Question
PLEASE READ ALL THE STEPS AND EXPLAIN THEM IN THE PROPER WAY CODE IS GIVEN BELOW IN THE RAW TEXT FORMAT I HAVE ALSO GIVEN
PLEASE READ ALL THE STEPS AND EXPLAIN THEM IN THE PROPER WAY
CODE IS GIVEN BELOW IN THE RAW TEXT FORMAT
I HAVE ALSO GIVEN TH TEXT FILE BELOW
THANKS FOR THE HELP
There are a number of files in this project. Most of them are filled in, but MovieReader has only two empty methods: load, and readLine. Your task is to write code that reads the data in moviesShort.txt into a MovieList. The ReaderTesty.java file contains a simple program that calls the methods in MovieReader and prints out the results. The readLine method has this header public static Movie readLine(String line) It takes one line from the file as a String parameter, and reads each individual field in the parameter into a String or long variable (domestic sales and international sales are long values, not String). It uses all those variables to create Movie object which is returned. There is one exception to this flow: release date. The corresponding instance variable in Movie is a LocalDate. So, you will need to read the value into a String variable, and then convert that String to a LocalDate. You will need a DateTimeFormatter
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd-MM-yyyy"); which you then pass into the LocalDate.parse method to create LocalDate. However, there is one more twist. Some of the release dates in the data file have no value, indicated by the string "NA". This is not uncommon in data files. Your code will need to test for
this when it reads the string. If the string's value is "NA", create LocalDate with the value 0000-01-01. To do , use this line of code releaseDate = LocalDate.of(0,1,1); One more complication: the delimiter that divides the fields in the data file is a pipe ('|'). After you create Scanner, you need to set the delimiter to this pipe character. To do , use this code Scanner lineScanner = new Scanner(line); lineScanner.useDelimiter("\\|"); The header for the load method is public static MovieList load(String filepath) This method uses the file path to create File object and pass it to a Scanner object. This Scanner is a different Scanner from the one that is created in readLine. The load method then reads each line from the file, passing it to readLine. The Movie object returned from readLine is added to the MovieList. When there are no more lines to be read, the method should close the Scanner and return the MovieList Use ReaderTesty to test your program. If you run into problems reading the file contents, either use the debugger to step through the readLine method, or print out each string's value as you read it. That is usually enough to find bugs.
CODE --
movie.java -----
import java.time.LocalDate;
/**
* Represents one movie from a publicdatasetof highest grossing
*Hollywoodmovies. Fields include an id, the movie title, a description
* of the movie, the distributor, the release date, total domestic sales,
* total international sales, a list of keywords representing genres, and
* the r..;@authorBonnieK. MacKellar
*
*/
public class Movie {
private String id;
private String title;
private String info;
private String distributor;
private LocalDate releaseDate;
private long domesticSales;
private long intlSales;
private String genre;
private String rating;
/** create Movie object from the parameters
*
*@paramid
*@paramtitle
*@paraminfo
*@paramdistributor
*@paramreleaseDate
*@paramdomesticSales
*@paramintlSales
*@paramgenre
*@paramrating
*/
public Movie(String id, String title, String info, String distributor, LocalDate releaseDate, long domesticSales,
long intlSales, String genre, String rating) {
this.id = id;
this.title = title;
this.info = info;
this.distributor = distributor;
this.releaseDate = releaseDate;
this.domesticSales = domesticSales;
this.intlSales = intlSales;
this.genre = genre;
this.rating = rating;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getInfo() {
return info;
}
public void setInfo(String info) {
this.info = info;
}
public String getDistributor() {
return distributor;
}
public void setDistributor(String distributor) {
this.distributor = distributor;
}
public LocalDate getReleaseDate() {
return releaseDate;
}
public void setReleaseDate(LocalDate releaseDate) {
this.releaseDate = releaseDate;
}
public long getDomesticSales() {
return domesticSales;
}
public void setDomesticSales(int domesticSales) {
this.domesticSales = domesticSales;
}
public long getIntlSales() {
return intlSales;
}
public void setIntlSales(int intlSales) {
this.intlSales = intlSales;
}
public String getGenre() {
return genre;
}
public void setGenre(String genre) {
this.genre = genre;
}
public String getRating() {
return rating;
}
public void setRating(String rating) {
this.rating = rating;
}
/**
* return a formatted string containing the data for the movie
*/
@Override
public String toString() {
String output = "ID: " + id + " ** Title: " + title + " ** Distributor: " + distributor + " ** Release date: " + releaseDate + " "
+ " ** Movie description: " + info + " "
+ " ** Domestic sales in dollars: " + domesticSales + " ** International Sales in dollars: " + intlSales + " "
+ " ** Genre: " + genre + " ** Rating: " + rating + " ";
return output;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((distributor == null) ? 0 : distributor.hashCode());
result = prime * result + (int) (domesticSales ^ (domesticSales >>> 32));
result = prime * result + ((genre == null) ? 0 : genre.hashCode());
result = prime * result + ((id == null) ? 0 : id.hashCode());
result = prime * result + ((info == null) ? 0 : info.hashCode());
result = prime * result + (int) (intlSales ^ (intlSales >>> 32));
result = prime * result + ((rating == null) ? 0 : rating.hashCode());
result = prime * result + ((releaseDate == null) ? 0 : releaseDate.hashCode());
result = prime * result + ((title == null) ? 0 : title.hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
Movie other = (Movie) obj;
if (distributor == null) {
if (other.distributor != null)
return false;
} else if (!distributor.equals(other.distributor))
return false;
if (domesticSales != other.domesticSales)
return false;
if (genre == null) {
if (other.genre != null)
return false;
} else if (!genre.equals(other.genre))
return false;
if (id == null) {
if (other.id != null)
return false;
} else if (!id.equals(other.id))
return false;
if (info == null) {
if (other.info != null)
return false;
} else if (!info.equals(other.info))
return false;
if (intlSales != other.intlSales)
return false;
if (rating == null) {
if (other.rating != null)
return false;
} else if (!rating.equals(other.rating))
return false;
if (releaseDate == null) {
if (other.releaseDate != null)
return false;
} else if (!releaseDate.equals(other.releaseDate))
return false;
if (title == null) {
if (other.title != null)
return false;
} else if (!title.equals(other.title))
return false;
return true;
}
}
movieList.java ---------
import java.util.ArrayList;
importjava.util.List;
public class MovieList {
private ArrayList
/**
* Adds a movie to the list if it isn't already there If the id already
* exists on the list, return false. Otherwise, add the movie and return true
*/
public Boolean add(Movie movie) {
if (findById(movie.getId()) == null) {
movies.add(movie);
return true;
}
return false;
}
/**
* locate and return a Movie based on its id. If a Movie with the id does not
* exist on the list return null. Otherwise, return the matching Movie
*/
public Movie findById(String id) {
for (Movie movie : movies) {
if (movie.getId().equals(id)) {
return movie;
}
}
return null;
}
public int numMovies() {
return movies.size();
}
}
movieReader.java ----------
import java.io.File; import java.io.FileNotFoundException; import java.time.LocalDate; import java.time.format.DateTimeFormatter; import java.util.Scanner;
/** * This class manages the task of reading movies from a file * Each record in the file is assumed to contain pipe delimited values * */ public class MovieReader { public static MovieList load(String filepath) { // code that opens the file, creates a Scanner, and // reads each line in the file into a String, until // the end of file is reached. For each line, it // should call readLine. } /** * read one movie from a String containing the field values * This method expects a Scanner object associated with an open file * * This method is meant to be called repetitively in order to read multiple * movies from a file * @param a String containing pipe delimitied values for one movie * @return a Movie object or null if the string is empty */ public static Movie readLine(String line){ // create Scanner with line as the parameter // set the delimiter to '|' // read each field into a String variable. // for the releaseDate field, convert to a LocalDate, // handling the case where it is "NA" according to // the lab instructions // create Movie with the string and LocalDate values // and return it.
} }
ReaderTtesty.java ---------
public class ReaderTesty {
public static void main(String[] args) {
MovieList list = MovieReader.load("moviesShort.txt");
System.out.println("list len is " + list.numMovies() + " records");
if (list.numMovies()!= 10) {
System.err.println("Got incorrect number of movies");
}
Movie movie;
for (int i=0;i String id = Integer.toString(i); movie = list.findById(id); System.out.println(movie); } } } TEXTFILE -------- 0|Star Wars: Episode VII - The Force Awakens (2015)|"As a new threat to the galaxy rises,Rey, a desert scavenger, andFinn, anex-stormtrooper, must joinHanSolo andChewbaccato search for the one hope of restoring peace."|WaltDisneyStudios Motion Pictures|16-12-2015|936662225|1132859475|"['Action', 'Adventure', 'Sci-Fi']"|PG-13 1|Avengers:Endgame(2019)|"After the devastating events ofAvengers: Infinity War, the universe is in ruins. With the help of remaining allies, theAvengersassemble once more in order to reverseThanos' actions and restore balance to the universe."|WaltDisneyStudios Motion Pictures|24-04-2019|858373000|1939128328|"['Action', 'Adventure', 'Drama', 'Sci-Fi']"|PG-13 2|Avatar(2009)|AparaplegicMarine dispatched to the moonPandoraon a unique mission becomes torn between following his orders and protecting the world he feels is his home.|Twentieth Century Fox|16-12-2009|760507625|2086738578|"['Action', 'Adventure', 'Fantasy', 'Sci-Fi']"|PG-13 3|Black Panther (2018)|"T'Challa, heir to the hidden but advanced kingdom ofWakanda, must step forward to lead his people into a new future and must confront a challenger from his country's past."|WaltDisneyStudios Motion Pictures|NA|700426566|647171407|"['Action', 'Adventure', 'Sci-Fi']"|NA 4|Avengers: Infinity War (2018)|TheAvengersand their allies must be willing to sacrifice all in an attempt to defeat the powerfulThanosbefore his blitz of devastation and ruin puts an end to the universe.|WaltDisneyStudios Motion Pictures|NA|678815482|1369544272|"['Action', 'Adventure', 'Sci-Fi']"|NA 5|Spider-Man: No Way Home (2021)|"With Spider-Man's identity now revealed, Peter asks Doctor Strange for help. When a spell goes wrong, dangerous foes from other worlds start to appear, forcing Peter to discover what it truly means to be Spider-Man."|SonyPictures Entertainment (SPE)|NA|675813257|868642706|"['Action', 'Adventure', 'Fantasy', 'Sci-Fi']"|NA 6|Titanic(1997)|"A seventeen-year-old aristocrat falls in love with a kind but poor artist aboard the luxurious, ill-fated R.M.S.Titanic."|Paramount Pictures|19-12-1997|659363944|1542283320|"['Drama', 'Romance']"|PG-13 7|JurassicWorld (2015)|"A new theme park, built on the original site ofJurassicPark, creates a genetically modified hybriddinosaur, theIndominusRex, which escapes containment and goes on a killing spree."|Universal Pictures|10-06-2015|652385625|1018130819|"['Action', 'Adventure', 'Sci-Fi']"|PG-13 8|TheAvengers(2012)|Earth's mightiest heroes must come together and learn to fight as a team if they are going to stop the mischievousLokiand his alien army from enslaving humanity.|WaltDisneyStudios Motion Pictures|25-04-2012|623357910|895457605|"['Action', 'Adventure', 'Sci-Fi']"|PG-13 9|Star Wars: Episode VIII - The LastJedi(2017)|"The Star Warssagacontinues as new heroes andgalacticlegends go on an epic adventure, unlocking mysteries of the Force and shocking revelations of the past."|WaltDisneyStudios Motion Pictures|13-12-2017|620181382|712517448|"['Action', 'Adventure', 'Fantasy', 'Sci-Fi']"|PG-13
Step by Step Solution
There are 3 Steps involved in it
Step: 1
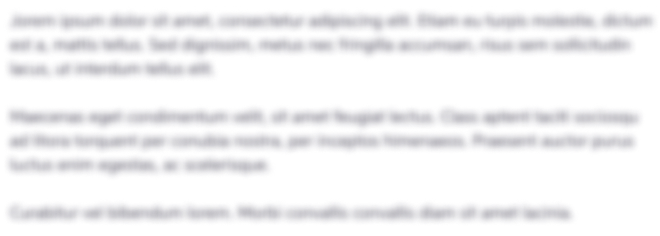
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started