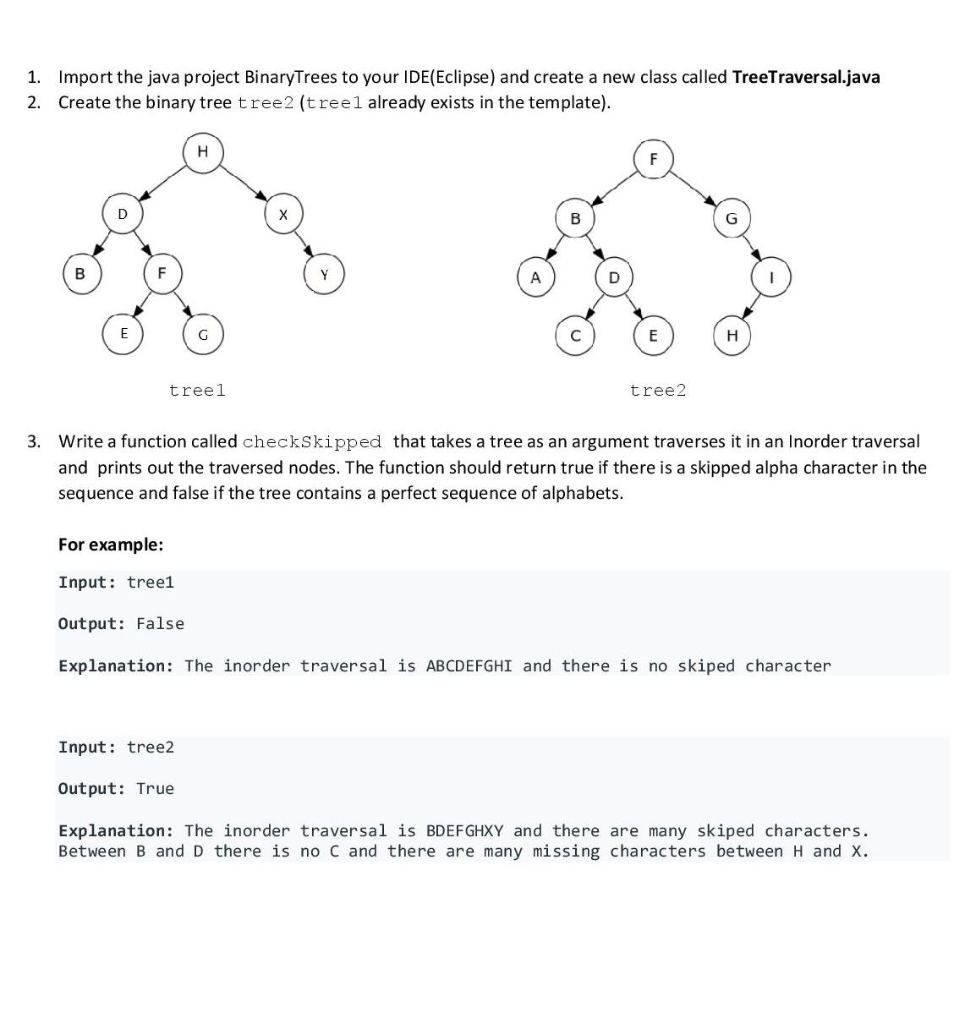
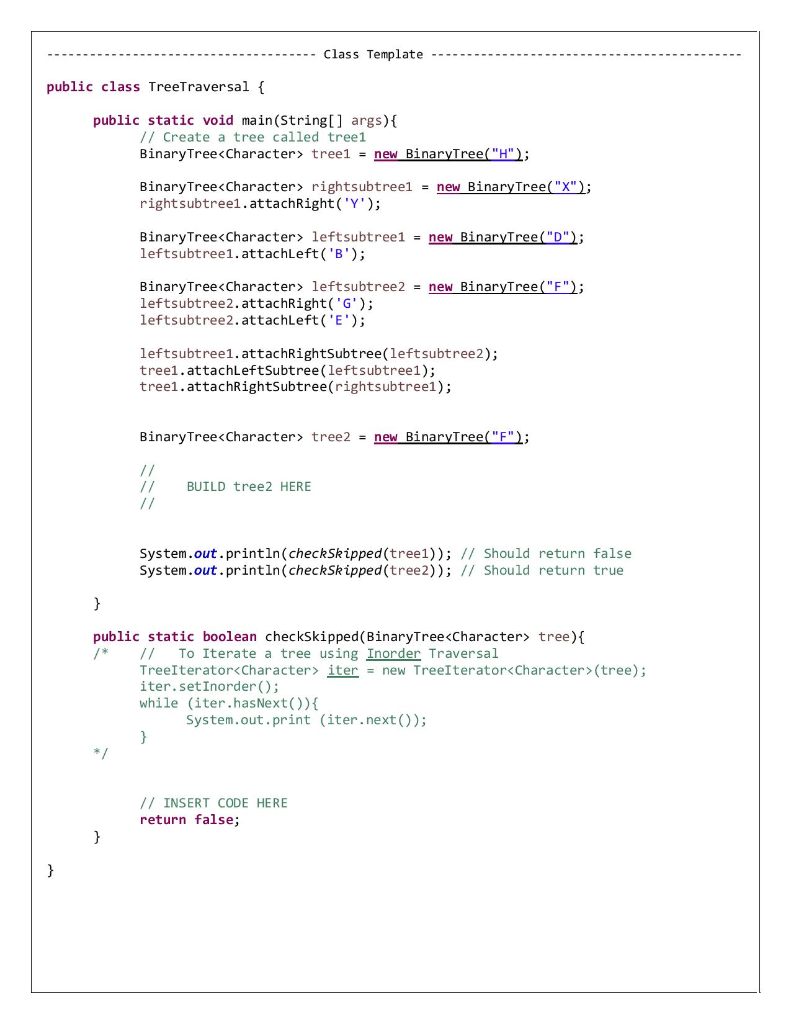
public class BinaryTree extends BinaryTreeBasis { public BinaryTree() { } // end default constructor public BinaryTree(T rootItem) { super(rootItem); } // end constructor public BinaryTree(T rootItem, BinaryTree leftTree, BinaryTree rightTree) { root = new TreeNode(rootItem, null, null); attachLeftSubtree(leftTree); attachRightSubtree(rightTree); } // end constructor public void setRootItem(T newItem) { if (root != null) { root.setItem(newItem); } else { root = new TreeNode(newItem, null, null); } // end if } // end setRootItem public void attachLeft(T newItem) { if (!isEmpty() && root.getLeft() == null) { // assertion: nonempty tree; no left child root.setLeft(new TreeNode(newItem, null, null)); } // end if } // end attachLeft public void attachRight(T newItem) { if (!isEmpty() && root.getRight() == null) { // assertion: nonempty tree; no right child root.setRight(new TreeNode(newItem, null, null)); } // end if } // end attachRight public void attachLeftSubtree(BinaryTree leftTree) throws TreeException { if (isEmpty()) { throw new TreeException("TreeException: Empty tree"); } else if (root.getLeft() != null) { // a left subtree already exists; it should have been // deleted first throw new TreeException("TreeException: " + "Cannot overwrite left subtree"); } else { // assertion: nonempty tree; no left child root.setLeft(leftTree.root); // don't want to leave multiple entry points into // our tree leftTree.makeEmpty(); } // end if } // end attachLeftSubtree public void attachRightSubtree(BinaryTree rightTree) throws TreeException { if (isEmpty()) { throw new TreeException("TreeException: Empty tree"); } else if (root.getRight() != null) { // a right subtree already exists; it should have been // deleted first throw new TreeException("TreeException: " + "Cannot overwrite right subtree"); } else { // assertion: nonempty tree; no right child root.setRight(rightTree.root); // don't want to leave multiple entry points into // our tree rightTree.makeEmpty(); } // end if } // end attachRightSubtree protected BinaryTree(TreeNode rootNode) { root = rootNode; } // end protected constructor public BinaryTree detachLeftSubtree() throws TreeException { if (isEmpty()) { throw new TreeException("TreeException: Empty tree"); } else { // create a new binary tree that has root's left // node as its root BinaryTree leftTree; leftTree = new BinaryTree(root.getLeft()); root.setLeft(null); return leftTree; } // end if } // end detachLeftSubtree public BinaryTree detachRightSubtree() throws TreeException { if (isEmpty()) { throw new TreeException("TreeException: Empty tree"); } else { BinaryTree rightTree; rightTree = new BinaryTree(root.getRight()); root.setRight(null); return rightTree; } // end if } // end detachRightSubtree } // end BinaryTree
1. Import the java project BinaryTrees to your IDE(Eclipse) and create a new class called TreeTraversal.java 2. Create the binary tree tree2 (treel already exists in the template) treel tree2 Write a function called checkSkipped that takes a tree as an argument traverses it in an Inorder traversal and prints out the traversed nodes. The function should return true if there is a skipped alpha character in the sequence and false if the tree contains a perfect sequence of alphabets. 3. For example: Input: tree1 Output: False Explanation: The inorder traversal is ABCDEFGHI and there is no skiped character Input: tree2 Output: True Explanation: The inorder traversal is BDEFGHXY and there are many skiped characters. Between B and D there is no C and there are many missing characters between H and x. public class TreeTraversal [ public static void main(String[] args)( // Create a tree called tree1 BinaryTreeCharacter tree! = new BinaryTree("H"); BinaryTreeCharacter rightsubtreel rightsubtree1.attachRight('Y"); new Bina ryTree("X". BinaryTree
tree){ /* / To Iterate a tree using Inorder Traversal Treelterator