Question
**PROGRAMMING QUESTION: MYPROGRAMMINGLAB CHAPTER 10 PROGRAMMING PROJECT 71124** Design a class named Person with fields for holding a person's name, address, and telephone number (all
**PROGRAMMING QUESTION: MYPROGRAMMINGLAB CHAPTER 10 PROGRAMMING PROJECT 71124**
Design a class named Person with fields for holding a person's name, address, and telephone number (all as Strings). Write a constructor that initializes all of these values, and mutator and accessor methods for every field. Next, design a class named Customer, which inherits from the Person class. The Customer class should have a String field for the customer number and a boolean field indicating whether the customer wishes to be on a mailing list. Write a constructor that initializes these values and the appropriate mutator and accessor methods for the class's fields. Demonstrate the Customer class in a program that prompts the user to enter values for the customer's name, address, phone number, and customer number, and then asks the user whether or not the customer wants to recieve mail. Use this information to create a customer object and then print its information. Put all of your classes in the same file. To do this, do not declare them public. Instead, simply write: class Person { ... } class Customer { ... }
The code I use is below but I get the following error in myProgramming lab when I submit it:
Driver.java:221: error: class Person_and_Customer is public, should be declared in a file named Person_and_Customer.java public class Person_and_Customer ^ 1 error
//Include the header file.
import java.util.Scanner;
//Define the class Person.
class Person
{
//Declare the variables.
String p_name, p_address, p_phone;
//Define the constructor.
public Person(String name,
String addr, String phone)
{
this.p_name = name;
this.p_address = addr;
this.p_phone = phone;
}//End of the constructor.
//The getter method for name.
public String get_pName()
{
//Return the person name.
return p_name;
}//End of the method getName.
//The setter method for name.
public void set_pName(String name)
{
//Set the name.
this.p_name = name;
}//End of the method set_pName.
//The getter method for address.
public String get_pAddress()
{
//Return the address.
return p_address;
}//End of the method set_pName.
//The setter method for address.
public void set_pAddress(String address)
{
//Set the address.
this.p_address = address;
}//End of the method set_pAddress.
//The getter method for phone.
public String get_pPhone()
{
//Return the phone number.
return p_phone;
}//End of the method get_pPhone.
//The setter method for phone.
public void set_pPhone(String phone)
{
//Set the number.
this.p_phone = phone;
}//End of the method set_pPhone.
}//End of the class Person.
//Define the class customer
//that extends Person class.
class Customer extends Person
{
//Declare the variables.
String customer_phone_number;
boolean mail_list;
//Define the constructor.
public Customer(String name, String addr,
String phone, String number,
boolean mail)
{
//Call the super method.
super(name, addr, phone);
this.customer_phone_number = number;
this.mail_list = mail;
}//End of the constructor.
//Define the getter method.
public String getCustomerNumber()
{
//Return the phone number
//of customer.
return customer_phone_number;
}//End of the method.
//Define the setter method.
public void setCustomerNumber
(String Number)
{
//Set the number.
this.customer_phone_number = Number;
}//End of the method.
//Define the function.
public boolean isMail()
{
//Return the list.
return mail_list;
}//End of the method.
//Define the getter method.
public void setMail(boolean mail_entered)
{
//Set the mail.
this.mail_list = mail_entered;
}//End of the method.
//Define the function.
public String toString()
{
//Return the information
//of the customer.
return "Name: " + get_pName()
+" Address: "+ get_pAddress()
+" Phone Number:" + get_pPhone()
+" Customer Number:"+
customer_phone_number + get_pPhone() +
" Received Mail?: " + mail_list;
}//End of the function toString().
}//End of the class Customer.
//Define the class.
public class Person_and_Customer
{
public static void main(String args[])
{
//Create an object of scanner type.
Scanner sc = new Scanner(System.in);
//Prompt the user to
//enter the information.
System.out.println("Enter name of "
+ "customer: ");
String name = sc.nextLine();
System.out.println("Enter address"
+ " of customer: ");
String address = sc.nextLine();
System.out.println("Enter phone number"
+ " of customer: ");
String phone = sc.nextLine();
System.out.println("Enter customer number: ");
String customerNumber = sc.nextLine();
//Prompt the user to enter the choice.
System.out.println("Enter yes/no -- does the"
+ " customer want to recieve mail?: ");
String input = sc.nextLine();
if(input.equals("no"))
{
//Define the variable.
boolean mail_list = false;
//Display the statement.
System.out.println(" Customer:");
//Display the information.
Customer cust = new Customer(name, address,
phone, customerNumber, mail_list);
System.out.println(cust);
}//End of if part.
//End of else part.
else
{
//Define the variable.
boolean mail_list = true;
//Display the statement.
System.out.println(" Customer:");
//Display the information.
Customer cust = new Customer(name, address,
phone, customerNumber, mail_list);
System.out.println(cust);
}//End of else part.
}//End of the main() function.
}//End of the class Person_and_Customer.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
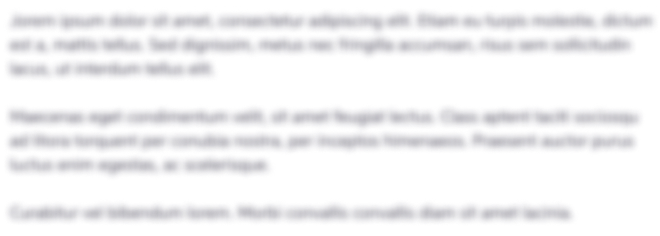
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started