHDF
Found IF 1 times on line 25
The line is:
TDSVTHAKDYNDUHCKKJILABGWANOUZGESMMNBZZMJRNZMXOISMUXBSORSXQZYYYCBIFTO
TYFBNHZZJMJPLVYTQEIUQUJUUGHXOAS
Found IF 1 times on line 30
The line is:
AYLXNIYFSJBGUJDMBJCSTOYMXONTOJWIFDAENCTISVMTSJWMGOCBCMEDPNZHPTNOPPEPDN
RAYIYISNTAUIHJGSRIHGTYGMMKZRC
Found IF 1 times on line 33
The line is:
PQWXZYHSCAMYHWGGEIUOFMUZOBUZUCXUPEGSBKVXJFYUTPZLZEOQTWFFWGOYFCVTRLX
MLZPSWVKJNLHDEZENXHPKQYJFFILZVIF
Found IF 1 times on line 36
The line is:
WWWIMIXYFZQYEIUJBTRUWBJUUAKSWEAOAMFNZAWMZXMECVDEDQRYLSTFPAHHGPVJZWD
ZMDHYJQHVWYORSDWCXXVTXHIFHONMMNI
Found IF 1 times on line 45
The line is:
OWCXBEPGSOBWSDZJPROBETOWHWOAPXWYHTSYMOUPCXWBZKYJAVLKYGSTFBEPLEGNIFR
HVKKKABMLGLQYRXPGOPRZEBCQZWNNYGI
5
Found IF 1 times on line 55
The line is:
ZFGKREOHTHCVYTKZVTDYHAYMFHRPEXTIFYNRDEXAUEZXGYWMMGQZXJHJUTBSMBQZJUFT
FMVHWJMLNXCWVMJDVHXEEAUZDIHVIDI
Found IF 1 times on line 61
The line is:
IATJUBGBRDPKVERWEXQYZZGXTWPIFOTGALFGNFNPCUMUZYUPDONCZHLTPVRFXODURIGRR
RUHAAVNSIKXZPCJGHZZCKUQVRQDMBM
Found IF 1 times on line 62
The line is:
LONWTGLUFWAMKKKBGSVZPPTNPYYQBPNOLVNZYQVGMVMJJITHGFTDHJUONBUTAEGLXBQ
NYBVBPARZVBYEVCIFWGDOQXCOIAEHMJE
Found IF 1 times on line 70
The line is:
CWSGURXTDEWNSKREFWOJPTZTLZPSWKYCCVXPTGQKRFHDPURVEQQVQGNIFRLRQSIIIODWT
KVJPRFZZUQHOHUQWLPIRYDPLATPJMM
Found IF 1 times on line 72
The line is:
FVNUQJKVLTIILZUVBGNBRPYTFFSCFDARIRBWLSLLOYDTSNMSQZHEFBKGFINAILLOPFDAABIF
PJXJPSRRCDTBTGWKMVDUDJZGJQJ
Found IF 1 times on line 88
The line is:
XRNUBQNNQVTOAWIFVSHAPXYVKGACPLVBUSLPYSOUZYAISDRTDCGUYPMXIATOMYMFUAY
BMEZXVQBXBJJBCZSEVEITJIDURVCYCKP
Found IF 1 times on line 108
The line is:
FVWULETGDSNJDSVYMSZVYQDGHTITIFGTIKNFFOKKEJFPQRRLMEXKXUHKZSMWLDBGGYAAJ
ABOSUNVYDYYECNYEXNLGXJIPEZHVHF
Found IF 1 times on line 121
The line is:
QVLQTWQUCTZAEHTHMPEHMGHTTWHJYYABTIJWQFEGJMJPVGORSBKIEOBFKIHACOEIJYDIEA
WSVBGZINLIFKTNTGQHKAEZAXKEJJV
Found IF 1 times on line 148
The line is:
MIOUGBGIFFVBKISLUDFLSPPOJPXXFFFCIVHWZISFHCDEUVRHIYFJGGTCACSMANQKFKIVVMVC
ZJOGDSCRFIXUZVNZKMWZXYJIQDP
Found IF 1 times on line 150
The line is:
OYEHWKQJQARTSQHOWCILIYEBLZIFLEYVDREJSWTBEVKLTGFFDZKZPMLPIWWYFJNLDDVUMF
HGIMLUDPEZJNUVFJCPQXOKNETMLNX
6
Found IF 1 times on line 157
The line is:
MEDMBQWTDXHRBCKPQALKHIERBHKXXJOJREJEIPAOPLRUSYHYXRGQAJEIHTPZKCQLDJTZZZ
ASBKTHSZUTQWYRJYTJQGKSGBPZKIF
Found IF 1 times on line 158
The line is:
YVMUGCFIYUEILQFZQFISOUMVZVFSARBIFODVUAZGGTXUFVGZRYIAZNWIHPEUHJUYHAZCUL
QWXNCOHMAJNORUGPNHMWIPQMXEROP
Found IF 1 times on line 189
The line is:
WIFPWFFRACMPIOUGFYZVOKDCIWZSYNRRODFMMOMLGRXJDRKOKHNUSYIUVDFLONTULUA
OFELSEMCOKMHDOPBHTJXKSQYZSQYOAIG
Found IF 1 times on line 191
The line is:
OLOHWEIIWNOBHTODZSILJDGBVCFTUFHTGIWZCXPAZABIYBCDGFMCLOCDHSGWISYOKDFOO
ERRPHNDDURGLIFVORFPXAKKRPEVNHA
Found IF 1 times on line 192
The line is:
KXUCORVXEMOKCLJWBGIFKZNETFVWTBGSTSNWXHTMPRNCEBJRYZJBVUVWPIZYUHMQGLFG
XKBRSSANBXXXRPMRREEKLKODHJYLYCK
Found IF 1 times on line 197
The line is:
VTOVICERQAKZYITRZVQBBGKEYMQETLMMHXDCKFQKQPVAPMMYHKPAZMENRLTWZVVTGU
DYZLIFZHYBYBBSPJTXUJCLCPPGJBFUNXG
Found IF 1 times on line 200
The line is:
MFXYUOUZJKSNJDVNCZLUWBHDONUOAQEDFZRGAKDRKGKQKLWODCEBLMRMWDBUUOVQP
BLPSEQKFLYNVUNZIKOMOSXHCSCHCQMLXIF
The string: "IF" was found a total of 25 times in the puzzle.
Do you want to search another word (q to quit)? y
Enter a word you want to search for: VOID
Found VOID 1 times on line 155
The line is:
ZCGLUHXEKOWJRNVHDNIOKUBVOIDQQAOZCUHYFMLQQRQLZQEEMKKZKRIJBXRGMRQLCXP
ODDWUGFYTFGDKZZAOONSSKVWVOKQIMKG
The string: "VOID" was found a total of 1 times in the puzzle.
Do you want to search another word (q to quit)? q
Step by Step Solution
There are 3 Steps involved in it
Step: 1
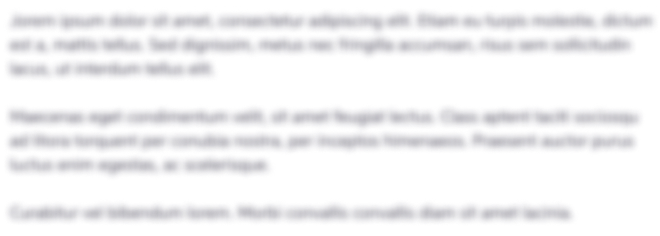
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started