programming in c:
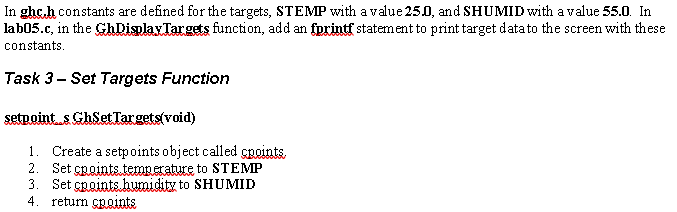
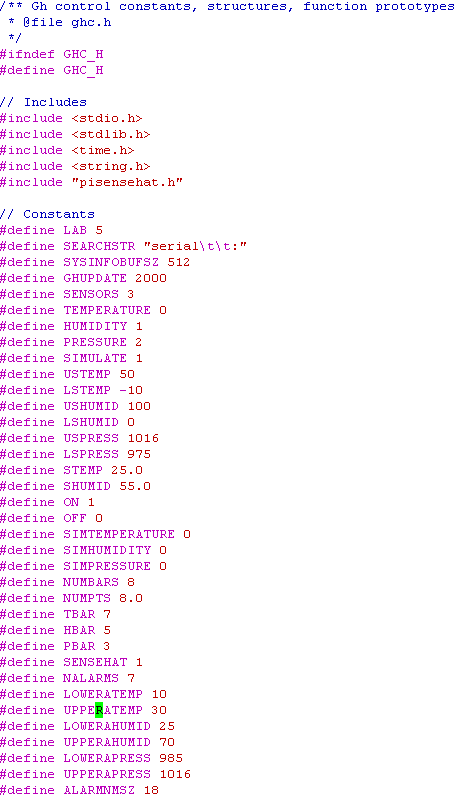
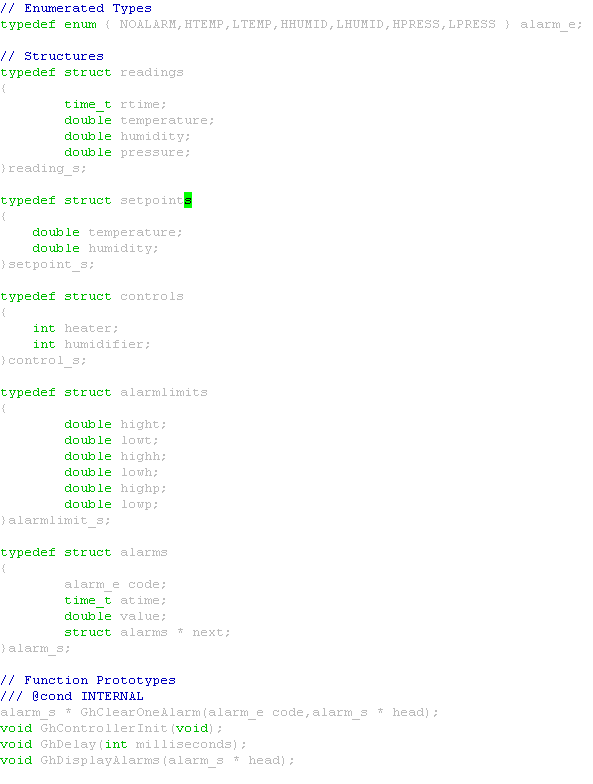
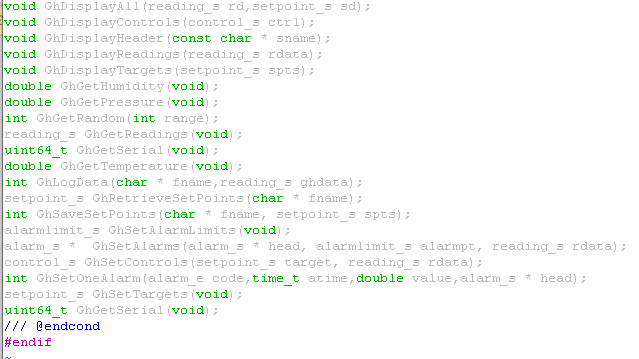
/** Gh Control functions
* @ file lab05.c
*/
# include "ghc.h"
/** Displays targets
* param spts setpoint_s spts
*@return void
*/
void GhDisplayTargets(setpoint_s spts)
{
}
/** Set targets
*@param void
*@ return setpoint_s structure with temperature,humidty targets
*/
setpoint_s GhSetTargets(void)
{
}
In ghch constants are defined for the targets, STEMP with a value 250, and SHUMID with a value 55.0. In lab05.c, in the GhDisplay Targets function, add an fprintf statement to print target data to the screen with these constants. Task 3 - Set Targets Function setpoint.s GhSetTargets(void) 1. Create a setpoints object called cpoints. 2. Set cpoints.temperature to STEMP 3. Set cpoints humidity to SHUMID 4. return cpoints ** Gh control constants, structures, function prototypes * file ghc.h #ifndef GHC_H #define GHC_H // Includes #include
#include #include #include #include "pisensehat.h" // Constants #define LAB 5 #define SEARCHSTR "serial\t\t:" #define SYS INFOBUFSZ 512 #define GHUPDATE 2000 #define SENSORS 3 #define TEMPERATURE O #define HUMIDITY 1 #define PRESSURE 2 #define SIMULATE 1 #define USTEMP 50 #define LSTEMP -10 #define USHUMID 100 #define LSHUMID O #define USPRESS 1016 #define LSPRESS 975 #define STEMP 25.0 #define SHUMID 55.0 #define ON 1 #define OFF O #define SIMTEMPERATURE O #define SIMHUMIDITY O #define SIMPRESSURE O #define NUMBARS 8 #define NUMPTS 8.0 #define TBAR 7 #define HBAR 5 #define PBAR 3 #define SENSEHAT 1 #define NALARMS 7 #define LOWERTEMP 10 #define UPPERTEMP 30 #define LOWERAHUMID 25 #define UPPERAHUMID 70 #define LOWERAPRESS 985 #define UPPERAPRESS 1016 #define ALARMNMSZ 18 // Enumerated Types typedef enum { NOALARM, HTEMP, LTEMP, HHUMID, LHUMID, HPRESS, LPRESS } alarm_e; // Structures typedef struct readings time_t rtime; double temperature; double humidity: double pressure; }reading_s; typedef struct setpoint double temperature; double humidity: } set point_s; typedef struct controls int heater: int humidifier; } control si typedef struct alarmlimits double hight; double lout; double highh; double lowh; double highp: double low: } alarmlimit_s; typedef struct alarms alarme code; time_t atime; double value; struct alarms * next; } alarm_s; // Function Prototypes /// @cond INTERNAL alarm 3 * GhClearonellarmialarm_e code, alarm_3 * head); void GhController Init (void); void GhDelay (int milliseconds); void GhDisplay,larms ( alarm s * head); void GhDisplayill(reading_3 rd, setpoint_3 sd); void GhDisplayControls (control_s ctrl); void GhDisplayHeader (const char * sname); void GhDisplayReadings (reading_3 rdata); void GhDisplayTargets (setpoint_3 spts); double GhGet Humidity (void); double GhGet Pressure (void); int GhGetRandom(int range); reading_s GhGet Readings (void); uint64_t GhGetSerial(void); double GhGet Temperature (void); int GhLogData(char * fname, reading_s ghdata); setpoint_s GhRetrieveSet Points (char * fname); int GhSaveSetPoints (char * fname, setpoint_s spts); alarmlimit 3 GhSetilarmLimits (void); alarm s * GhSetilarms (alarm 3 * head, alarmlimit_s alarmpt, reading_s rdata); control_s GhSetControls (setpoint_s target, reading_3 rdata); int GhSetOnedlarm(alarm_e code, time_t atime, double value, alarm s * head); setpoint_s GhSet Targets (void); uint64_t GhGetSerial(void); /// Bendcond #endif In ghch constants are defined for the targets, STEMP with a value 250, and SHUMID with a value 55.0. In lab05.c, in the GhDisplay Targets function, add an fprintf statement to print target data to the screen with these constants. Task 3 - Set Targets Function setpoint.s GhSetTargets(void) 1. Create a setpoints object called cpoints. 2. Set cpoints.temperature to STEMP 3. Set cpoints humidity to SHUMID 4. return cpoints ** Gh control constants, structures, function prototypes * file ghc.h #ifndef GHC_H #define GHC_H // Includes #include #include #include #include #include "pisensehat.h" // Constants #define LAB 5 #define SEARCHSTR "serial\t\t:" #define SYS INFOBUFSZ 512 #define GHUPDATE 2000 #define SENSORS 3 #define TEMPERATURE O #define HUMIDITY 1 #define PRESSURE 2 #define SIMULATE 1 #define USTEMP 50 #define LSTEMP -10 #define USHUMID 100 #define LSHUMID O #define USPRESS 1016 #define LSPRESS 975 #define STEMP 25.0 #define SHUMID 55.0 #define ON 1 #define OFF O #define SIMTEMPERATURE O #define SIMHUMIDITY O #define SIMPRESSURE O #define NUMBARS 8 #define NUMPTS 8.0 #define TBAR 7 #define HBAR 5 #define PBAR 3 #define SENSEHAT 1 #define NALARMS 7 #define LOWERTEMP 10 #define UPPERTEMP 30 #define LOWERAHUMID 25 #define UPPERAHUMID 70 #define LOWERAPRESS 985 #define UPPERAPRESS 1016 #define ALARMNMSZ 18 // Enumerated Types typedef enum { NOALARM, HTEMP, LTEMP, HHUMID, LHUMID, HPRESS, LPRESS } alarm_e; // Structures typedef struct readings time_t rtime; double temperature; double humidity: double pressure; }reading_s; typedef struct setpoint double temperature; double humidity: } set point_s; typedef struct controls int heater: int humidifier; } control si typedef struct alarmlimits double hight; double lout; double highh; double lowh; double highp: double low: } alarmlimit_s; typedef struct alarms alarme code; time_t atime; double value; struct alarms * next; } alarm_s; // Function Prototypes /// @cond INTERNAL alarm 3 * GhClearonellarmialarm_e code, alarm_3 * head); void GhController Init (void); void GhDelay (int milliseconds); void GhDisplay,larms ( alarm s * head); void GhDisplayill(reading_3 rd, setpoint_3 sd); void GhDisplayControls (control_s ctrl); void GhDisplayHeader (const char * sname); void GhDisplayReadings (reading_3 rdata); void GhDisplayTargets (setpoint_3 spts); double GhGet Humidity (void); double GhGet Pressure (void); int GhGetRandom(int range); reading_s GhGet Readings (void); uint64_t GhGetSerial(void); double GhGet Temperature (void); int GhLogData(char * fname, reading_s ghdata); setpoint_s GhRetrieveSet Points (char * fname); int GhSaveSetPoints (char * fname, setpoint_s spts); alarmlimit 3 GhSetilarmLimits (void); alarm s * GhSetilarms (alarm 3 * head, alarmlimit_s alarmpt, reading_s rdata); control_s GhSetControls (setpoint_s target, reading_3 rdata); int GhSetOnedlarm(alarm_e code, time_t atime, double value, alarm s * head); setpoint_s GhSet Targets (void); uint64_t GhGetSerial(void); /// Bendcond #endif