Question
pls help fill in parts to complete i am lost. Here's a summary of the modifications needed for each part: Part 1: Write C program
pls help fill in parts to complete i am lost.
Here's a summary of the modifications needed for each part:
Part 1: Write C program based on the provided sample code for "smallsh" by creating multiple source code files (smallsh.c, smallsh.h, userinput.c, processline.c, runcommand.c) and a Makefile. Ensure your shell can execute Linux commands and exit gracefully.
Part 2: Modify your shell to interpret comments indicated by a leading '#' character. This means that any line starting with '#' should be treated as a comment and not executed as a command.
Part 3: Extend your shell to support the "cd" (change directory) command. Implement logic to change the current working directory based on the provided directory argument or to the user's home directory if no argument is given. Display an error message if the target directory does not exist or has insufficient permissions.
Part 4: Enhance your shell to support I/O redirection for standard input and output. Use the '<' character to indicate input redirection from a file and the '>' character to indicate output redirection to a file. Handle errors appropriately, such as displaying an error message if the input file doesn't exist.
Part 5: Improve your shell's signal handling. Allow the "control-C" keyboard interrupt to terminate a foreground process started by the shell without killing the shell itself. Ensure that background processes started from within the shell are not terminated by SIGINT or SIGQUIT generated from the keyboard. Optionally, consider supporting the "suspend" keyboard interrupt (usually control-Z) for foreground and background process control.
Bonus: Extend your shell to support the pipe ('|') feature. Detect the presence of the pipe character in the command arguments and create a pipe. Fork a child process for each command in the pipe, redirecting the standard output of the first command to the write end of the pipe and the standard input of the second command to the read end of the pipe.
These modifications require changes in various files, including userinput.c, processline.c, runcommand.c, and possibly others. The provided code snippets demonstrate the required changes, but you'll need to integrate them properly into your existing codebase.
Explanation:
Explanation for each part of the assignment in detail and provide the necessary code snippets.
Part 1: Creating the Shell (myshell)
To create the shell, you'll need to create multiple source code files and a Makefile. Based on the provided sample code for "smallsh," you can modify and create the following files:
1. smallsh.c: This file will contain the main function of the shell.
#include "smallsh.h" int main() { // Code for executing the shell return 0; }
2. smallsh.h: This file will contain the function declarations and necessary header files.
#ifndef SMALLSH_H #define SMALLSH_H // Include necessary header files here // Function declarations #endif
3. userinput.c: This file will handle user input and parsing.
#include "smallsh.h" char* get_user_input() { // Code for reading user input and returning it as a string } char** parse_input(char* input) { // Code for parsing the input string into an array of arguments (tokenization) }
4. processline.c: This file will handle processing the user input and executing commands.
#include "smallsh.h" void process_line(char** args) { // Code for processing the user input (e.g., checking for built-in commands or executing external commands) }
5. runcommand.c: This file will handle executing external commands.
#include "smallsh.h" void execute_command(char** args) { // Code for executing external commands (e.g., using fork, execvp, wait) }
6. Makefile: This file will specify the compilation instructions for building the shell.
CC = gcc CFLAGS = -Wall all: myshell myshell: smallsh.o userinput.o processline.o runcommand.o $(CC) $(CFLAGS) -o myshell smallsh.o userinput.o processline.o runcommand.o smallsh.o: smallsh.c $(CC) $(CFLAGS) -c smallsh.c userinput.o: userinput.c $(CC) $(CFLAGS) -c userinput.c processline.o: processline.c $(CC) $(CFLAGS) -c processline.c runcommand.o: runcommand.c $(CC) $(CFLAGS) -c runcommand.c clean: rm -f myshell *.o
Make sure to adjust the code and Makefile according to your specific needs.
Part 2: Interpreting Comments
To interpret comments in the shell, you need to modify the `process_line` function in the `processline.c` file.
void process_line(char** args) { // Check for comments if (args[0][0] == '#') { // Ignore the comment and return return; } // Rest of the code for processing the command }
This modification checks if the first argument starts with a '#' character and ignores the command if it does. This way, comments are properly interpreted and not executed as commands.
Part 3: Supporting "cd" Command
To support the "cd" command, you need to modify the `execute_command` function in the `runcommand.c` file.
void execute_command(char** args) { // Check if the command is "cd" if (strcmp(args[0], "cd") == 0) { // Change directory if (args[1] == NULL) { // No arguments provided, change to the user's home directory chdir(getenv("HOME")); } else { // Change to the specified directory if (chdir(args[1]) != 0) { // Directory change failed perror("cd"); } } } else { // Rest of the code for executing external commands } }
This modification checks if the command is "cd" and handles the directory change accordingly. If no arguments are provided, it changes to the user's home directory. If a directory is specified, it attempts to change to that directory using the `chdir` function.
Part 4: I/O Redirection
To support I/O redirection for standard input and standard output, you need to modify the `execute_command` function in the `runcommand.c` file.
void execute_command(char** args) { // Check for input/output redirection int input_fd = STDIN_FILENO; // Default input is stdin int output_fd = STDOUT_FILENO; // Default output is stdout // Search for "<" or ">" int i = 0; while (args[i] != NULL) { if (strcmp(args[i], "<") == 0) { // Input redirection args[i] = NULL; // Remove the "<" from the arguments char* input_file = args[i + 1]; input_fd = open(input_file, O_RDONLY); if (input_fd < 0) { perror("open"); return; } } else if (strcmp(args[i], ">") == 0) { // Output redirection args[i] = NULL; // Remove the ">" from the arguments char* output_file = args[i + 1]; output_fd = open(output_file, O_WRONLY | O_CREAT | O_TRUNC, 0644); if (output_fd < 0) { perror("open"); return; } } i++; } // Rest of the code for executing the command with the specified input/output file descriptors // Close the file descriptors if they were modified if (input_fd != STDIN_FILENO) { close(input_fd); } if (output_fd != STDOUT_FILENO) { close(output_fd); } }
This modification searches for "<" and ">" in the command arguments and performs input and output redirection accordingly. It uses the `open` system call to open the specified input/output file and updates the file descriptors for stdin and stdout accordingly. The command is then executed with the modified file descriptors.
Part 5: Handling Signals
To handle signals like a real Linux shell, you need to modify the `main` function in the `smallsh.c` file.
#include "smallsh.h" // Signal handler function void handle_signal(int signo) { // Handle the signal // For example, if signo is SIGINT, terminate the foreground process } int main() { // Set up signal handlers signal(SIGINT, handle_signal); // Rest of the code for executing the shell }
This modification adds a signal handler function (`handle_signal`) and registers it using the `signal` function. Inside the signal handler function, you can handle specific signals like SIGINT (generated by Ctrl+C) to terminate the foreground process.
To handle background processes not being terminated by SIGINT or SIGQUIT, you need to modify the `execute_command` function in the `runcommand.c` file. You can use the `sigaction` function to ignore the signals for background processes.
void execute_command(char** args) { // Check if the command is running in the background int is_background = 0; int i = 0; while (args[i] != NULL) { if (strcmp(args[i], "&") == 0) { is_background = 1; args[i] = NULL; // Remove the "&" from the arguments break; } i++; } // Fork and execute the command // In the child process if (pid == 0) { // Ignore SIGINT and SIGQUIT if it's a background process if (is_background) { struct sigaction sa; sa.sa_handler = SIG_IGN; sigemptyset(&sa.sa_mask); sa.sa_flags = 0; sigaction(SIGINT, &sa, NULL); sigaction(SIGQUIT, &sa, NULL); } // Execute the command // Exit the child process exit(0); } // Rest of the code for the parent process }
This modification checks if the command is running in the background by searching for "&" in the command arguments. If it is a background process, it modifies the signal action using `sigaction` to ignore SIGINT and SIGQUIT.
Bonus Part: Supporting Pipe Feature
To support the pipe feature, you need to modify the `execute_command` function in the `runcommand.c` file.
void execute_command(char** args) { // Check for pipe character "|" int is_piped = 0; int i = 0; while (args[i] != NULL) { if (strcmp(args[i], "|") == 0) { is_piped = 1; args[i] = NULL; // Remove the "|" from the arguments break; } i++; } // Fork and execute the command // In the child process if (pid == 0) { if (is_piped) { // Create a pipe int pipefd[2]; if (pipe(pipefd) == -1) { perror("pipe"); exit(1); } // Fork again for the second command pid_t pid2 = fork(); if (pid2 == -1) { perror("fork"); exit(1); } else if (pid2 == 0) { // Child process of the second command // Close the write end of the pipe close(pipefd[1]); // Redirect stdin to the read end of the pipe dup2(pipefd[0], STDIN_FILENO); // Execute the second command execvp(args[i + 1], &args[i + 1]); perror("execvp"); exit(1); } else { // Parent process // Close the read end of the pipe close(pipefd[0]); // Redirect stdout to the write end of the pipe dup2(pipefd[1], STDOUT_FILENO); // Execute the first command execvp(args[0], args); perror("execvp"); exit(1); } } else { // Execute the command // Exit the child process exit(0); } } // Rest of the code for the parent process }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
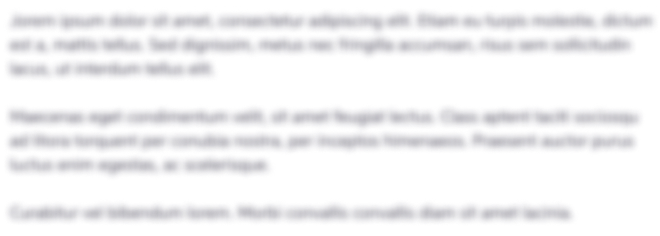
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started