Question
== Programming Assignment == For this assignment you will write a program that reads in climate data (imagine it is coming from a weather station)
== Programming Assignment ==
For this assignment you will write a program that reads in climate data (imagine it is coming from a weather station) and stores that data in a linked list. After reading and storing all of the data your program will generate a report from the data.
The objective of this assignment is to learn how to implement a linked list and to gain familiarity with using a linked list in solving a problem. Since one of the objectives is to learn how to implement a linked list you cannot use the STL linked list.
Your program should use good design methodologies so you should have separate classes for each of the following:
- datalogger -- This class represents the "business logic" of adding data to the storage. This class also handles filtering out duplicate data items (see the requirements listed below). This is the only class that weatherlog.cpp knows about and it expects two methods: addData() and printReport(). - linkedlist -- This class should implement a linked list. This class should be distinct from the datalogger class (although I would expect datalogger to have a data member that is a linkedlist object). - weatherdata -- This class encapsulates the weather data (time, temperature, windspeed).
You are welcome to create any additional classes that you need.
Below are the specific external and internal requirements for this assignment. Your program will be evaluated based on these requirements.
== External Requirements ==
- The main driver (weatherlog.cpp) will provide a sequence of data readings. The program needs to store these readings. After all of the data is read in, the program must create a report identical to the one in the expected.txt output file. - Data with a duplicate timestamp indicates contradictory or inaccurate data and should not be stored (all records with the same timestamp). - The report includes: - a line that states the timestamp range - a line that states the number of data entries - a temperature section that contains a detailed report (see below) - a windspeed section that contains a detailed report (see below) - detailed report data: - the minumum value measured - the maximum value measured - a list of all of the readings >= the 99% reading (in order) - a list of all of the readings <= the 1% reading (in order) - see the provided "expected.txt" to see the report format
== Internal Requirements ==
- The program must use the supplied weatherlog.cpp file, unmodified, as the main driver. - All of the weather data must be stored in a linked list. - The linked list must have three separate "chains". One that keeps the data ordered by timestamp, one that keeps the data ordered by temperature, and one that keeps the data ordered by windspeed. - Strings must be stored using char* variables, not std::string. - No memory leaks.
weatherlog.cpp file
#include
using namespace std;
int main(int argc, char** argv) {
datalogger dl;
if (argc != 2) { cout << "Usage: " << argv[0] << "
// Read the data
char* datafile = argv[1]; ifstream infile(datafile); int timestamp; double temperature; double windspeed;
while (!infile.eof()) { infile >> timestamp; infile >> temperature; infile >> windspeed;
if (!infile.eof()) { dl.addData(timestamp, temperature, windspeed); } }
// Output the report dl.printReport();
return(0);
Some example climate data values in climate.txt:
1480911409 -132 565 1480911412 -132 603 1480911416 -134 533 1480911418 -137 556 1480911418 -138 515 1480911425 -139 614 1480911430 -143 708 1480911435 -143 659 1480911443 -140 705 1480911443 -139 773 1480911452 -138 676 1480911452 -134 655 1480911453 -134 673 1480911454 -130 584 1480911459 -130 616 1480911463 -126 615 1480911468 -126 610 1480911469 -126 677 1480911472 -122 705 1480911473 -121 644 1480911482 -121 691 1480911487 -118 747 1480911493 -114 650 1480911497 -112 672 1480911503 -108 654 1480911503 -107 576 1480911504 -104 585 1480911512 -104 614 1480911518 -104 554 1480911522 -103 485 1480911527 -100 399 1480911529 -97 476 1480911529 -93 539 1480911532 -93 489 1480911537 -90 504 1480911537 -86 420 1480911541 -84 376 1480911544 -83 402 1480911546 -81 435 1480911551 -78 455 1480911557 -75 465 1480911557 -73 520
Code should be in C++
Step by Step Solution
There are 3 Steps involved in it
Step: 1
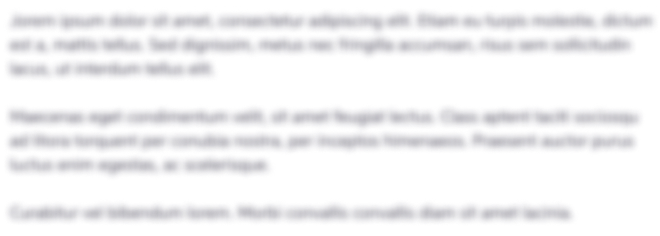
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started