Question
public class Container { private String country; // country of origin private int id; // identification number private int length; // in ft private double
public class Container {
private String country; // country of origin
private int id; // identification number
private int length; // in ft
private double weight; // in tons
public Container(int id, int length, double weight, String country ) {
this.id = id;
this.length = length;
this.weight = weight;
this.country = country;
}
public int getId() {
return id;
}
public int getLength() {
return length;
}
public double getWeight() {
return weight;
}
public String getCountry() {
return country;
}
@Override
public String toString() {
return String.format("%4d: %2dft %ft %s", id, length, weight, country);
}
}
--------------------------
public class Midterm1 {
public static void main(String[] args) {
Container[] containers = {
new Container(1234, 20, 1.9, "China"),
new Container(1235, 40, 3.97, "USA"),
new Container(1236, 40, 4.22, "China"),
new Container(1237, 20, 2.16, "Ghana"),
new Container(1238, 20, 2.1, "USA"),
new Container(1239, 40, 4.08, "Italy"),
new Container(1240, 40, 3.81, "China"),
new Container(1241, 40, 4.2, "USA"),
new Container(1242, 20, 1.82, "Italy")
};
StdOut.println("Containers: ");
StdOut.println("=========== ");
for(Container c : containers) {
StdOut.println(c);
}
System.out.println();
StdOut.println("= = = = Part 1 = = = = ");
StdOut.println("Containers by natural order:");
StdOut.println("============================");
StdOut.println("Containers in reverse order:");
StdOut.println("============================");
StdOut.println("= = = = Part 2 = = = = ");
StdOut.println("Foreign Containers:");
StdOut.println("===================");
}
}
*with doc comment
*JAVA Programing language
Getting Started: // 3 points Output o Containers: eate a new Java project called 2420_Midtermi Create a package called mol Add algs 4. iar to the BuildPath Feate 2 classes: Container and Midterml Midterml includes the main method. ownload the starter code from Midterml CODE opy paste the starter code into your Java project I'll get a compile error because StdOut cannot be resolved Fix the problem and run the code. 1234: 20ft 1.900000t China 1235: 40ft 3.970000t USA 1236: 40ft 4.220000t China 1237: 20ft 2.160000t Ghana 1238: 20ft 2.100000t USA 1239: 40ft 4.080000t Italy 1240: 40ft 3.810000t China 1241: 40ft 4.200000t USA 1242: 20ft 1.820000t Italy Style / Best Practices / Doc Comments: //5 points Use proper indentation, descriptive names, follow Java naming Lunventions, use single empty lines to group related code segments and related output, avoiding code duplication, etc. huu doc comments to all classes, constructors, and methods - including private methods. Output from Part1 (in addition to Output 0): = = = = Part 1 = = = = Create Part1: // 16 points Restrictions: Part 1 can only use classes from java.lang and algs 4, and functionality provided in the classes java.util.Arrays, and java.util.Collections. Containers by natural order: = = = = = = = = == = = = = 1242: 20ft 1.8t Italy 1234: 20ft 1.9t China 1238: 20ft 2.1t USA 1237: 20ft 2.2t Ghana 1240: 40ft 3.8t China 1235: 40ft 4.0t USA 1239: 40ft 4.1t Italy 1241: 40ft 4.2t USA 1236: 40ft 4.2t China Make changes in class Container that reduce the displayed digits after the decimal point to one (1). (4 out of 18 points) The value returned by the getter should not be affected. Make the necessary changes to the code so that you can call the method sort from class Arrays to sort the array containers by its natural order. We define the 'natual order' like this: A containerA is smaller than containerB if the length is shorter. If both containers have the same length, containerA is smaller than containerB if the weight is smaller. (see output) Print the sorted array. (8 out of 18 points) Use a comparator to reverse the order of the elements in the array. Print the array again. (4 out of 18 points) Containers in reverse order: EEEEEEEEEEE================= 1236: 40ft 4.2t China 1241: 40ft 4.2t USA 1239: 40ft 4.1t Italy 1235: 40ft 4.0t USA 1240: 40ft 3.8t China 1237: 20ft 2.2t Ghana 1238: 20ft 2.1t USA 1234: 20ft 1.9t China 1242: 20ft 1.8t Italy Important: The code still needs to work if I initialize the array with different instances of class Container. Getting Started: // 3 points Output o Containers: eate a new Java project called 2420_Midtermi Create a package called mol Add algs 4. iar to the BuildPath Feate 2 classes: Container and Midterml Midterml includes the main method. ownload the starter code from Midterml CODE opy paste the starter code into your Java project I'll get a compile error because StdOut cannot be resolved Fix the problem and run the code. 1234: 20ft 1.900000t China 1235: 40ft 3.970000t USA 1236: 40ft 4.220000t China 1237: 20ft 2.160000t Ghana 1238: 20ft 2.100000t USA 1239: 40ft 4.080000t Italy 1240: 40ft 3.810000t China 1241: 40ft 4.200000t USA 1242: 20ft 1.820000t Italy Style / Best Practices / Doc Comments: //5 points Use proper indentation, descriptive names, follow Java naming Lunventions, use single empty lines to group related code segments and related output, avoiding code duplication, etc. huu doc comments to all classes, constructors, and methods - including private methods. Output from Part1 (in addition to Output 0): = = = = Part 1 = = = = Create Part1: // 16 points Restrictions: Part 1 can only use classes from java.lang and algs 4, and functionality provided in the classes java.util.Arrays, and java.util.Collections. Containers by natural order: = = = = = = = = == = = = = 1242: 20ft 1.8t Italy 1234: 20ft 1.9t China 1238: 20ft 2.1t USA 1237: 20ft 2.2t Ghana 1240: 40ft 3.8t China 1235: 40ft 4.0t USA 1239: 40ft 4.1t Italy 1241: 40ft 4.2t USA 1236: 40ft 4.2t China Make changes in class Container that reduce the displayed digits after the decimal point to one (1). (4 out of 18 points) The value returned by the getter should not be affected. Make the necessary changes to the code so that you can call the method sort from class Arrays to sort the array containers by its natural order. We define the 'natual order' like this: A containerA is smaller than containerB if the length is shorter. If both containers have the same length, containerA is smaller than containerB if the weight is smaller. (see output) Print the sorted array. (8 out of 18 points) Use a comparator to reverse the order of the elements in the array. Print the array again. (4 out of 18 points) Containers in reverse order: EEEEEEEEEEE================= 1236: 40ft 4.2t China 1241: 40ft 4.2t USA 1239: 40ft 4.1t Italy 1235: 40ft 4.0t USA 1240: 40ft 3.8t China 1237: 20ft 2.2t Ghana 1238: 20ft 2.1t USA 1234: 20ft 1.9t China 1242: 20ft 1.8t Italy Important: The code still needs to work if I initialize the array with different instances of class ContainerStep by Step Solution
There are 3 Steps involved in it
Step: 1
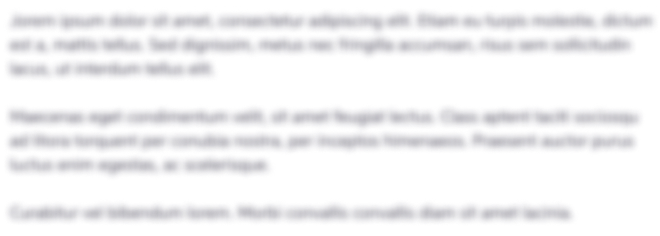
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started