Question
Program Description A local bike share company wants a program that will help them track their daily bike rentals. Use the following instructions to code
Program Description
A local bike share company wants a program that will help them track their daily bike rentals. Use the following instructions to code the program:
File 1 - BikeRental.java
- Write a BikeRental class that will hold fields for the following:
- The bikes ID (example: RB1234)
- The full name (first and last) of the person that rented the bike
- The age of the person that rented the bike
- The number of miles that the bike was ridden
- The BikeRental class should also contain the following methods:
- A constructor that doesnt accept arguments.
- A constructor that accepts arguments for each field.
- Appropriate accessor and mutator methods (i.e., setters and getters).
- A method named getRentalPrice that accepts no arguments and calculates and returns the price of the bike rental based on the following information:
There is a base fee of $2.50 to rent the bike, plus an additional fee based on the riders age and how far the bike is ridden. The additional fee can be calculated as below:
Riders Age | Miles Ridden | Cost Per Mile |
Under 18 | Up to 10 (inclusive) | $0.25 |
More than 10 | $0.20 | |
18 or over | Up to 10 (inclusive) | $0.40 |
More than 10 | $0.35 |
File 2 - BikeRentalDemo.java
- Write a program that demonstrates the class and calculates the cost of bike rentals. The program should ask the user for the following:
- The bikes ID
- The renters full name
- The renters age
- The miles ridden by the renter
- All numerical input should be validated. The program should not accept a number less than 0 for the renters age or the number of miles ridden.
- The program should prompt the user for all the data necessary to fully initialize the object.
- The program should create the objects using the constructor that does not accept arguments.
- The program should continue allowing the user to input information until the user indicates to stop (see Sample Input and Output).
- The program should keep track of the number of bike rentals that were processed while it is running and output that number at the end of the program.
- The program should display the input and output exactly as shown in the provided sample output (Note: this includes blank lines, decimal formatting, user input on the same line as the prompt).
Sample Input and Output (formatting and spacing should be exactly as shown below)
Bike Rental Tracking Service
Enter bikes ID: RB1234
Enter the name of the person renting the bike: John Doe
Enter the age of the person renting the bike: 24
Enter the number of miles that the bike was ridden: 11.2
Bike ID: RB1234
Renter Name: John Doe
Renter Age: 24
Miles Ridden: 11.2
Cost of Rental: $6.42
Do you wish to enter information for another bike rental (Y/N)? Y
Enter bikes ID: RB5678
Enter the name of the person renting the bike: Jane Doe
Enter the age of the person renting the bike: 16
Enter the number of miles that the bike was ridden: 4.75
Bike ID: RB5678
Renter Name: Jane Doe
Renter Age: 16
Miles Ridden: 4.75
Cost of Rental: $3.69
Do you wish to enter information for another bike rental (Y/N)? Y
Enter bikes ID: RB4321
Enter the name of the person renting the bike: Jack Doe
Enter the age of the person renting the bike: 57
Enter the number of miles that the bike was ridden: 2.1
Bike ID: RB4321
Renter Name: Jack Doe
Renter Age: 57
Miles Ridden: 2.1
Cost of Rental: $3.34
Do you wish to enter information for another bike rental (Y/N)? N
Total number of bike rentals processed: 3
Step by Step Solution
There are 3 Steps involved in it
Step: 1
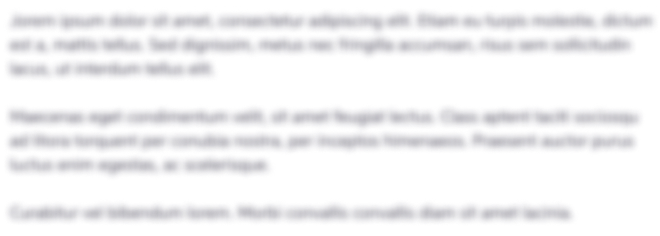
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started