Question
Python An anagram is a permutation of the letters of a word to produce another word. The method print_anagram show below prints all the anagrams
Python
An anagram is a permutation of the letters of a word to produce another word. The method print_anagram show below prints all the anagrams of a given word. To do this, it generates all permutations of the letters in the word and, for each permutation, it checks if it is a valid word in the English language. For example, if you call print_anagrams(spot), the method should print the following words: spot, stop, post, pots, opts, and tops
def print_anagrams(word, prefix=""): if len(word) engish_words: print(prefix + word) else: for i in range(len(word)): cur = word[i: i + 1] before = word[0: i] # letters before cur after = word[i + 1:] # letters after cur if cur not in before: # Check if permutations of cur have not been generated. print_anagrams(before + after, prefix + cur)
The method uses a data structure called engish_words to determine if a given anagram is a valid word in the English language. You can think of engish_words as a container of all the words in the English language. We will implement this data structure using an AVL tree. To populate engish_words, we will use a text file called words.txt that contains English words. Write a function that reads a file and populates an AVL tree with all the English words contained in the file. The code below is the Node and AVL Tree class. How can i adapt the code below to include the word and make it the key.
Text File
class Node:
def __init__(self, key): self.key = key self.parent = None self.left = None self.right = None self.height = 1 def get_balance(self): left_height = -1 if self.left is not None: left_height = self.left.height right_height = -1 if self.right is not None: right_height = self.right.height return left_height - right_height
def update_height(self): left_height = -1 if self.left is not None: left_height = self.left.height right_height = -1 if self.right is not None: right_height = self.right.height
self.height = max(left_height, right_height) + 1
def set_child(self, which_child, child): if which_child != "left" and which_child != "right": return False
if which_child == "left": self.left = child else: self.right = child
if child is not None: child.parent = self
self.update_height() return True
def replace_child(self, current_child, new_child): if self.left is current_child: return self.set_child("left", new_child) elif self.right is current_child: return self.set_child("right", new_child) return False
class AVLTree:
def __init__(self): self.root = None
def rotate_left(self, node): right_left_child = node.right.left if node.parent is not None: node.parent.replace_child(node, node.right) else: # node is root self.root = node.right self.root.parent = None
node.right.set_child('left', node) node.set_child('right', right_left_child) return node.parent
def rotate_right(self, node): left_right_child = node.left.right if node.parent is not None: node.parent.replace_child(node, node.left) else: self.root = node.left self.root.parent = None
node.left.set_child('right', node) node.set_child('left', left_right_child) return node.parent
def rebalance(self, node): node.update_height() if node.get_balance() == -2: if node.right.get_balance() == 1: self.rotate_right(node.right) return self.rotate_left(node) elif node.get_balance() == 2:
if node.left.get_balance() == -1: self.rotate_left(node.left) return self.rotate_right(node) return node def insert(self, node): if self.root is None: self.root = node node.parent = None
else: current_node = self.root while current_node is not None: if node.key
node = node.parent while node is not None: self.rebalance(node) node = node.parent
def search(self, key): current_node = self.root while current_node is not None: if current_node.key == key: return current_node elif current_node.key
def remove_key(self, key): node = self.search(key) if node is None: return False else: return self.remove_node(node)
def main(): if __name__ =='__main__': main()
words.txt File Edit Format View Help apple donut orange cheeta dog cat Triton Abelia sleep sky green Fontes raised hey oh marvel tree fifth words.txt File Edit Format View Help apple donut orange cheeta dog cat Triton Abelia sleep sky green Fontes raised hey oh marvel tree fifthStep by Step Solution
There are 3 Steps involved in it
Step: 1
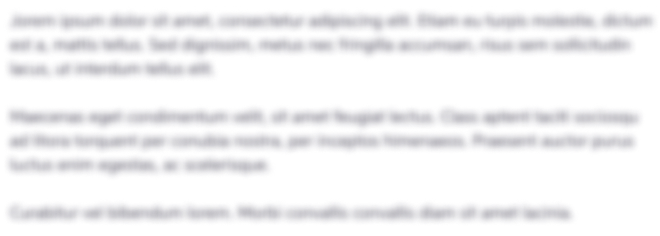
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started