Answered step by step
Verified Expert Solution
Question
1 Approved Answer
please use C++ language. The initial code is pasted below: include ref_manager.h # include cassert > ReferenceManager::ReferenceManager () { numToDelete = 0 ;
please use C++ language. The initial code is pasted below:
cated eventually, otherwise it will cause memory leak. While some programming languages (e.g., C and C++ ) require programmers to manually free the memory once they are finished with it, other programming languages (e.g., Python, Java and Go) automatically deallocate the memory for the programmer. This automatic deallocation of memory is called garbage collection. Specifically, the memory that no longer has a variable referencing it is periodically cleaned up, without involving the programmer in this process. In this exercise you will emulate a garbage collector. The garbage collector will allocate and deallocate memory for strings represented as character arrays. These strings will not contain a space character. Internally, the garbage collector uses an array of 100 pointers that can point to the allocated strings and another array of 100 pointers to store strings that are no longer referenced by a pointer in the first array. The design of our garbage collector is described below: - const char *pointers[MAX_PTRS]: An array of 100 pointers to strings that have been created. - const char * garbage [MAXGARBAGE] : An array of 100 pointers to strings that were created and are no longer referenced by the pointers array. - int numToDelete: The number of pointers to strings in the garbage array that must be deleted the next time garbage collection is requested. - unordered_map refcount: An unordered map to keep track of the number of pointers in the pointers array that are referencing each string. So, for example, if the pointer at index 17 and index 99 both reference the string "he110", then the unordered map would contain the following key-value pair: { "he 110",2}. The garbage collector needs to support the following queries: - c p str This query will allocate memory for a new string. p and n are both integers, with p being the index of the pointer that should point to this new string and n being the length of the string (not including the null character). str is the string to be stored. All strings provided will consist of exactly n alphanumeric characters with no spaces. For example, the query c 175 he 110 would point the pointer at index 17 in the pointers array to the newly created string "he 110. - s1p2 This query will cause the pointer p1 to reference what is referenced to by p2. For example, if pointer 17 references the string "he 110", the query s 117 would cause the pointer at index 1 to also reference the string "he 110". - q p This query will print the string that is referenced to by p or NULL if p is not currently referencing any string. - This query will cause the garbage collection to take place. Any strings in the garbage array must be deallocated using delete. - Te This query will exit the program. The logic for determining which query to run, as well as reading the pointer indexes and string lengths (for the queries that require them) has been implemented already in we3_test. cpp. Do not modify this driver file. It instantiates an object of class ReferenceManager and parses the queries. Your job is to implement some of the methods in the Referencemanage class, as described below. Required Methods and Destructor - readstring(unsigned int ptrindex, unsigned int length) This method will allocate space for a string of size length using new. Remember that you must include space for the terminating null character. Then it must read a string of size length from the standard input and store it in the allocated space. The string provided to your program will consist of exactly length alphanumeric characters with no spaces. Finally it must use the method reassignpointer () to have the pointer at index ptrindex reference the newly allocated string. - reassignpointer (unsigned int ptrindex, const char *newAddr) This method will make the pointer at ptrindex in the pointers array reference newAddr. This method should support reasignment to NULL, i.e. newAddr can be NULL. In addition to doing this, the reference counts must be updated in the unordered map refcount. In order to know when a string must be garbage collected, we must keep track of the number of pointers in the pointers array that reference each string. Every time reassignpointer () is called the reference count for both the string previously pointed to by ptrindex and the string ptrindex is now pointing to must be updated. If this causes a string 's reference count to fall to 0 (i.e., no pointers in the pointers array reference it) then the string should be added to the garbage array, to be deleted the next time garbage collection is requested. - garbagecollect () This method will go through all the strings in the ga rbage array, print each string and then free the memory using the delete operator. The strings should be printed in the order they were added to the garbage array. - destructor The destructor for this class should go through and free any memory that is currently allocated. This means that it must go through all pointers in the pointers array from the first index to the last one and call reassignPointer () to reassign all pointers in the array to NULL. Then the garbageCollect () method must be called to free the memory. Thus, the output from the final garbage collection call from the destructor will first consists of all strings that were already in the garbage array before the destructor was called (as they were already scheduled for garbage collection) and then all remaining strings in the order their reference counts dropped to 0 as their pointers were reassigned to NULL. Feel free to add private methods or attributes if you think they might help. However, you are not allowed to add new public methods or attributes to the ReferenceManager class. Standard Ct+ header files can be included if needed. There is no Big-O running time bound for this problem. The test data is not very large and a correct solution will likely run in a fraction of a second. Other Remarks - A correct solution will produce output in a unique way. As specified above, the garbage collection will print out the strings being collected in the order their reference counts dropped to 0 . The destructor will first collect all remaining strings for garbage collection by reducing their reference counts one at a time, starting from the first pointer, and then garbage collection will be performed. If you adhere to this specification, the output of a correct solution is unique. - We will provide test data such that a correct solution will never queue up more than 100 pointers for garbage collection at a time (i.e., between garbage collection runs, at most 100 pointers will be added). This even includes the final step in a correctlyimplemented destructor (when the strings with references are added to the garbage collector during the destructor). Example Runs Sample Input 1 cqe17175hello Sample Output 1 Sample Output 2 include "ref_manager.h" | |
#include cassert> | |
ReferenceManager::ReferenceManager() { | |
numToDelete = 0; | |
for (int i = 0; i | |
pointers[i] = NULL; | |
} | |
} | |
ReferenceManager::~ReferenceManager() { | |
// CODE HERE | |
} | |
void ReferenceManager::garbageCollect() { | |
// CODE HERE | |
} | |
void ReferenceManager::reassignPointer(unsigned int ptrIndex, const char* newAddr) { | |
// CODE HERE | |
} | |
void ReferenceManager::readString(unsigned int ptrIndex, unsigned int length) { | |
// CODE HERE | |
} | |
const char* ReferenceManager::getPtr(unsigned int ptrIndex) { | |
assert(ptrIndex | |
return pointers[ptrIndex]; | |
} |




Step by Step Solution
There are 3 Steps involved in it
Step: 1
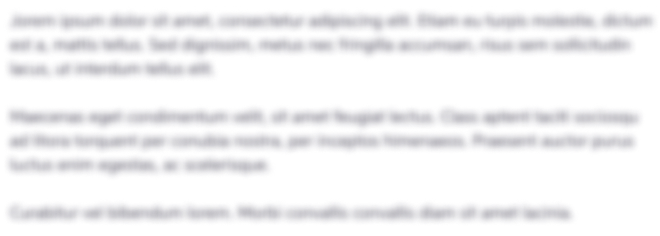
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started