Question
Problem 1) Memory Leak Scavenger Hunt A memory leak is a failure in a program to release discarded memory, causing impaired performance or failure. Common
Problem 1) Memory Leak Scavenger Hunt
A memory leak is "a failure in a program to release discarded memory, causing impaired performance or failure."
Common causes of memory leaks in code students submit are:
-Dynamically allocating memory for a local variable and forgetting to release it before that variable goes out of scope.
-Releasing memory for a double pointer (i.e. a pointer to an array of pointers), without first releasing the memory allocated for the elements held in the array.
-Failing to declare a base class destructor as virtual, and then deleting an instance of a derived-class via a base-class type pointer.
If the last item above seems confusing, review inheritance and dynamic binding in the course notes. When you attempt to de-allocate memory in such a situation the compiler will not check the type of the object being pointed to. Assuming that the object is of type base class, the base class destructor will be called. However, if the derived class happens to have additional data elements stored on the heap, their memory will not be released. In other words: declaring a destructor as virtual means that object type will be checked at run time, as opposed to compile time.
Exercise
The demo code provided has three memory leaks (similar to the ones described above). Find them and fix them.
demo code :
#ifndef STACK_H #define STACK_H // Class Stack is a template for a bounded LIFO stack. // Note that Stack only stores the pointers to its items (i.e. it is not "responsible" for its contents). templateclass Stack { public: // Default constructor Stack(const int size = 10) : capacity(size), numberOfItems(0) { if(size < 1) { capacity = 10; } buffer = new T * [capacity]; } // Destructor ~Stack() { delete [] buffer; } // Checks if the stack contains any elements bool empty() const { return (numberOfItems < 1); } // Checks if the stack is full bool full() const { return (numberOfItems == capacity); } // Pushes a pointer to an element onto the stack, returns itself Stack & push(T * item) { if(numberOfItems < capacity) { buffer[numberOfItems++] = item; } return *this; } // Pops an elements off the stack, returns itself Stack & pop() { if(!empty()) { numberOfItems--; } return *this; } // Returns the pointer to the element at the top of the stack T * peek() const { if(!empty()) { return buffer[numberOfItems-1]; } return NULL; } // Output the contents of the stack to a stream virtual void printOn(ostream & outstream) const { outstream << "Contents: "; if(!empty()) { for(int i=0; i < numberOfItems; i++) { outstream << *(buffer[i]) << endl; } } else { outstream << "EMPTY "; } return; } private: T ** buffer; int capacity, numberOfItems; }; template ostream & operator<<(ostream & ostr, const Stack & aName) { aName.printOn(ostr); return ostr; }; #endif // STACK_H
************************************
#define _CRTDBG_MAP_ALLOC // -------------- SOLUTION CODE ------------------ #include#include #include using namespace std; #include "Stack.h" #include "LabelledStack.h" #define STACKSIZE 10 void t9() { cout << "----- T 9 - EASTER EGG ... ERR, MEMORY LEAK HUNT ----- "; // Make a couple of stacks Stack * stack = new Stack (); Stack * labelledStack = new LabelledStack (STACKSIZE, "The Mighty Stack of int Pointers"); // Generate some random numbers cout << "> generating some random numbers and stuffing them into an array "; int ** numbers = new int*[STACKSIZE]; for(int i=0; i full()); i++) { stack->push((numbers[i])); } // Print out contents of the stack cout << "> stack " << *stack << endl; // Print out contents of the labelledStack cout << "> labelledstack " << *labelledStack << endl; // Empty the stack into labelledStack cout << "> popping off the stack and pushing into labelled stack "; while(!(stack->empty())) { labelledStack->push(stack->peek()); stack->pop(); } // Print out contents of the stack cout << "> stack " << *stack << endl; // Print out contents of the labelledStack cout << "> labelledstack " << *labelledStack << endl; // Clean-up! delete stack, labelledStack; delete [] numbers; cout << "----- THE END ------ "; return; } int main() { t9(); return 0; }
*******************************************
#ifndef LABELLEDSTACK_H #define LABELLEDSTACK_H #include "Stack.h" // (Derived) Class LabelledStack is a template for a bounded LIFO stack with a label. // Note that Stack only stores the pointers to its items (i.e. it is not "responsible" for its contents). templateclass LabelledStack : public Stack { public: // Default constructor LabelledStack(const int size, string newLabel) : Stack (size){ this->label = new string(newLabel); } // Destructor ~LabelledStack() { delete label; } // Output the contents of the stack to a stream void printOn(ostream & outstream) const { outstream << "Stack label: " << *label << endl; Stack ::printOn(outstream); return; } private: string * label; }; #endif // LABELLEDSTACK_H
Step by Step Solution
There are 3 Steps involved in it
Step: 1
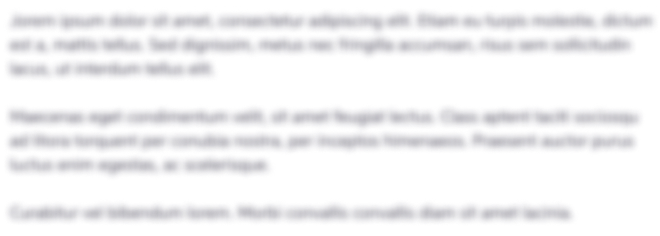
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started