Question
public class MorseCodeTree extends java.lang.Object implements LinkedConverterTreeInterface This is a MorseCodeTree which is specifically used for the conversion of morse code to english It relies
public class MorseCodeTree
extends java.lang.Object
implements LinkedConverterTreeInterface
This is a MorseCodeTree which is specifically used for the conversion of morse code to english It relies on a root (reference to root of the tree) The root is set to null when the tree is empty. The class uses an external generic TreeNode class which consists of a reference to the data and a reference to the left and right child. The TreeNode is parameterized as a String, TreeNode This class uses a private member root (reference to a TreeNode) The constructor will call the buildTree method
Constructor Summary | |
---|---|
MorseCodeTree() Constructor - calls the buildTree method |
Method Summary | |
---|---|
void | addNode(TreeNode |
void | buildTree() This method builds the MorseCodeTree by inserting the nodes of the tree level by level based on the code. |
MorseCodeTree | delete(java.lang.String data) This operation is not supported in the MorseCodeTree |
java.lang.String | fetch(java.lang.String code) Fetch the data in the tree based on the code This method will call the recursive method fetchNode |
java.lang.String | fetchNode(TreeNode |
TreeNode | getRoot() Returns a reference to the root |
MorseCodeTree | insert(java.lang.String code, java.lang.String letter) Adds element to the correct position in the tree based on the code This method will call the recursive method addNode |
void | LNRoutputTraversal(TreeNode |
void | setRoot(TreeNode |
java.util.ArrayList | toArrayList() Returns an ArrayList of the items in the linked Tree in LNR (Inorder) Traversal order Used for testing to make sure tree is built correctly |
MorseCodeTree | update() This operation is not supported in the MorseCodeTree |
Methods inherited from class java.lang.Object |
---|
equals, getClass, hashCode, notify, notifyAll, toString, wait, wait, wait |
Constructor Detail |
---|
MorseCodeTree
public MorseCodeTree()
Constructor - calls the buildTree method
Method Detail |
---|
getRoot
public TreeNodegetRoot()
Returns a reference to the root
Specified by:
getRoot in interface LinkedConverterTreeInterface
Returns:
reference to root
setRoot
public void setRoot(TreeNodenewNode)
sets the root of the MorseCodeTree
Specified by:
setRoot in interface LinkedConverterTreeInterface
Parameters:
newNode - a copy of newNode will be the new root
insert
public MorseCodeTree insert(java.lang.String code, java.lang.String letter)
Adds element to the correct position in the tree based on the code This method will call the recursive method addNode
Specified by:
insert in interface LinkedConverterTreeInterface
Parameters:
code - the code for the new node to be added, example ".-."
Returns:
letter the letter for the new node to be added, example "r"
addNode
public void addNode(TreeNoderoot, java.lang.String code, java.lang.String letter)
This is a recursive method that adds element to the correct position in the tree based on the code. A '.' (dot) means traverse to the left. A "-" (dash) means traverse to the right. The code ".-" would be stored as the right child of the left child of the root Algorithm for the recursive method: 1. if there is only one character a. if the character is '.' (dot) store to the left of the current root b. if the character is "-" (dash) store to the right of the current root c. return 2. if there is more than one character a. if the first character is "." (dot) new root becomes the left child b. if the first character is "-" (dash) new root becomes the right child c. new code becomes all the remaining charcters in the code (beyond the first character) d. call addNode(new root, new code, letter)
Specified by:
addNode in interface LinkedConverterTreeInterface
Parameters:
root - the root of the tree for this particular recursive instance of addNode
code - the code for this particular recursive instance of addNode
letter - the data of the new TreeNode to be added
fetch
public java.lang.String fetch(java.lang.String code)
Fetch the data in the tree based on the code This method will call the recursive method fetchNode
Specified by:
fetch in interface LinkedConverterTreeInterface
Parameters:
code - the code that describes the traversals to retrieve the string (letter)
Returns:
the string (letter) that corresponds to the code
fetchNode
public java.lang.String fetchNode(TreeNoderoot, java.lang.String code)
This is the recursive method that fetches the data of the TreeNode that corresponds with the code A '.' (dot) means traverse to the left. A "-" (dash) means traverse to the right. The code ".-" would fetch the data of the TreeNode stored as the right child of the left child of the root
Specified by:
fetchNode in interface LinkedConverterTreeInterface
Parameters:
root - the root of the tree for this particular recursive instance of addNode
code - the code for this particular recursive instance of addNode
Returns:
the string (letter) corresponding to the code
delete
public MorseCodeTree delete(java.lang.String data) throws java.lang.UnsupportedOperationException
This operation is not supported in the MorseCodeTree
Specified by:
delete in interface LinkedConverterTreeInterface
Parameters:
data - data of node to be deleted
Returns:
reference to the current tree
Throws:
java.lang.UnsupportedOperationException
update
public MorseCodeTree update() throws java.lang.UnsupportedOperationException
This operation is not supported in the MorseCodeTree
Specified by:
update in interface LinkedConverterTreeInterface
Returns:
reference to the current tree
Throws:
java.lang.UnsupportedOperationException
buildTree
public void buildTree()
This method builds the MorseCodeTree by inserting the nodes of the tree level by level based on the code. The root will have a value of "" (empty string) level one: insert(".", "e"); insert("-", "t"); level two: insert("..", "i"); insert(".-", "a"); insert("-.", "n"); insert("--", "m"); etc. Look at the tree and the table of codes to letters in the assignment description.
Specified by:
buildTree in interface LinkedConverterTreeInterface
toArrayList
public java.util.ArrayListtoArrayList()
Returns an ArrayList of the items in the linked Tree in LNR (Inorder) Traversal order Used for testing to make sure tree is built correctly
Specified by:
toArrayList in interface LinkedConverterTreeInterface
Returns:
an ArrayList of the items in the linked Tree
LNRoutputTraversal
public void LNRoutputTraversal(TreeNoderoot, java.util.ArrayList list)
The recursive method to put the contents of the tree in an ArrayList in LNR (Inorder)
Specified by:
LNRoutputTraversal in interface LinkedConverterTreeInterface
Parameters:
root - the root of the tree for this particular recursive instance
list - the ArrayList that will hold the contents of the tree in LNR order
Step by Step Solution
There are 3 Steps involved in it
Step: 1
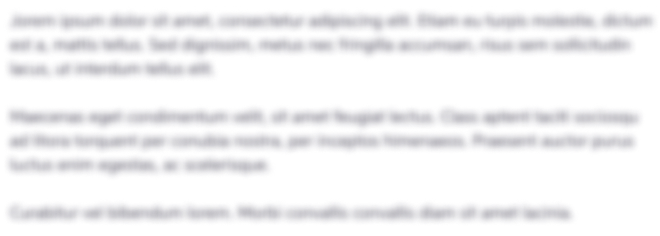
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started