Question
Please provide a step by step with code on how to complete this A4 project using C in Putty. Thank you! A4 builds on A3
Please provide a step by step with code on how to complete this A4 project using C in Putty. Thank you!
A4 builds on A3 so see A3 project description and code below grading.
Objective:
In this assignment, you will be writing a multithreaded program that shares data between threads. You will need to properly protect data that is shared between threads. You will be using mutex locks around critical sections to ensure you program works correctly and does not experience race conditions.
Details:
The previous program could possibly use too many threads and we need to fix that problem. You will rewrite the program from Assignment 3 - Multithreaded Program to only use three threads; the main thread, a producer, and a consumer. The three threads will accomplish the following tasks:
Main Thread
Create the producer and consumer threads
Send each number from the command line to the producer thread via a shared buffer.
This buffer only needs to be shared between the main thread and the producer.
Wait for each thread to complete before terminating the program
Producer Thread
Wait for numbers to be added to the buffer shared with the main thread
Factor each number
Save numbers to a buffer shared between the producer and consumer threads
Consumer Thread
Wait for factors to be added to the buffer shared with the producer thread
Display the factors
This program will use two sets of shared data, in the producer-consumer style. Both sets of data need to be protected using pthread_mutex_t variables. The only thread producing any output to the console should be the consumer thread. All other threads should perform their actions silently.
Output:
The output for this program should be identical to the output of Assignment 3 - Multithreaded Program.
Hints:
You have an example of a producer/consumer threading model (Links to an external site.)Links to an external site..
Here is the example:
#include | |
#include | |
#include | |
#define BUFFER_SIZE (20) | |
int *buffer; | |
int counter; | |
int in; | |
int out; | |
void *producer(void *ptr) { | |
int value = 1; | |
while(1) { | |
while(counter == BUFFER_SIZE) | |
; /* do nothing */ | |
buffer[in] = value++; | |
in = (in + 1) % BUFFER_SIZE; | |
counter++; | |
} | |
} | |
void consumer() { | |
int last = 0; | |
while(last | |
while(counter == 0) | |
; /* do nothing */ | |
last++; | |
if ( last != buffer[out] ) { | |
printf("Error at value %d! I was expecting %d ", buffer[out], last); | |
exit(-1); | |
} | |
out = (out + 1) % BUFFER_SIZE; | |
counter--; | |
} | |
} | |
int main() { | |
pthread_t child; | |
buffer = malloc(sizeof(int) * BUFFER_SIZE); | |
counter = 0; | |
in = 0; | |
out = 0; | |
pthread_create(&child, NULL, producer, NULL); | |
consumer(); | |
return 0; | |
} |
The buffer between the main thread and the producer thread can be a simple integer array
The buffer between the producer thread and the consumer thread might be an array of integer pointers.
Each element in the array of integer pointers will point to an array of integers that contains the original number and the factors of that number.
You could also make the producer/consumer buffer an array of pointers to structures. The possibilities are endless.
Submission:
You should submit two files in a tarball. Those two files are:
Makefile - This should contain the necessary make commands to build your program
assn3.c - This is your main source file
You should not include your binary program. I will be deleting it and rebuilding it myself.
I highly recommend creating and using a git repository.
Grading:
This is the grading for this assignment
Points | Description |
3 | tarball of source code submitted to Canvas |
2 | Properly interprets numeric command line option |
5 | Properly creates two threads. No data is required to be passed as parameters |
5 | Shared data is used between Main Thread and Producer thread |
5 | Shared data is used between Producer Thread and Consumer Thread |
10 | Locks are properly implemented for data shared between Main and Producer threads |
10 | Locks are properly implemented for data shared between Producer and Consumer threads |
A3
Please provide a step by step with code on how to complete this project using C in Putty. Thank you!
Objective:
In this assignment, you will be writing a multithreaded program. You will demonstrate your knowledge of the POSIX Pthreads library and how to create, destroy, and wait for threads. You will also demonstrate how to pass data between parent and child threads.
Details:
You will create a multithreaded program that takes one or more numeric arguments from the command line and displays the prime factors (Links to an external site.)Links to an external site. of each number. Each child thread will be passed a number from the parent thread that should be factored by that thread. Each child thread will return a sorted integer array of prime factors for that number. The parent thread will wait for each thread to terminate. The parent thread will display the original number provided and all factors returned by the child thread to the console.
The child thread must not display any data. Your only output should be provided by the main thread, and only after waiting for the thread to complete.
There are many ways to find the prime factors (Links to an external site.)Links to an external site. of a number. The simplest algorithm is probably trial division (Links to an external site.)Links to an external site.. You are welcome to submit more complicated algorithms, but please do not sacrifice your assignment quality for a fancy factorization algorithm. There is no extra credit for complexity.
Output:
Here is some examples of what is expected:
# ./assn3 Usage:./assn3
# ./assn3 12 12: 2 2 3
# ./assn3 7 12 25 7: 7 12: 2 2 3 25: 5 5
# ./assn3 {32..48} 32: 2 2 2 2 2 33: 3 11 34: 2 17 35: 5 7 36: 2 2 3 3 37: 37 38: 2 19 39: 3 13 40: 2 2 2 5 41: 41 42: 2 3 7 43: 43 44: 2 2 11 45: 3 3 5 46: 2 23 47: 47 48: 2 2 2 2 3
Note: The bracket notation in bash allows for a sequence of numbers, separated by spaces
Submission:
You should submit two files in a tarball. Those two files are:
Makefile - This should contain the necessary make commands to build your program
assn3.c - This is your main source file
You should not include your binary program. I will be deleting it and rebuilding it myself.
I highly recommend creating and using a git repository.
Expert Answer
Anonymous answered this
Was this answer helpful?
0
0
85 answers
#include
#include
#include
#include
// A function to print all prime factors of a given number n
void* primeFactors(void *param)
{
//int *p = (int *)param; // pointer to 'int'
//int n = *(int *)param; // the 'int' being pointed to
int n = atoi(param);
// Print the number of 2s that divide n
while (n%2 == 0)
{
printf("%d ", 2);
n = n/2;
}
// n must be odd at this point. So we can skip
// one element (Note i = i +2)
for (int i = 3; i
{
// While i divides n, print i and divide n
while (n%i == 0)
{
printf("%d ", i);
n = n/i;
}
}
// This condition is to handle the case when n
// is a prime number greater than 2
if (n > 2)
printf ("%d ", n);
//Exit the thread
pthread_exit(0);
}
int main(int argc, char *argv[]) {
//Verify two args were passed in
if(argc
fprintf(stderr, "USAGE: ./prime.out
exit(1);
}
//verify the input is greater then or equal to two
if(atoi(argv[1])
fprintf(stderr, "USAGE: %d must be >= 2 ", atoi(argv[1]));
exit(1);
}
pthread_t tid; //Thread ID
pthread_attr_t attr; //Set of thread attributes
for(int i=1;i
{
//Get the default attributes
pthread_attr_init(&attr);
//Create the thread
printf(" %d: ",atoi(argv[i]));
pthread_create(&tid,&attr,primeFactors,argv[i]);
//Wait for the thread to exit
pthread_join(tid,NULL);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
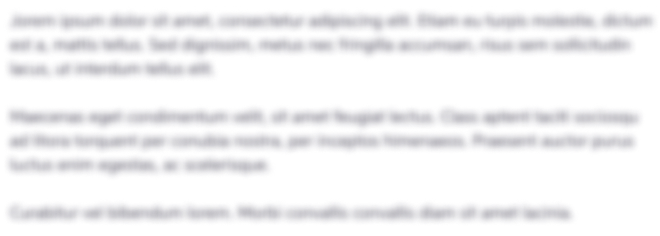
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started