Question
project 5 down written. .............................................................. package bank; //Customer class public class Customer { private String firstName; private String lastName; private String ssn; //Constructor Customer(){ }
project 5 down written.
..............................................................
package bank;
//Customer class public class Customer { private String firstName; private String lastName; private String ssn;
//Constructor Customer(){ }
//setter and getter method public String getFirstName() { return firstName; } public void setFirstName(String firstName) { this.firstName = firstName; } public String getLastName() { return lastName; } public void setLastName(String lastName) { this.lastName = lastName; } public String getSsn() { return ssn; } public void setSsn(String ssn) { if((ssn.charAt(3) != '-')||(ssn.charAt(6) != '-')|| ssn.length()!=11) System.out.println("Invalid SSN."); else this.ssn = ssn; } }
///////////////////////////////
package bank;
//BankAccount class public abstract class BankAccount {
protected float balance; protected Customer customer; protected long accountNumber; public float getBalance() { return balance; }
public void setBalance(float balance) { this.balance = balance; }
public Customer getCustomer() { return customer; }
public void setCustomer(Customer customer) { this.customer = customer; }
public long getAccountNumber() { return accountNumber; }
public void setAccountNumber(long accountNumber) { this.accountNumber = accountNumber; }
//Constructors BankAccount (){ }
BankAccount(String firstName, String lastName, String ssn,float balance) { customer = new Customer(); customer.setFirstName(firstName); customer.setLastName(lastName); customer.setSsn(ssn); this.balance = balance; }
public void deposit(float amount) { balance = balance + amount; System.out.println(customer.getFirstName() + " " + customer.getLastName() + " deposited $" + amount + ". Current Balance $" + balance);
}
public void withdraw(float amount) { if (balance >= amount) { balance = balance - amount; System.out.println(customer.getFirstName() + " " + customer.getLastName() + " withdrew $" + amount + ". Current Balance $" + balance); } if (balance
}
//////////////////////
package bank;
//SavingAccount class public class SavingAccount extends BankAccount {
public SavingAccount() { }
public SavingAccount(String firstName, String lastName, String ssn, float balance) { super(firstName, lastName, ssn, balance); System.out.println("Successfully created account for " + firstName + " " + lastName + " " + accountNumber); System.out.println(firstName + " " + lastName + ", Balance $" + balance); }
public float applyInterest () { balance += ((balance - 10000) * 5)/100; return balance; }
long accountNumber() { long accountNumber = (long) Math.floor(Math.random() * 9000000000L) + 1000000000L; return accountNumber; }
float checkBalance() { System.out.println(customer.getFirstName() + " " + customer.getLastName() + ", Balance $" + balance); return balance; } }
/////////////////////////////
package bank;
//CheckingAccount class public class CheckingAccount extends BankAccount {
public CheckingAccount() { }
public CheckingAccount(String firstName, String lastName, String ssn, float balance) { super(firstName, lastName, ssn, balance); System.out.println("Successfully created account for " + firstName + " " + lastName + " " + accountNumber()); }
public float applyInterest () { balance += ((balance - 10000)*2)/100; return balance; }
float checkBalance() {
System.out.println(customer.getFirstName() + " " + customer.getLastName() + ", Balance $" + balance);
return balance; }
long accountNumber() { long accountNumber = (long) Math.floor(Math.random() * 9000000000L) + 1000000000L; return accountNumber; }
......................................................................................................................................................................
project 6
Added compareTo() method in BankAccount.java file for sorting list of accounts on balance. Added new java file BankDatabase.java. Tested output files with exact format are also attached.
package com.mycompany.project6;
import java.util.*;
public class BankDatabase { ArrayList
String names[] = customerName.split(" "); String firstName = names[0]; String lastName = names[1]; // create new instance of CheckingAccount SavingAccount account = new SavingAccount(firstName, lastName, ssn, deposit); bankAccounts.add(account); } public void print(){ //Sort the bank accounts on price in ascending order and print to console Collections.sort(bankAccounts); for (BankAccount account: bankAccounts) { // Prints details of each bank account as per the format System.out.println(account.getCustomer().getFirstName() + " " + account.getCustomer().getLastName() + ", accn #: " + account.getAccountNumber() + ", Balance $" + account.getBalance()); } } public void applyInterest(){ for (BankAccount account: bankAccounts) { account.applyInterest(); } } }
SAVINGS ACCOUNT .JAVA
package com.mycompany.project6;
public class SavingAccount extends BankAccount { public SavingAccount() { } public SavingAccount(String firstName, String lastName, String ssn, float balance) { super(firstName, lastName, ssn, balance); this.accountNumber = accountNumber(); System.out.println("Successfully created account for " + firstName + " " + lastName + " Account Number " + this.accountNumber); } public float applyInterest () { balance += ((balance - 10000) * 5)/100; return balance; } long accountNumber() { long accountNumber = (long) Math.floor(Math.random() * 9000000000L) + 1000000000L; return accountNumber; } float checkBalance() { System.out.println(customer.getFirstName() + " " + customer.getLastName() + ", Balance $" + balance); return balance; } }
CHECKING ACCOUNT .JAVA
package com.mycompany.project6;
public class CheckingAccount extends BankAccount { public CheckingAccount() { } public CheckingAccount(String firstName, String lastName, String ssn, float balance) { super(firstName, lastName, ssn, balance); this.accountNumber = accountNumber(); System.out.println("Successfully created account for " + firstName + " " + lastName + " Account Number " + this.accountNumber); } public float applyInterest () { balance += ((balance - 10000)*2)/100; return balance; } float checkBalance() { System.out.println(customer.getFirstName() + " " + customer.getLastName() + ", Balance $" + balance); return balance; }
long accountNumber() { long accountNumber = (long) Math.floor(Math.random() * 9000000000L) + 1000000000L; return accountNumber; } @Override public int compareTo(BankAccount account) { return Math.round((super.balance - account.balance)); } }
BANKAPP.JAVA
package com.mycompany.project6;
public class BankApp { public static void main(String args[]){ BankDatabase acctDatabase = new BankDatabase(); acctDatabase.createCheckingAccount("Alin Parker", "123-45-6789", 20000.0f); acctDatabase.createSavingAccount("Mary Jones", "987-65-4321", 15000.0f); acctDatabase.createSavingAccount("John Smith", "123-45-6789", 12000.0f); acctDatabase.print(); acctDatabase.applyInterest(); acctDatabase.print(); } }
BANKACCOUNT.jAVA
package com.mycompany.project6;
public abstract class BankAccount implements Comparable
//Constructors BankAccount (){ } BankAccount(String firstName, String lastName, String ssn,float balance) { customer = new Customer(); customer.setFirstName(firstName); customer.setLastName(lastName); customer.setSsn(ssn); this.balance = balance; } public void deposit(float amount) { balance = balance + amount; System.out.println(customer.getFirstName() + " " + customer.getLastName() + " deposited $" + amount + ". Current Balance $" + balance); } public void withdraw(float amount) { if (balance >= amount) { balance = balance - amount; System.out.println(customer.getFirstName() + " " + customer.getLastName() + " withdrew $" + amount + ". Current Balance $" + balance); } if (balance
// Sorts Bankaccounts in ascending order based on balance public int compareTo(BankAccount account) { return Math.round((this.balance - account.balance)); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
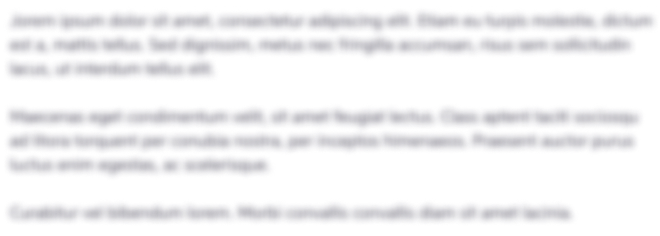
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started