Question
public class Node { private int element; private Node next; public Node(int element) { this.element = element; next = null; } public int getElement() {
public class Node { private int element; private Node next; public Node(int element) { this.element = element; next = null; } public int getElement() { return element; } public void setElement(int element) { this.element = element; } public Node getNext() { return next; } public void setNext(Node next) { this.next = next; } @Override public String toString() { return "" + element; } }
public class LinkedList { private Node head; private int size; public LinkedList() { head = null; size = 0; } public void add(int element) { Node newNode = new Node(element); if (head == null) { head = newNode; size = 1; } else { Node current = head; while (current.getNext() != null) { current = current.getNext(); } current.setNext(newNode); size++; } } public int getSize() { return size; } @Override public String toString() { String s = ""; if (head == null) { return s; } else { Node current = head; while (current.getNext() != null) { s += current.toString() + ", "; current = current.getNext(); } s += current.toString(); // last item has no trailing comma return s; } } }
class Main {
public static void main(String[] args) { /* theChain is 3, 4, 7, 6, 3, 2, 9, 6, 3, 6 */ LinkedList list = new LinkedList(); list.add(3); list.add(4); list.add(7); list.add(6); list.add(3); list.add(2); list.add(9); list.add(6); list.add(3); list.add(6);
System.out.println(list); } }
Language is java
Your task is to implement a public method in the LinkedList class called removeN that takes an integer called element that indicates the element to be removed from the list and an integer parameter n that indicates the number of copies of that element to remove, if possible. The method should return a boolean indicating whether or not the remove operation was successful. Be sure to also update the size of the list appropriately. If there are at least n occurrences of the element in the list, then n nodes with that element are removed starting from the beginning of the list and moving to the right node by node and the method returns true. If there aren't enough occurrences of the element in the list, then the list should not be altered and the method should return false. Write a driver program that throughly tests your method. Examples: list is 3,4,7,6, 3, 2,9,6, 3,6 list.removeN(6, 2) returns true and list is now 3,4,7,3, 2, 9, 3,6 list is 3,4,7,6, 3, 2, 9, 6, 3, 6 list.removeN(6,4) returns false and list is still the same, because there are not four occurrences of the element 6 in the listStep by Step Solution
There are 3 Steps involved in it
Step: 1
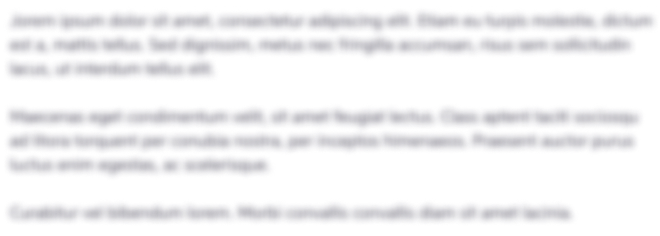
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started