Question
project3code.cpp #include #include #include #include #include #include #include using namespace std; class movie{ public: int id; char title[250]; int year; char rating[6]; int totalCopies; int
project3code.cpp
#include
#include
#include
#include
#include
#include
#include
using namespace std;
class movie{
public:
int id;
char title[250];
int year;
char rating[6];
int totalCopies;
int rentedCopies;
};
class Catalog{
private:
movie movies[200];
int count = 0;
public:
int loadData(ifstream &infile, movie movies[]);
void printAll();
void printRated();
void printTitled();
void addMovie();
void returnMovie();
void rentMovie();
void saveToFile(char *filename);
void printMovie(movie &m);
int find(movie movies[], int count, int id);
};
int main(int argc, char *argv[])
{
Catalog obj; //declaring catalog object
//open file for catalog.txt
char filename[100] = "catalog.txt";
ifstream infile(filename);
movie movies[200];
int count = 0;
if(infile.is_open())
{
obj.loadData(infile, movies);
}
int choice = 0;
while(choice != 7)
{
cout
cout
cout
cout
cout
cout
cout
cout
cout
cin >> choice; //switch statements for different user choices
switch(choice)
{
case 1:
obj.printAll();
break;
case 2:
obj.printTitled();
break;
case 3:
obj.printRated();
break;
case 4:
obj.addMovie();
break;
case 5:
obj.rentMovie();
break;
case 6:
obj.returnMovie();
break;
case 7:
obj.saveToFile(filename);
break;
default:
cout
}
}
return 0;
}
int Catalog::loadData(ifstream &infile, movie movies[])
{
int count = 0;
while(infile >> movies[count].id)
{
infile.ignore();
infile.getline(movies[count].title, 250);
infile >> movies[count].year;
infile >> movies[count].rating;
infile >> movies[count].totalCopies;
infile >> movies[count].rentedCopies;
count++;
}
infile.close();
return count;
}
void Catalog:: printMovie(movie &m)
{
cout
cout
cout
cout
cout
cout
cout
}
void Catalog::printAll()
{
cout
for(int i = 0 ;i
{
printMovie(movies[i]);
}
cout
}
void Catalog::printRated()
{
int r = -1;
char rating[5] = "";
cout
cout
cout
cout
cout
cout
while(true)
{
cin >> r;
if(r 5)
cout
else
break;
}
if(r == 0)
strcpy(rating, "NONE");
else if(r == 1)
strcpy(rating, "G");
else if(r == 2)
strcpy(rating, "PG");
else if(r == 3)
strcpy(rating, "PG13");
else if(r == 4)
strcpy(rating, "R");
else if(r == 5)
strcpy(rating, "N17");
for(int i = 0 ;i
{
if(strcmp(movies[i].rating, rating) == 0)
printMovie(movies[i]);
}
cout
}
void Catalog::printTitled()
{
char title[250];
cout
cin.ignore(); //flush newline
cin.getline(title, 250);
cout
for(int i = 0 ;i
{
if(strcmp(movies[i].title, title) == 0)
{
printMovie(movies[i]);
break;
}
}
cout
}
int Catalog::find(movie movies[], int count, int id)
{
for(int i = 0; i
{
if(movies[i].id == id)
return i;
}
return -1;
}
void Catalog::addMovie() //pass by ref
{
int id;
cout
cin >> id;
while(find(movies, count, id) != -1)
{
cout
cin >> id;
}
movies[count].id = id;
cin.ignore();
cout
cin.getline(movies[count].title, 250);
cout
cin >> movies[count].year;
cout
int r = -1;
cout
cout
cout
cout
cout
cout
while(true)
{
cin >> r;
if(r 5)
cout
else
break;
}
if(r == 0)
strcpy(movies[count].rating, "NONE");
else if(r == 1)
strcpy(movies[count].rating, "G");
else if(r == 2)
strcpy(movies[count].rating, "PG");
else if(r == 3)
strcpy(movies[count].rating, "PG13");
else if(r == 4)
strcpy(movies[count].rating, "R");
else if(r == 5)
strcpy(movies[count].rating, "N17");
cout
while(true)
{
cin >> movies[count].totalCopies;
if(movies[count].totalCopies
cout
else
break;
}
movies[count].rentedCopies = 0;
count++;
}
void Catalog::returnMovie()
{
char answer;
cout
cin >> answer;
if(answer == 'y' || answer == 'Y')
printAll();
int id;
cout
while(true)
{
cin >> id;
if(id == -1)
break;
int index = find(movies, count, id);
if(index == -1)
cout
else
{
if(movies[index].rentedCopies == 0)
cout
else
{
movies[index].rentedCopies--;
cout
}
break;
}
}
}
void Catalog::rentMovie()
{
char answer;
cout
cin >> answer;
if(answer == 'y' || answer == 'Y')
printAll();
int id;
cout
while(true)
{
cin >> id;
if(id == -1)
break;
int index = find(movies, count, id);
if(index == -1)
cout
else
{
if(movies[index].rentedCopies == movies[index].totalCopies)
cout
else
{
movies[index].rentedCopies++;
cout
}
break;
}
}
}
void Catalog::saveToFile(char *filename)
{
ofstream outfile(filename);
if(!outfile.is_open())
cout
else
{
for(int i = 0; i
{
outfile
outfile
outfile
outfile
outfile
outfile
}
outfile.close();
}
}
The purpose of this program is to learn how to use a linear link list to expand or contract the size of list when needed Remember in Project 3 the movie rental app, the number of movies after being read in from the file was fixed at some value which is not very flexible. We still don't want to create more memory that we need but we want the number of movies to increase or decrease as we add movies while running our program. Also, we would like to delete movies as well and print out the titles in sorted order instead of the order they are read in from the file What To Code Take your project 3 code (not the project 4 code)and re-write the Catalog class to use a sorted linear linked list. Add functionality to the program to allow the user to add or delete movies from the catalog. Again, it should read in a list of movies and write out a list of movies and do all the other functionality from project 3 Keep in mind that a linked list doesn't have an index. So to make it more convenient, the find function should pass back a pointer to the object instead of an index. The list should stay sorted for add and delete. However, if the user decides to change the title of the movie then this will cause the list to become unsorted. One way to re-sort the list is to first delete the modified movie and then re-add it to the list. Requirement There should be no arrays (except C-strings) being created in your code anywhere All data is stored in a sorted linear link list. When reading in your file, you should read in one Movie object and call an addToList function to add it to the list; this way you're not duplicating any code as you will need to add to the list when the user adds a new movie. So have both the reading in of a movie and the user adding a new movie call the same addToList function The best way to assure that the list is in 'sorted' order is to always place a new movie in its 'right place' each time you add a movie to the list. Deletion shouldn't change the order of your list so only adding will be affected here. Also, it's okay to write the list out in sorted order even if it was originally unsorted You need to use C-strings, no 'string' type in your code. Also, all functions should less than 30 lines long and no global variablesStep by Step Solution
There are 3 Steps involved in it
Step: 1
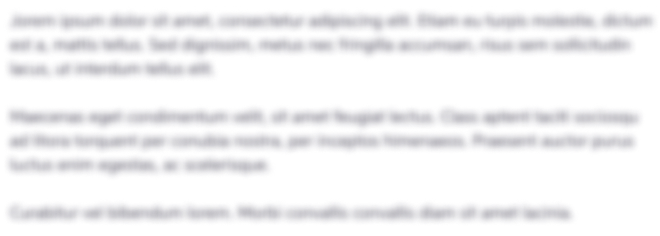
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started