Question
Problems (to be solved on LEGv8 assembly; all code should adhere to standard register, stack use, paremeter passing, etc. conventions, as discussed in lecture and
Problems (to be solved on LEGv8 assembly; all code should adhere to standard register, stack use, paremeter passing, etc. conventions, as discussed in lecture and the textbook):
* Implement a swap procedure that swaps the values in two different 8-byte integers in memory. * Implement a find smallest procedure that finds the smallest 8-byte integer in an array and returns its index. * Implement selection sort using your find smallest and swap procedures. To be clear, selection sort can be more efficiently implemented without any helper procedures, but implementing it in assembly is easier with the helpers--and it gives you experience with procedure calls and stack manipulation--so we are requiring that you do it this way.
Pseudocode for the selection sort:
SelectionSort(array) for each element in array in order from first to last: swap element with smallest element in the subarray that it begins
* Implement a procedure to fill an array with consecutive 8-byte integers in reverse-sorted (high to low) order * Implement a "main" procedure that ties all of this together by: ** Calling your fill procedure to create a reverse sorted array in main memory. ** Sorting that array using your selection sort implementation ** Program ends with a DUMP
Step by Step Solution
There are 3 Steps involved in it
Step: 1
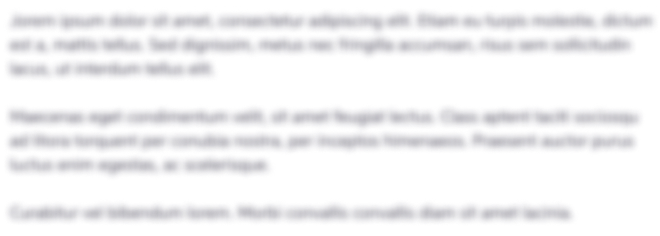
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started