Question
Python Assignment PyFighter P a g e | 1 This program will implement a combat system for an imaginary game called PyFighter (Python Fighter) Read
Python Assignment PyFighter P a g e | 1 This program will implement a combat system for an imaginary game called PyFighter (Python Fighter) Read the document carefully, including the sample of a complete battle shown on page 5. Pages 2 and 3 describe the rules for the combat. Page 4 shows a sample combat round. Page 5 shows a complete battle. Page 6 shows additional considerations to prevent endless battles. Input: Allow the user to enter the stats (attributes) for fighter 1 and fighter 2. Output: Show the results of the battle, round by one. An example of this is shown on page 5. Your output does not have to match this but should be informative, at least showing the attacks, real damage, and who lost that round. The output does not have to include the "CR History" shown at the bottom of the sample output, but you earn more credit if you do. How the assignment is graded: 80%: Create a correct implementation of the rules shown on page 2 and 3. The output from the program must be clear and easily understood by me. 10%: Good code comments. 10%: Add one or more fixes to prevent a never-ending battle, as shown on page 6. Add comments to document your fix at the top of the program. For the rest of this document, assume that all dice mentioned are six sided dice (cubes) that roll random numbers between 1 and 6. Python Assignment PyFighter P a g e | 2 Description: The program will pit two fighters against each other. The fighters will fight round after round until one is defeated. Each fighter has 4 attributes (called stats for the rest of this document): name combat_rating (called CR for the rest of this document) armors attack_dice These stats are used to determine the outcome of a series of rounds, according to the following rules. The combat is over when one fighter or the other's CR drops to zero or below. Explanation of stats: name A string containing the name of the character combat_rating The health of the fighter. when CR drops to 0 or below, the fighter is defeated. CR is also used to determine an attack bonus equal to 1/2 the CR. attack_dice How many dice the fighter gets to roll in an attack. armor How many points of damage is absorbed / ignored per round Example fighter: name: Conan CR: 10 (the fighter's health. Also used to generate an attack bonus) armor: 2 (how many points of damage can be absorbed per round) attack_dice: 5 (how many dice the fighter rolls per attack) Program flow: The program should o Ask the user for the stats of two fighters o Loop, doing combat rounds, until one fighter or the other is defeated o Announce the name of the winning fighter Python Assignment PyFighter P a g e | 3 Combat round: 1. Determine attacks 2. Compare attacks to determine the loser 3. Calculate damage 4. Calculate real damage for the fighter that loses 5. Apply the real damage to the fighter's CR. Details: 1. Each fighter generates an attack value as described below: The fighter's combat roll is the sum of their dice (the number of dice equals their "dice" stat) The fighter's CR bonus is 1/2 their CR. The attack = combat roll + CR bonus 2. If their attacks are exactly equal, nothing happens and the round is over. Otherwise 3. Damage is calculated as the difference between the attacks. 4. "Real damage" = damage minus the loser's armor (but cannot be less than 0) 5. If the real damage is greater than 0, the loser's CR is lowered by that amount Stated again: these are the same rules, worded a bit differently to help understanding Steps 1 An attack for a round consists of the fighter's attack dice rolled and added together, plus 1/2 of the fighter's combat rating. Both fighters attacks are generated. Let's say we call them attack1 and attack2. Step 2: If attack1 equals attack2, that's it, and the round is over. Step 3 To determine damage, you take the diffence between the attacks. So damage = the absolute value of attack1 minus attack2. Steps 4 and 5 The real damage = the damage minus the losing fighter's armor. If real damage > 0, then the fighter who made the lower attack has that damage subtraced from their CR. Combat continues, round by round, until someone's CR reaches 0 or below. When done the winner will be announced. Python Assignment PyFighter P a g e | 4 Combat round example: Assuming our example fighter, Conan: name: Conan CR: 10 armor: 2 attack_dice: 5 He has an opponent with six attack dice, and the same CR and armor. Steps 1 Determine attacks Conan has 5 attack dice. Five random numbers are added together. Say the random numbers are 2+5+3+6+4 for a total of 22. So his base attack roll = 22 His bonus is 1/2 CR. 1/2 * 10 is 5. So his bonus is 5. Conan's total attack: 22 + 5 = 27 So Conan's total attack is 27 His opponent's total attack is also calculated. Six random numbers 5+4+5+3+6+4 + CR bonus of 5 = 32. Step 2 Compare attacks The attacks are not equal, so the round is not over yet. Conan loses this round. Step 3: Calculate raw damage Damage = 32 - 27 = 5 (Use absolute value to make sure damage is a positive value.) Step 4: Calculate real damage Real damage = damage minus armor. Damage was 5. Conan's armor is 2. 5 - 2 = 3 Step 6: The real damage is > 0, so Conan will take damage. Conan takes 3 points of real damage, and his CR lowers from 10 to 7. Loop, repeating those steps until one (or both) fighter's CR is 0 or less. An example of a complete battle follows on the next page. Python Assignment PyFighter P a g e | 5 First, the user inputs stats for each fighter. In this example, the stats are identical. Then the program continues Conan has a CR of 10, armor of 1, and rolls 5 dice Trogdar has a CR of 10, armor of 1, and rolls 5 dice ((((((Let the battle begin!)))))) (((((( round 1 )))))) Conan rolls = ( 3 3 6 2 3 ) plus CR bonus of 5 for a total attack of 22 Trogdar rolls = ( 1 1 6 2 6 ) plus CR bonus of 5 for a total attack of 25 Conan total attack = 24 and Trogdar total attack = 22 Damage is 2 Conan's armor's absorbs 1 so Conan is hit for 2 points Conan CR is now 8 and Trogdar CR is now 10 (((((( round 2 )))))) Conan rolls = ( 5 4 4 2 4 ) plus CR bonus of 4 for a total attack of 23 Trogdar rolls = ( 1 5 4 6 2 ) plus CR bonus of 5 for a total attack of 23 Attacks equal, so nobody takes damage Skipping round 3, where Conan again take 2 points of damage. His CR is now 6, Trogdar's still 10. (((((( round 4 )))))) Conan rolls = ( 4 4 2 5 6 ) plus CR bonus of 3 for a total attack of 23 Trogdar rolls = ( 1 4 2 1 2 ) plus CR bonus of 5 for a total attack of 15 Conan total attack = 23 and Trogdar total attack = 15 Damage is 8 Trogdar armor's absorbs 1 so Trogdar is hit for 7 points Conan CR is now 6 and Trogdar CR is now 3 (((((( round 5 )))))) Conan rolls = ( 6 2 3 1 4 ) plus CR bonus of 3 for a total attack of 19 Trogdar rolls = ( 2 1 4 3 3 ) plus CR bonus of 1 for a total attack of 14 Conan total attack = 19 and Trogdar total attack = 14 Damage is 5 Trogdar armor's absorbs 1 so Trogdar is hit for 4 points Conan CR is now 6 and Trogdar CR is now 0 Conan CR history round 1: ******** round 2: ******** round 3: ****** round 4: ****** round 5: ****** Trogdar CR history round 1: ********** round 2: ********** round 3: ********** round 4: *** round 4: Trogdar is defeated (((((( The battle is over after 4 rounds )))))) Conan wins! Python Assignment PyFighter P a g e | 6 Optional considerations to prevent endless battle Once you implement the combat logic, you will notice a problem If the fighters have armor that is too high, the battle will go endlessly with neither fighter taking any real damage. Try it: Each fighter has a CR of 10, 5 dice, but with an armor of 10. The program will loop, round after round. If their armor is better than the highest possible damage that could be generated, it will be an infinite loop. How could this be resolved? Think about how the program could prevent a combat from going endlessly. Here are some optional combat rules I thought about. Feel free to come up with your own! Here's a few I thought about. Maybe just limit the number of rounds to 10 or 20 or the something, and if there is no winner, call it a tie. This is the simple (boring) solution. You won't get credit for this method! Perhaps some particular value is a "critical hit" that automatically subtracts 1 from the other fighter's CR, no matter who wins or loses the round. Perhaps the minimum real damage is 1, no matter what. Or perhaps the losing fighter's armor rating goes down, even if no real damage occurred. (This would lower the armor rating for the fighters as time goes by so that real damage will start occurring.) Maybe the # of attack rolls could be increased as the combat goes on. (They get angrier!) These are just some ideas. The goal would be to somehow reduce the armor or increase the damage as the battle progresses. There are lots of ways this could be done. Implementation notes: Allow the user to enter the states for fighter 1 and fighter 2. Implement at least one optional rule to prevent infinite looping. Document your optional rules in comments at the top of the program. Comment your code well. The battle output does not have to match what is shown on page 5. But make it clear what is happening. Don't just have your program run for a while with no output and then print a winner's name. Python Assignment PyFighter P a g e | 7
Step by Step Solution
There are 3 Steps involved in it
Step: 1
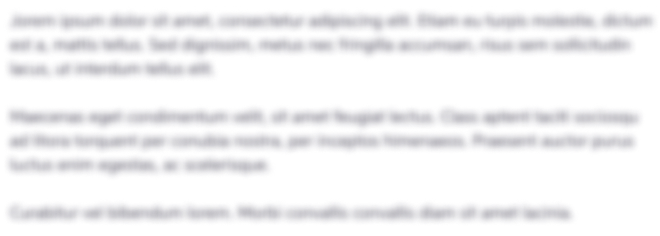
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started