Question
PLEASE REMEMBER YOU HAVE TO WRITE SHELL PROGRAM WITH PIPES WHICH CAN EXECUTE TWO COMMANDS LIKE ls -l | wc -l. I DON'T NEED THE
PLEASE REMEMBER YOU HAVE TO WRITE SHELL PROGRAM WITH PIPES WHICH CAN EXECUTE TWO COMMANDS LIKE ls -l | wc -l. I DON'T NEED THE ANSWER ALREADY IN CHEGG WHICH ARE INCORRECT. PLEASE DO IT CORRECTLY.
Your job is to implement an advanced UNIX/Linux shell in C++ capable for executing a sequence of programs that communicate through a pipe. More specifically, for example, if the user types ls | wc, your program should fork off the two programs, which together will calculate the number of files in the directory.
Our goal for this part of the Lab is two-fold:
- To exercise your C++/Linux skills
- And to get you to think about a clean design that handles the concurrency aspect of the shell
Because of this, you shouldn't worry about any of the more complex aspects of shells, like supporting command line arguments, environment variables, or other shell features (&, >, etc.).
All you need to do is handle the execution of programs and being able to pipe stdout from one program to the stdin of another. For this, you will need to use several of the Linux system calls described above in Part # 1: fork, exec, open, close, pipe, dup2, and wait. Note: You will have to replace stdin and stdout in the child process with the pipe file descriptors; that is the role of dup2.
Part 2: Specification
- Read commands to execute from stdin. A command is terminated by a newline character (' ') and consists of one or more sequence of programs separated by the string " | ".
- Continue reading and executing commands until stdin returns EOF.
- Wait for the current command to terminate before starting the next command.
- The child programs of a command must execute in parallel (effective use of pipe, |)
- Programs can be named by either an absolute path or just by the program name (exec*p should handle this for you).
- For input exit, break the infinite while loop
- Print an easy-to-parse prompt to stdout
Part 2: Hints
To solve this programming exercise, you'll need to use some (but perhaps not all) of the following Linux system calls: fork, exec*p, close, pipe, dup2, and wait. As a hint, you should use pipe to create a pipe, fork to execute the programs, and dup2 to replace stdin or stdout, and wait to wait for the processes to terminate, probably in that order.
- In case stdin not recognizable in dup2, just can use 0
- In case stdout not recognizable in dup2, just can use 1
You might also want to look at the strtok()/ split function, this will simplify the job of parsing user input in your shell. Following is intended just as a sketch of a solution:
#include
#include
int main(int argc, char *argv[]) {
char *prog1 = NULL, *prog2 = NULL;
char **args1 = NULL, **args2 = NULL;
int pipefd[2]; // file descriptors for the pipe
int childpid1 , childpid2 ;
// we omit the details of parsing a pipe command
while (readAndParsePipeCmd(&prog1 , &args1 , &prog2 , &args2 ))
{
// create a pipe with two open file descriptors
// can write to pipefd[1] and read from pipefd[0]
// open file descriptors are inherited across fork
pipe(pipefd);
// Create the first child process
childpid1 = fork ();
if ( childpid1 == 0) {
// I m the first child process.
// Run prog1 with stdout as the write end of the pipe
dup2(pipefd [1] , stdout );
exec(prog1 , args1 );
// NOT REACHED
}
else {
// I m the parent
// Create the second child process
childpid2 = fork ();
if ( childpid2 == 0) {
// I m the second child process.
// Run prog2 with stdin as the read end of the pipe
dup2(pipefd [0] , stdin ); exec(prog2 , args2 );
// NOT REACHED
} else {
// I m the parent
wait(childpid2);
wait(childpid1);
return 0;
}
}
} // end while
} // end main
Step by Step Solution
There are 3 Steps involved in it
Step: 1
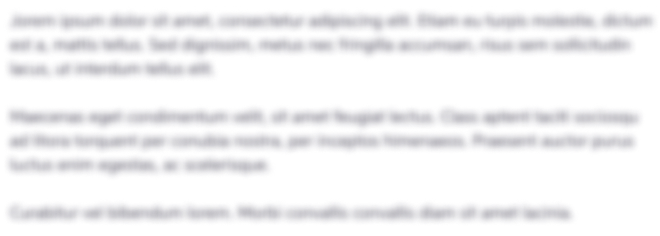
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started