Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Please write a C programing code for the following problem. Thank you! PLEASE HELP ME OUT WITH THIS PROJECT. THAN YOU! 1 Overview Write a
Please write a C programing code for the following problem. Thank you!
PLEASE HELP ME OUT WITH THIS PROJECT. THAN YOU!
1 Overview Write a C program to implement a cellular automaton simulation of fire spreading through a forest. The initial state of the forest will be inputted from a text file and you will then process the fire spreading through the forest until no more fire exists then outputting the final state of the forest after the fire. 2 Cellular Automata A cellular automaton is a collection of cells on a grid (think of a two-dimensional space rep- resented by a two-dimensional array in a program) of a specified shape that evolves through a number of discrete time steps according to a set of rules based on the states of neighboring cells. The rules are then applied iteratively for as many time steps as desired. For example, consider this small 3-by-3 grid with each cell containing a blank or the letter X: Generation #1 1 2 3 1 2 X 3 X Now we wish to look at each cell in turn and apply the following rules: if a cell has exactly 2 neighbor cells (don't count the cell being considered!) with an x then an x will remain or be created in that cell in the next generation (a new 3-by-3 grid) else the same cell in the next generation will contain a blank So applying these rules to each cell one by one in the example above yields the following grid (here we are considering diagonal cells as neighbors): Generation #2 1 2 3 1 X X 2 X 3 Our automaton would continue by applying the same rules to this new generation to produce the next one: 1 Generation #3 1 2 3 1 Cellular automatons usually continue like this producing generation after generation until some defined stop state is reached. Note that in the above example the next generation (generation #4) would contain all blanks. Cellular automatons can be very useful in modeling stochastic systems across many fields. https://en.wikipedia.org/wiki/Cellular_automaton 3 Fire in Our Forest If a cell in our forest is on fire, then that fire spreads to all neighboring cells in one generation with some probability determined by factors such as wind, precipitation falling, dryness of leaves and underbrush, etc. While it would be possible in a more complicated simulation to model all of this uncertainty, we are going to greatly simplify things by providing a single probability that at each iteration fire will spread from one burning cell to another. We will specify this probability, as a percentage from 0% to 100% in our input file. In other words if there is a 100% probability that fire will spread it then will always catch a neighboring cell on fire, or if the probability is 50% then only half of the time will a neighboring cell actually catch on fire in one generation, etc. We will use a random number generator to determine the probability of fire spreading; more on this later. To simplify matters somewhat we will not be considering diagonal cells as neighbors; therefore example cell (r,c) below has only 4 neighbors: c+1 T-1 1 (r.c-1) (r-1.c) (rc) (r+1,c) (r,c+1) r+1 Fire completely burns a tree in one generation leaving behind an empty cell. 4 Implementation 4.1 Representation of the Forest You will represent your forest as an x-b two dimensional array of integers Each cell in your array will contain one of 3 possible values: O means the cell is empty, 1 means it contains a tree, and 2 means fire. The initial state of your forest will be determined by input from a text file (explained below). We will initialize the first and last rows plus the first and 2 last columns (i.e. all the border squares) to empty (0) to make your program logic easier; think of it as a built-in fire barrier (fire will not be allowed to jump empty spaces). The largest forest we will consider is 100-by-100; therefore to represent a 100-by-100 forest you will need to create an array with at least 102 rows and 102 columns. 4.2 Program input The initial state of the forest will be specified by input from a text file whose name will be given on the command line. The text file will have the following format; note that bold remarks below are for explanation and not part of the actual data in the file: 8 + number of rows 20 + number of columns 28 + random number generator seed s (exit program with message if s >> Time: 19 12345678901234567890 1 2 3 3 4 4 5 6 7 8 TT 8 12345678901234567890 1 2 total burned: 89 (63.1%) trees left: 52 homebox:-/cprogs/% Note that the C standard does not require that the function rand() will give the same sequence of values for the same seed across different machines! Therefore your results might and probably will be slightly different than mine given above even with 6 the same inputs. 6 Implementation Suggestions Some general suggestions on how to approach this project. 1. Begin by getting your file input and the printing of your initial configuration correct for a variety of forest sizes. 2. Use the following general algorithm as a starting point for your program: initializations input data set the time output initial info while there is still fire in the forest do compute next generation increment time endwhile output final generation and info 3. Create a debug mode where you print out every generation that is created. When you do this you will need to pause the output after each generation; probably the easiest way to do this: void pause() { printf("
Step by Step Solution
There are 3 Steps involved in it
Step: 1
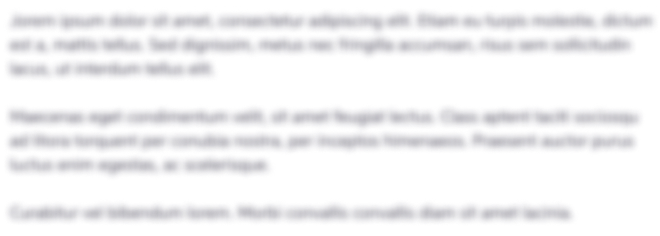
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started