Question
public class WorkingWithBags { // instance variables should be created/initialized by the constructor private BagInterface bagOfStrings; private BagInterface bagOfVowels; // constants should be initialized at
public class WorkingWithBags
{
// instance variables should be created/initialized by the constructor
private BagInterface
private BagInterface
// constants should be initialized at the definition level
final BagInterface
final int MAX_NUMBER_OF_STRINGS_TO_GENERATE = 41;
final int MIN_NUMBER_OF_STRINGS_TO_GENERATE = 35;
final int MAX_STRING_LENGTH = 3;
final int MIN_STRING_LENGTH = 1;
/**
* constructor creates this.bagOfStrings and this.bagOfVowels objects
*/
public WorkingWithBags()
{
// TODO
} // default constructor
/**
* Adds the given entry to this.bagOfStrings if valid
*
* throws InvalidParameterException exception if the length of the string is outside the given bounds
* throws InvalidParameterException exception if the string contains characters other than lowercase letters
*/
public void addToBagOfStrings(String newEntry) throws InvalidParameterException
{
// TODO
}
/**
* Generates randomly string objects and adds them to this.bagOfStrings
*/
public void generateStrings()
{
System.out.println("*** Generating strings ***");
final int SEED = 41;
final int ASCII_LOWERCASE_A = 97;
final int ASCII_LOWERCASE_Z = 122;
Random random = new Random(SEED); // using a seed to help with testing - the output should match exactly the sample run provided
// TODO
// randomly generate an integer between MIN_NUMBER_OF_STRINGS_TO_GENERATE and MAX_NUMBER_OF_STRINGS_TO_GENERATE inclusive
// representing the number of String objects to be generated
// generate the String objects consisting of letters in lower case of randomly generated
// length between MIN_STRING_LENGTH and MAX_STRING_LENGTH inclusive
// call addToBagOfStrings to add the objects to the bag of strings
} // end generateStrings
/**
* Removes the given entry from this.bagOfStrings and if successful creates and adds the replacement to the bag
* The replacement is the given string in reverse
*
* @return returns String in reverse or null
*/
public String replaceGivenEntryWithItsReverse(String entryToReplace)
{
String reverse = null;
// TODO
// no need to call contains - call remove and check its returned value
return reverse;
} // replaceGivenEntryWithItsReverse
/**
* Removes all entries from this.bagOfStrings and if the removed entry is a vowel adds it to this.bagOfVowels
*/
public void findVowels()
{
// TODO
} // findVowels
/**
* Counts and prints the frequency of each vowel in this.bagOfVowels
*/
public void countVowels()
{
// TODO
// display the number of entries in the bag of vowels
// create counters array
// utilize toArray to get an array of vowels from ALL_VOWELS bag
// utilize getFrequencyOf to get frequency of each vowel in the bag of vowels and display the count
} // countVowels
/**
* Displays the number of entries and the content of the given bag
* If the bag is empty displays appropriate message
*/
private void displayContentOfBag(BagInterface
{
// TODO
// If the bag is empty display appropriate message
// Otherwise display the number of entries and the content of the given bag
// Utilize Arrays.toString
} // end displayContentOfBag
public void displayContentOfBagOfStrings()
{
System.out.println("Displaying content of bag of strings:");
displayContentOfBag(this.bagOfStrings);
}
public void displayContentOfBagOfVowels()
{
System.out.println("Displaying content of bag of vowels:");
displayContentOfBag(this.bagOfVowels);
}
public static void main(String args[])
{
long startTime = Calendar.getInstance().getTime().getTime(); // get current time in milliseconds
System.out.println("--> calling constructor");
WorkingWithBags tester = new WorkingWithBags();
tester.displayContentOfBagOfStrings();
tester.displayContentOfBagOfVowels();
System.out.println(" --> calling addToBagOfStrings method to add valid and invalid strings");
String[] stringsToAdd = {"A", "e", "a1", "a c", "Ab", "CSUCI", "csuci", "cal", "if"};
for (int i = 0; i < stringsToAdd.length; i++)
{
try
{
tester.addToBagOfStrings(stringsToAdd[i]);
}
catch (InvalidParameterException ipe)
{
System.out.println(ipe.getMessage());
}
}
tester.displayContentOfBagOfStrings();
System.out.println(" --> calling generateStrings method");
tester.generateStrings();
tester.displayContentOfBagOfStrings();
String entryToReplace = "ca";
System.out.println(" --> calling replaceGivenEntryWithItsReverse method to replace \""
+ entryToReplace + "\"");
String reverse = tester.replaceGivenEntryWithItsReverse(entryToReplace);
if (reverse != null)
System.out.println("\"" + entryToReplace + "\" was successfully replaced with \"" + reverse + "\"");
else
System.out.println("\"" + entryToReplace + "\" was not found");
entryToReplace = "cal";
System.out.println(" --> calling replaceGivenEntryWithItsReverse method to replace \""
+ entryToReplace + "\"");
reverse = tester.replaceGivenEntryWithItsReverse(entryToReplace);
if (reverse != null)
System.out.println("\"" + entryToReplace + "\" was successfully replaced with \"" + reverse + "\"");
else
System.out.println("\"" + entryToReplace + "\" was not found");
entryToReplace = "if";
System.out.println(" --> calling replaceGivenEntryWithItsReverse method to replace \""
+ entryToReplace + "\"");
reverse = tester.replaceGivenEntryWithItsReverse(entryToReplace);
if (reverse != null)
System.out.println("\"" + entryToReplace + "\" was successfully replaced with \"" + reverse + "\"");
else
System.out.println("\"" + entryToReplace + "\" was not found");
entryToReplace = "e";
System.out.println(" --> calling replaceGivenEntryWithItsReverse method to replace \""
+ entryToReplace + "\"");
reverse = tester.replaceGivenEntryWithItsReverse(entryToReplace);
if (reverse != null)
System.out.println("\"" + entryToReplace + "\" was successfully replaced with \"" + reverse + "\"");
else
System.out.println("\"" + entryToReplace + "\" was not found");
System.out.println();
tester.displayContentOfBagOfStrings();
System.out.println(" --> calling findVowels method");
tester.findVowels();
tester.displayContentOfBagOfStrings();
tester.displayContentOfBagOfVowels();
System.out.println(" --> calling countVowels method");
tester.countVowels();
long stopTime = Calendar.getInstance().getTime().getTime();
System.out.println(" The time required was " + (stopTime - startTime) + " milliseconds");
} // end main
Step by Step Solution
There are 3 Steps involved in it
Step: 1
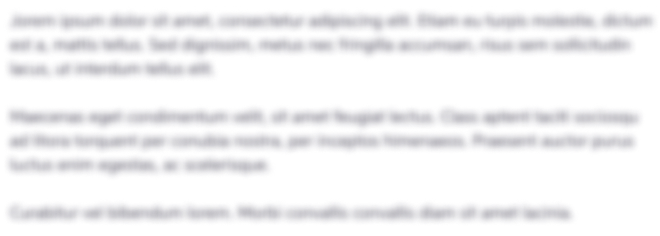
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started