Question
public class Banana extends Fruit { private boolean ripe; public Banana(String colour, boolean ripe) { super(colour); this.ripe = ripe; } public boolean getRipe() { return
public class Banana extends Fruit { private boolean ripe; public Banana(String colour, boolean ripe) { super(colour); this.ripe = ripe; } public boolean getRipe() { return this.ripe; } public void setRipe(boolean r) { this.ripe = r; } public String toString () { return "Banana: colour="+this.colour+" ripe="+this.ripe; } }
public class Apple extends Fruit{ private int age; public Apple (String colour, int age) { super(colour); this.age = age; } public int getAge() { return this.age; } public void setAge(int a) { this.age = a; } public String toString () { return "Apple: colour="+this.colour+" age="+age; } }
public class Fruit { protected String colour; public Fruit (String colour) { this.colour = colour; } public String getColour () { return this.colour; } public void setColour(String c) { this.colour = c; } public String toString () { return "This is the toString for a fruit object! You need to override this method in any subclasses."; } }
import java.util.*;
// Pay attention to the comments of this file // We will tell you where you need to write your code
public class PoD { public static void main(String [] args) { Scanner input = new Scanner (System.in); // Initialize the fruitBasket ArrayList here! // Remember, it is of type Fruit! while (input.hasNext()) { String fruitType = input.next(); String fruitColour = input.next(); if (fruitType.equals("apple")) { int appleAge = input.nextInt(); // Add an Apple to the fruit basket array list here! // Remember, the input for the Apple constructor is (String colour, int age)! } else if (fruitType.equals("banana")) { boolean bananaRipeness = input.nextBoolean(); // Add an Apple to the fruit basket array list here! // Remember, the input for the Apple constructor is (String colour, int age)! } } // Here is the for loop that will print the details of all Fruit in the basket for (Fruit f : fruitBasket) { System.out.println(f); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
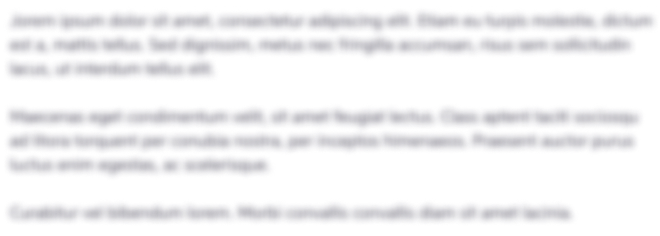
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started