Question
Problem - VERY easy C program (when completed please post all source code and screenshot of compiled output) Add serialization (1), StoreVECTOR() , and deserialization
Problem - VERY easy C program (when completed please post all source code and screenshot of compiled output)
Add serialization (1), StoreVECTOR(), and deserialization (2), LoadVECTOR(), member functions to the VECTOR abstract data type. The member functions labeled (1) and (2) below are fully complete, I just don't know where to specifially add them in the code so please put them in the appropriate area.
Create a project with the five source files ADTExceptions.h, ADTExceptions.c, Vector.h, Vector.c, and Problem2.c (all five files located below) and build load module Problem2.exe. Play with the program enough to prove that the program works correctly.
Please take screenshot of it compiled once complete (which should look sorta similar to the sample one provided) and copy&paste full source code will be much appreciated.
Submit the new versions of Vector.h and Vector.c along with
SCREENSHOT which should look like the sample one provided.
(1) This is the StoreVECTOR() member function that needs to be added to the code. (i think somewhere in vector.c or vector.h)
//--------------------------------------------------------------
void StoreVECTOR(FILE *OUT,const VECTOR *vector,
void (*WriteElement)(FILE *OUT,const void *element,bool *error))
//--------------------------------------------------------------
{
int i;
fwrite(*vector->LB, sizeof(int), 1, OUT);
fwrite(*vector->UB, sizeof(int), 1, OUT);
fwrite(*vector->size, sizeof(int), 1, OUT);
fwrite(vector->elements,vector->size*sizeof(void *),1,OUT);
if ( ferror(OUT) ) RaiseADTException(VECTOR_IO_ERROR);
for (i = vector->LB; i UB; i++)
if ( vector->elements[PHYSICALINDEX(i,vector->LB)] != NOOBJECT )
{
bool error;
WriteElement(OUT,vector->elements[PhysicalIndex(i,vector->LB)],&error);
if ( error ) RaiseADTException(VECTOR_IO_ERROR);
}
}
(2) This is the LoadVECTOR() that needs to be added to the code. (I think somewhere in vector.c or vector.h)
//--------------------------------------------------------------
void LoadVECTOR(FILE *IN,VECTOR *vector,
void (*ReadElement)(FILE *IN,void **element,bool *error))
//--------------------------------------------------------------
{
int i;
DestructVECTOR(vector);
fread(*vector->LB, sizeof(int), 1, IN);
fread(*vector->UB, sizeof(int), 1, IN);
fread(*vector->size, sizeof(int), 1, IN);
vector->elements = (void **) malloc(vector->size*sizeof(void *));
if ( vector->elements == NULL ) RaiseADTException(MALLOC_ERROR);
fread(vector->elements,vector->size*sizeof(void *),1,IN);
if ( ferror(IN) ) RaiseADTException(VECTOR_IO_ERROR);
for (i = vector->LB; i UB; i++)
if ( vector->elements[PhysicalIndex(i,vector->LB)] != NOOBJECT )
{
bool error;
ReadElement(IN,&vector->elements[PhysicalIndex(i,vector->LB)],&error);
if ( error ) RaiseADTException(VECTOR_IO_ERROR);
}
}
//--------------------------------------------------------------
// Generic VECTOR abstract data type
// Vector.c
//--------------------------------------------------------------
#include
#include
#include
#include "Vector.h"
#include "ADTExceptions.h"
#define PHYSICALINDEX(logicalIndex,LB) ((logicalIndex)-(LB))
int PhysicalIndex(const int logicalIndex,const int LB);
//--------------------------------------------------------------
void ConstructVECTOR(VECTOR *vector,const int LB,const int UB,
void (*DestructElement)(const void *element))
//--------------------------------------------------------------
{
int i;
vector->LB = LB;
vector->UB = UB;
if ( !(LB
vector->size = UB-LB+1;
vector->elements = (void **) malloc(vector->size*sizeof(void *));
if ( vector->elements == NULL ) RaiseADTException(MALLOC_ERROR);
for (i = LB; i
vector->elements[PHYSICALINDEX(i,LB)] = NOOBJECT;
vector->DestructElement = DestructElement;
}
//--------------------------------------------------------------
void DestructVECTOR(VECTOR *vector)
//--------------------------------------------------------------
{
if ( vector->DestructElement != NULL )
{
int i;
for (i = vector->LB; i UB; i++)
if ( vector->elements[PhysicalIndex(i,vector->LB)] != NOOBJECT )
vector->DestructElement(vector->elements[PhysicalIndex(i,vector->LB)]);
}
free( vector->elements );
}
//--------------------------------------------------------------
void SetVECTOR(VECTOR *vector,const int index,const void *element)
//--------------------------------------------------------------
{
if ( index LB )
{
int newLB = index;
int newSize = vector->UB-newLB+1;
int i;
void **newElements = (void **) malloc(sizeof(void *)*newSize);
if ( newElements == NULL ) RaiseADTException(MALLOC_ERROR);
for (i = newLB; i UB; i++)
if ( i LB )
newElements[PhysicalIndex(i,newLB)] = NOOBJECT;
else
newElements[PhysicalIndex(i,newLB)] = vector->elements[PhysicalIndex(i,vector->LB)];
vector->LB = newLB;
free(vector->elements);
vector->elements = newElements;
vector->size = newSize;
}
else if ( index > vector->UB )
{
int newUB = index;
int newSize = newUB-vector->LB+1;
int i;
void **newElements = (void **) malloc(sizeof(void *)*newSize);
if ( newElements == NULL ) RaiseADTException(MALLOC_ERROR);
for (i = vector->LB; i
if ( i > vector->UB )
newElements[PhysicalIndex(i,vector->LB)] = NOOBJECT;
else
newElements[PhysicalIndex(i,vector->LB)] = vector->elements[PhysicalIndex(i,vector->LB)];
vector->UB = newUB;
free(vector->elements);
vector->elements = newElements;
vector->size = newSize;
}
if ( (vector->elements[PhysicalIndex(index,vector->LB)] != NOOBJECT) &&
(vector->DestructElement != NULL) )
vector->DestructElement(vector->elements[PhysicalIndex(index,vector->LB)]);
vector->elements[PhysicalIndex(index,vector->LB)] = (void *) element;
}
//--------------------------------------------------------------
void *GetVECTOR(const VECTOR *vector,const int index)
//--------------------------------------------------------------
{
if ( !((vector->LB UB)) ) RaiseADTException(VECTOR_INDEX_ERROR);
return( vector->elements[PhysicalIndex(index,vector->LB)] );
}
//--------------------------------------------------------------
int GetLBVECTOR(const VECTOR *vector)
//--------------------------------------------------------------
{
return( vector->LB );
}
//--------------------------------------------------------------
int GetUBVECTOR(const VECTOR *vector)
//--------------------------------------------------------------
{
return( vector->UB );
}
//--------------------------------------------------------------
int GetSizeVECTOR(const VECTOR *vector)
//--------------------------------------------------------------
{
return( vector->size );
}
//--------------------------------------------------------------
int PhysicalIndex(const int logicalIndex,const int LB)
//--------------------------------------------------------------
{
return( logicalIndex-LB );
}
//--------------------------------------------------------------
// Generic VECTOR abstract data type
// Vector.h
//--------------------------------------------------------------
#ifndef VECTOR_H
#define VECTOR_H
#include
//==============================================================
// Data model definitions
//==============================================================
#define NOOBJECT NULL
typedef struct VECTOR
{
int LB;
int UB;
int size;
void **elements;
void (*DestructElement)(const void *element);
} VECTOR;
//==============================================================
// Public member function prototypes
//==============================================================
void ConstructVECTOR(VECTOR *vector,const int LB,const int UB,
void (*DestructElement)(const void *element));
void DestructVECTOR(VECTOR *vector);
void SetVECTOR(VECTOR *vector,const int index,const void *element);
void *GetVECTOR(const VECTOR *vector,const int index);
int GetLBVECTOR(const VECTOR *vector);
int GetUBVECTOR(const VECTOR *vector);
int GetSizeVECTOR(const VECTOR *vector);
//==============================================================
// Private utility member function prototypes
//==============================================================
//int PhysicalIndex(const int logicalIndex,const int LB);
#endif
//--------------------------------------------------------------
// ADTExceptions.h
//--------------------------------------------------------------
#ifndef ADTEXCEPTIONS_H
#define ADTEXCEPTIONS_H
// ADT exception definitions
#define MALLOC_ERROR "malloc() error"
#define DATE_ERROR "DATE error"
#define STACK_CAPACITY_ERROR "STACK capacity error"
#define STACK_UNDERFLOW "STACK underflow"
#define STACK_OVERFLOW "STACK overflow"
#define STACK_OFFSET_ERROR "STACK offset error"
#define VECTOR_BOUNDS_ERROR "VECTOR bounds error"
#define VECTOR_INDEX_ERROR "VECTOR index error"
#define VECTOR_IO_ERROR "VECTOR IO error"
// ADT exception-handler prototype
void RaiseADTException(char exception[]);
#endif
//--------------------------------------------------------------
// ADTExceptions.c
//--------------------------------------------------------------
#include
#include
#include
#include "ADTExceptions.h"
//--------------------------------------------------------------
void RaiseADTException(char exception[])
//--------------------------------------------------------------
{
fprintf(stderr,"\a Exception \"%s\" ",exception);
system("PAUSE");
exit( 1 );
}
//--------------------------------------------------------------
// VECTOR ADT Problem #2
// Problem2.c
//--------------------------------------------------------------
#include
#include
#include
#include ".\Vector.h"
//--------------------------------------------------------------
int main()
//--------------------------------------------------------------
{
void DestructDouble(const void *element);
void DisplayVector(const VECTOR *vector);
void WriteVectorToDiskFile(const VECTOR *vector);
void ReadVectorFromDiskFile(VECTOR *vector);
int LB,UB;
printf("LB? ");
while ( scanf("%d",&LB) != EOF )
{
VECTOR vector;
int index;
printf("UB? "); scanf("%d",&UB);
ConstructVECTOR(&vector,LB,UB,DestructDouble);
for (index = GetLBVECTOR(&vector); index
{
double *element = (double *) malloc(sizeof(double));
*element = (double) index;
SetVECTOR(&vector,index,element);
}
DisplayVector(&vector);
WriteVectorToDiskFile(&vector);
DestructVECTOR(&vector);
ConstructVECTOR(&vector,LB,UB,DestructDouble);
ReadVectorFromDiskFile(&vector);
DisplayVector(&vector);
DestructVECTOR(&vector);
printf(" LB? ");
}
system("PAUSE");
return( 0 );
}
//--------------------------------------------------------------
void DestructDouble(const void *element)
//--------------------------------------------------------------
{
free((double *) element);
}
//--------------------------------------------------------------
void DisplayVector(const VECTOR *vector)
//--------------------------------------------------------------
{
int index;
printf("LB = %3d, UB = %3d, size = %d ",
GetLBVECTOR(vector),GetUBVECTOR(vector),GetSizeVECTOR(vector));
for (index = GetLBVECTOR(vector); index
if ( GetVECTOR(vector,index) == NOOBJECT )
printf("vector[%3d] = NOOBJECT ",index);
else
printf("vector[%3d] = %lf ",index,*(double *) GetVECTOR(vector,index));
}
//--------------------------------------------------------------
void WriteVectorToDiskFile(const VECTOR *vector)
//--------------------------------------------------------------
{
void WriteDouble(FILE *OUT,const void *element,bool *error);
char fileName[80+1];
FILE *OUT;
do
{
printf("OUT fileName? ");
scanf("%s",fileName);
if ( (OUT = fopen(fileName,"wb")) == NULL ) printf("Unable to open file! ");
} while ( OUT == NULL );
StoreVECTOR(OUT,vector,WriteDouble);
fclose(OUT);
}
//--------------------------------------------------------------
void WriteDouble(FILE *OUT,const void *element,bool *error)
//--------------------------------------------------------------
{
*error = (fwrite(element,sizeof(double),1,OUT) != 1);
}
//--------------------------------------------------------------
void ReadVectorFromDiskFile(VECTOR *vector)
//--------------------------------------------------------------
{
void ReadDouble(FILE *IN,void **element,bool *error);
char fileName[80+1];
FILE *IN;
do
{
printf(" IN fileName? ");
scanf("%s",fileName);
if ( (IN = fopen(fileName,"rb")) == NULL ) printf("Unable to open file! ");
} while ( IN == NULL );
LoadVECTOR(IN,vector,ReadDouble);
fclose( IN);
}
//--------------------------------------------------------------
void ReadDouble(FILE *IN,void **element,bool *error)
//--------------------------------------------------------------
{
*element = (void *) malloc(sizeof(double));
if ( *element == NULL )
*error = true;
else
*error = (fread(*element,sizeof(double),1,IN) != 1);
}
Sample Program Dialo ? ENCOURSESICS1311CodeADTsIVECTORProblem2.exe LB?-2 UB? 2 vector [ -2 ] =-2. 000000 vectorI-11-1.000000 vector 010.G000O0 vector[ 1] 1.000000 vector 21 2.000000 OUT fileName? u1.vector IN fileName? u1.vector LB = -2, UB = 2, size = 5 vector[ -21 -2.000000 vector[ -1] = -1 .000000 ectoF 01 0.00000 vector[ 1] = 1.000000 vector 21 2.0G0000 LB? Z Press any key to continue... _Step by Step Solution
There are 3 Steps involved in it
Step: 1
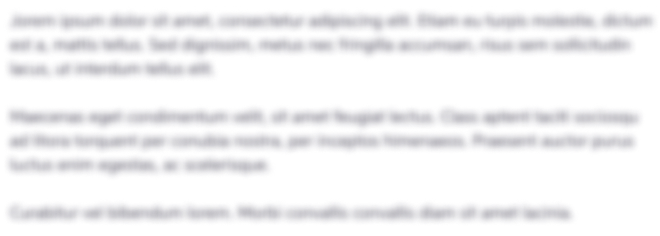
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started