Question
public class Car { private int speed; private int mileage; private String color; private String brand; public Car(int s, int m, String c, String b)
public class Car {
private int speed;
private int mileage;
private String color;
private String brand;
public Car(int s, int m, String c, String b)
{
speed = 0; //just a placeholder
mileage = 0; //just a placeholder
color = "No Color"; //just a placeholder
brand = "No Brand"; //just a placeholder
}
public int getSpeed()
{
return speed;
}
public int getMileage()
{
return mileage;
}
public String getColor()
{
return color;
}
public String getBrand()
{
return brand;
}
public boolean setSpeed(int s)
{
if(s == 0) {
System.exit(0);
}
else
this.speed = s;
return true;
}
public boolean setMileage(int m)
{
if(m == 0) {
System.exit(0);
}
else
this.mileage = m;
return true;
}
public boolean setColor(String c)
{
if(c == null) {
System.exit(0);
}
else
this.color = c;
return true;
}
public boolean setBrand(String b)
{
if(b == null) {
System.exit(0);
}
else
this.brand = b;
return true;
}
}
MAINCODE
public class CarDemo {
public static void main(String[] args) {
Car c = new Car(12,4200,"Red","Ford");
System.out.println("Car speed: "+c.getSpeed()+" km/h");
System.out.println("Car mileage: "+c.getMileage()+" miles");
System.out.println("Car color: "+c.getColor());
System.out.println("Car brand: "+c.getBrand());
}
}
The ouput produce
Car speed : 0 km/h
Car mileage: 0 miles
Car color: null
Car brand: null
How do I change my code if the output that I want
Car speed : 12 km/h
Car mileage: 4200 miles
Car color: Red
Car brand: Ford
Step by Step Solution
There are 3 Steps involved in it
Step: 1
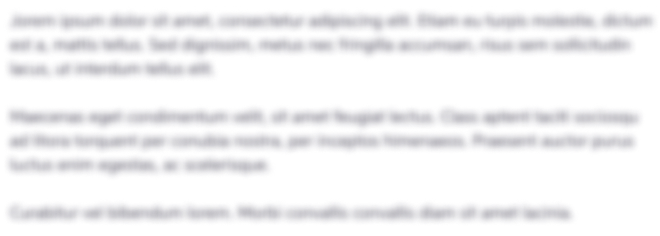
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started