Question
Project 2 Project results in one program to be turned in online. Must be javadocd completely. Problem Description Write a program to assign passengers seats
Project 2
Project results in one program to be turned in online. Must be javadocd completely.
Problem Description
Write a program to assign passengers seats in a small airplane.
You only need to deal with this configuration airplane:
ROW
1 A B C D
2 A B C D
3 A B C D
4 A B C D
5 A B C D
6 A B C D
7 A B C D
You MUST use a 2-dimensional array. The program should update to show an X when the seat is taken. For example, after 1A, 2C, 7B is taken - this is what should be displayed:
ROW
1 X B C D
2 A B X D
3 A B C D
4 A B C D
5 A B C D
6 A B C D
7 A X C D
After displaying three seats available, prompt the user to enter their desired seat. And then print out an updated display of available seats. The program continues until the user is done or all the seats have been assigned. If the user enters a seat already assigned, the program should say that is seat is occupied and enter a new seat selection.
Hints - based on my experience, do however you want
-
I used a String[][] array - you could use char if you want.
-
When I asked them to select the seat - I separated the entry- like 1C - into a 1 and a C and then used a switch to convert the 1 to the correct integer I could use as a row index and then another switch where I took the C and converted it to an integer to use for the column index.
-
Remember when you are checking for equality with string - even an entry in a string array you have to use the equals method
-
Remember that in java if you pass an array to a method- and that method updates the array- the array does not have to be returned to the main method. When the main method uses the array again, it will see the update the method did. This is because arrays are Pass by Reference - you dont actually copy the array and pass the the method- you pass the memory address where the array is stored to the the method so the method is updating the actual array
-
Write a method to print out the seatSelection array - I made mine a void method
-
I wrote a method for updateSeat I passed the seatSelection array to and it returned a boolean if the update worked. I prompt the user for the String seat I the method
Here a sample from my program
1 A B C D
2 A B C D
3 A B C D
4 A B C D
5 A B C D
6 A B C D
7 A B C D
After I forced some updates to make testing easier
1 A X C D
2 A X X D
3 A B C D
4 A B C D
5 A X C D
6 A B X D
7 A B C D
Give row then seat- no spaces
3A
1 A X C D
2 A X X D
3 X B C D
4 A B C D
5 A X C D
6 A B X D
7 A B C D
Give row then seat- no spaces
4D
1 A X C D
2 A X X D
3 X B C D
4 A B C X
5 A X C D
6 A B X D
7 A B C D
Give row then seat- no spaces
6C
Seat taken look again
1 A X C D
2 A X X D
3 X B C D
4 A B C X
5 A X C D
6 A B X D
7 A B C D
Give row then seat- no spaces
continues until plane full
Step by Step Solution
There are 3 Steps involved in it
Step: 1
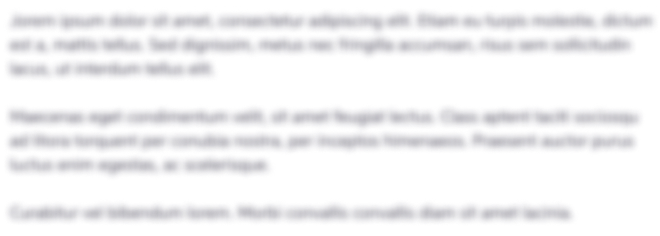
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started