Question
Project 7 Change the program to use structs for temperature, wind, and weather measurement. Refactor your program so that the code for - temperature is
Project 7
Change the program to use structs for temperature, wind, and weather measurement.
Refactor your program so that the code for
- temperature is in two files (.h declarations) and (.cpp implementations)
- wind is in two files (.h declarations) and (.cpp implementations)
-WeatherMeasurement is in two files (.h declarations) and (.cpp implementations)
- And your main is in one file
C++ code:
#include "stdafx.h"
#include
#include
using namespace std;
void moveTemperaturesToRight(double temperatures[],
double windSpeed[],
string windDirection[])
{
for (int i = 3; i > 0; i--)
{
temperatures[i] = temperatures[i - 1];
windSpeed[i] = windSpeed[i - 1];
windDirection[i] = windDirection[i - 1];
}
}
int main()
{
string name;
int choice;
int numOfReadings = 0;
int size;
double temperatures[4], windSpeeds[4];
string windDirections[4];
bool initialized = false;
char str;
//Have the user provide a name for the weather station upon entry.
cout << "Enter the name of weather station: ";
getline(cin, name);
//Control loop to perform various actions.
while (true)
{
cout << "I. Input a complete weather reading." << " ";
cout << "P. Print the current weather." << " ";
cout << "H. Print the weather history (from most recent to oldest)." << " ";
cout << "E. Exit the program." << " ";
cout << "Enter your choice: ";
cin >> str;
if (str != 'I'&& str != 'P'&& str != 'H'&& str != 'E')
choice = 0;
else
choice = str;
//Switch based on choice.
switch (choice)
{
case 'I':
moveTemperaturesToRight(temperatures,
windSpeeds,
windDirections);
cout << "Enter the temperature:";
cin >> temperatures[0];
//get correct wind speed
do
{
cout << "Enter the wind speed (a value >=0):";
cin >> windSpeeds[0];
while (cin.fail() || (cin.peek() != ' ' && cin.peek() != ' '))
{
cout << "Invalid Input! Re Enter the wind speed" << endl;
cin.clear();
while (cin.get() != ' ');
cin >> windSpeeds[0];
}
} while (windSpeeds[0] < 0);
//get correct wind direction
do
{
cout << "Enter the wind direction (North,South,East or West):";
cin >> windDirections[0];
} while (windDirections[0] != "North" && windDirections[0] != "South" && windDirections[0] != "East" && windDirections[0] != "West");
initialized = true;
if (initialized)
numOfReadings++;
if (numOfReadings > 4)
numOfReadings = 4;
break;
case 'H': //Print the current weather, if valid weather is entered.
cout << "Enter size of the history wants:";
cin >> size;
if (numOfReadings { cout << "History size is high"; break; } for (int i = 0; i < size; i++) { cout << "*****" << name << "*****" << " "; cout << "Temperature: " << temperatures[i] << " "; cout << "Wind speed: " << windSpeeds[i] << " "; cout << "Wind direction: " << windDirections[i] << " " << " "; } if (numOfReadings == 0) cout << "Please enter the details before asking to print." << " "; break; case 'P': if (numOfReadings == 0) { cout << "Please enter the details before asking to print." << " "; break; } cout << "*****" << name << "*****" << " "; cout << "Temperature: " << temperatures[0] << " "; cout << "Wind speed: " << windSpeeds[0] << " "; cout << "Wind direction: " << windDirections[0] << " " << " "; break; case 'E': return 0; //Stops execution. default: cout << "Invalid choice. Please follow the menu." << " "; } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
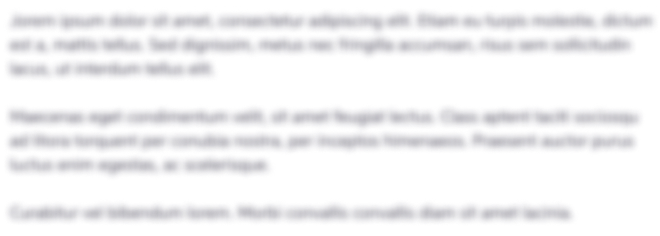
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started