Question
Please Write the program in C++ language: Problem Description: 1. Create NumDays Class. Create a class to store a value that represents number of hours
Please Write the program in C++ language:
Problem Description:
1. Create NumDays Class.
Create a class to store a value that represents number of hours worked and number
of days worked. Must ensure that the two representations are always consistent.
2. Create TimeOff Class
Create a TimeOff Class is to track an employees sick leave and vacation time.
It should consist of the following members: maxSickDays, sickTaken,
maxVacation, and vacTaken. These members should be of type NumDays.
An employee, after one year of service, receives at the beginning of the year 160
hrs(20 days) of vacation time that must be taken in that year.
Also received is 40 hrs(5 days) of sick leave available to be take in that year.
3. Create TimeOff Report
Create a Report that starts with starts with a Time Bank that has 160 hrs of
Vacation Time and 40 hrs of available Sick Leave.
Create a scenario to use vacation and sick leave to demonstrate ALL class methods.
Program Structure
#include
#include "NumDays.h"
#include
using namespace std; //NumDays Class class NumDays { private: double hours; double days; public: NumDays(double h); void setHours(double h); double getHours() const; void setDays(double d); double getDays() const; //TimeTaken Class #include "NumDays.h" class TimeTaken { private: string name; NumDays maxSickDays, sickTaken, maxVacation, vacTaken; public: //Constructor TimeTaken(string, double, double);
//Mutators void setMaxSick(double h); void setSickTaken(double h); void setMaxVacation(double h); void setVacationTaken(double h); public: // Accessors string getName() const; double getMaxSick() const; double getSickTaken() const; double getMaxVacation() const; double getVacationTaken() const; double getMaxSickDays() const; double getSickTakenDays() const; double getMaxVacationDays() const; double getVacationTakenDays() const; //Embedded consructors /Embedded Constructors TimeTaken ( string n, double mS, double mV ) : name(n), maxSickDays(mV), sickTaken(0), maxVacation(mV), vacTaken(0) { } //Embedded Mutator void setMaxSick(double h) { maxSickDays.setHours(h); }
//Embedded Accessor double getMaxSick() const { return maxSickDays.getHours(); } }; //TimeBank Report TimeTakenamy("Amy Adams", 40, 160); cout<<"Beginning of the Year:" << endl; cout<< "Available vacation: " << amy.getMaxVacation() << " hours "; cout<< "Available vacation: " << amy.getMaxVacationDays() << " days "; cout<<" Takefive days of Vacation: "<
Step by Step Solution
There are 3 Steps involved in it
Step: 1
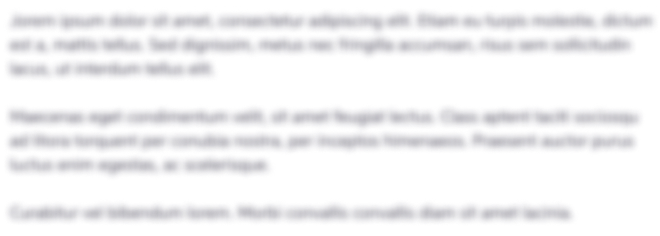
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started