Question
Program Using C. Write a program that reads file cylinders.txt and sort the cylinders by volume. Output the sorted cylinders, including volumes in a text
Program Using C.
Write a program that reads file cylinders.txt and sort the cylinders by volume. Output the sorted cylinders, including volumes in a text file called sorted_cylinders.txt. A cylinder has the following attributes:
1. radius inches double
2. height inches double
3. Weight pounds double
You can assume the file as the following format for each cylinder.
2.5, 12.2, 23.4
1. Name your program cylinders.c.
2. The program should be built around an array of structures, with each structure containing information of a cylinders radius, height, weight, and volume. Assume that there are no more than 1000 cylinders in the file.
3. Use fscanf and fprintf to read and write data.
4. Modify the selection_sort function provided to sort an array of product struct. The cylinders should be sorted by volume in ascending order. The function should have the following prototype:
void selection_sort(struct cylinder my_cylinders[], int n);
5. Output the sorted cylinders, including volume, in a text file called sorted_cylinders.txt, in the following format.
# radius Height Volume Weight
0 3.500000 2.500000 96.211275 15.800000
1 6.000000 1.000000 113.097336 2.9000002
2 2.400000 12.000000 217.146884 15.000000
3 21.000000 1.000000 1385.442360 100.000000
4 18.200000 14.200000 14776.820338 25.900000
5 22.800000 10.600000 17311.130565 4.500000
Suggestions:
1. Set up cylinder struct.
2. Use fscanf function to read the input file (note that fscanf returns number of entries filled).
3. Initially output unsorted array to screen to make sure the file input and array setup are working.
4. Modify the selection_sort function for processing cylinders.
5. Initially output sorted array to the screen.
6. When output is correct, write to the output file.
selection_sort.c
#include
#define N 10
voidselection_sort(int a[], int n);
int main(void)
{
int i;
int a[N];
printf("Enter %d numbers to be sorted: ", N);
for (i = 0; i < N; i++)
scanf("%d", &a[i]);
selection_sort(a, N);
printf("In sorted order:");
for (i = 0; i < N; i++)
printf(" %d", a[i]);
printf(" ");
return 0;
}
void selection_sort(int a[], int n)
{
int i, largest = 0, temp;
if (n == 1)
return;
for (i = 1; i < n; i++)
if (a[i] > a[largest])
largest = i;
if (largest < n - 1) {
temp = a[n-1];
a[n-1] = a[largest];
a[largest] = temp;
}
selection_sort(a, n - 1);
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
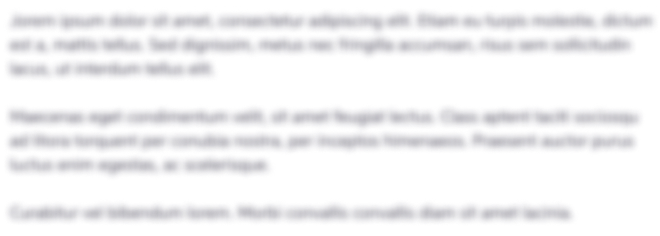
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started