Question
Python 3 Queue Class Implementation: import numpy as np #Custom Queue Implementation #Your code here Define class Queue that implements the ADT (Abstract Data Type)
Python 3 Queue Class Implementation:
import numpy as np #Custom Queue Implementation #Your code here
Define class Queue that implements the ADT (Abstract Data Type) Queue using a circular array.
You must use a np.array for this question:
Somewhere in your constructor you must have a line that looks like self.data=np.array...........
You must observe the FIFO convention.
When implementing a queue, we have to worry about running time for its basic operations: dequeue and enqueue. Both of them must run in Theta(1).
Unfortunately, a regular array does not give you desired complexity.
You will need the following instance attributes:
front - index of the front of the queue
rear - index of the first empty slot (or index of the last element, it is up to you)
num_elems - integer that tracks the number of elements present in the queue
capacity - capacity of the queue, initially 5, initialize your array with elements set to None
data - an array of length capacity (represents your queue)
To implement the behavour of the ADT you need to provide implementations for the following methods:
dequeue - removes and returns the front element
enqueue - adds element, doubling the capacity if is full
is_full - checks whether a queue is full. Returns True if full, False otherwise.
is_empty - checks whether a queue is empty. Returns True if empty, False otherwise.
print_queue - prints queue.
----Format: Assume that elements 4, 5, 6, 7, 8 were added to the queue q. Then q.print_queue() will print: [ | 4 | 5 | 6 | 7 | 8 | ]
----If a queue is empty, print: [ ]
Step by Step Solution
There are 3 Steps involved in it
Step: 1
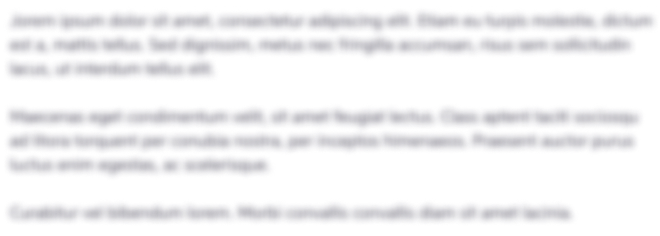
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started